
- •About the Author
- •About the Technical Editor
- •Credits
- •Is This Book for You?
- •Software Versions
- •Conventions This Book Uses
- •What the Icons Mean
- •How This Book Is Organized
- •How to Use This Book
- •What’s on the Companion CD
- •What Is Excel Good For?
- •What’s New in Excel 2010?
- •Moving around a Worksheet
- •Introducing the Ribbon
- •Using Shortcut Menus
- •Customizing Your Quick Access Toolbar
- •Working with Dialog Boxes
- •Using the Task Pane
- •Creating Your First Excel Worksheet
- •Entering Text and Values into Your Worksheets
- •Entering Dates and Times into Your Worksheets
- •Modifying Cell Contents
- •Applying Number Formatting
- •Controlling the Worksheet View
- •Working with Rows and Columns
- •Understanding Cells and Ranges
- •Copying or Moving Ranges
- •Using Names to Work with Ranges
- •Adding Comments to Cells
- •What Is a Table?
- •Creating a Table
- •Changing the Look of a Table
- •Working with Tables
- •Getting to Know the Formatting Tools
- •Changing Text Alignment
- •Using Colors and Shading
- •Adding Borders and Lines
- •Adding a Background Image to a Worksheet
- •Using Named Styles for Easier Formatting
- •Understanding Document Themes
- •Creating a New Workbook
- •Opening an Existing Workbook
- •Saving a Workbook
- •Using AutoRecover
- •Specifying a Password
- •Organizing Your Files
- •Other Workbook Info Options
- •Closing Workbooks
- •Safeguarding Your Work
- •Excel File Compatibility
- •Exploring Excel Templates
- •Understanding Custom Excel Templates
- •Printing with One Click
- •Changing Your Page View
- •Adjusting Common Page Setup Settings
- •Adding a Header or Footer to Your Reports
- •Copying Page Setup Settings across Sheets
- •Preventing Certain Cells from Being Printed
- •Preventing Objects from Being Printed
- •Creating Custom Views of Your Worksheet
- •Understanding Formula Basics
- •Entering Formulas into Your Worksheets
- •Editing Formulas
- •Using Cell References in Formulas
- •Using Formulas in Tables
- •Correcting Common Formula Errors
- •Using Advanced Naming Techniques
- •Tips for Working with Formulas
- •A Few Words about Text
- •Text Functions
- •Advanced Text Formulas
- •Date-Related Worksheet Functions
- •Time-Related Functions
- •Basic Counting Formulas
- •Advanced Counting Formulas
- •Summing Formulas
- •Conditional Sums Using a Single Criterion
- •Conditional Sums Using Multiple Criteria
- •Introducing Lookup Formulas
- •Functions Relevant to Lookups
- •Basic Lookup Formulas
- •Specialized Lookup Formulas
- •The Time Value of Money
- •Loan Calculations
- •Investment Calculations
- •Depreciation Calculations
- •Understanding Array Formulas
- •Understanding the Dimensions of an Array
- •Naming Array Constants
- •Working with Array Formulas
- •Using Multicell Array Formulas
- •Using Single-Cell Array Formulas
- •Working with Multicell Array Formulas
- •What Is a Chart?
- •Understanding How Excel Handles Charts
- •Creating a Chart
- •Working with Charts
- •Understanding Chart Types
- •Learning More
- •Selecting Chart Elements
- •User Interface Choices for Modifying Chart Elements
- •Modifying the Chart Area
- •Modifying the Plot Area
- •Working with Chart Titles
- •Working with a Legend
- •Working with Gridlines
- •Modifying the Axes
- •Working with Data Series
- •Creating Chart Templates
- •Learning Some Chart-Making Tricks
- •About Conditional Formatting
- •Specifying Conditional Formatting
- •Conditional Formats That Use Graphics
- •Creating Formula-Based Rules
- •Working with Conditional Formats
- •Sparkline Types
- •Creating Sparklines
- •Customizing Sparklines
- •Specifying a Date Axis
- •Auto-Updating Sparklines
- •Displaying a Sparkline for a Dynamic Range
- •Using Shapes
- •Using SmartArt
- •Using WordArt
- •Working with Other Graphic Types
- •Using the Equation Editor
- •Customizing the Ribbon
- •About Number Formatting
- •Creating a Custom Number Format
- •Custom Number Format Examples
- •About Data Validation
- •Specifying Validation Criteria
- •Types of Validation Criteria You Can Apply
- •Creating a Drop-Down List
- •Using Formulas for Data Validation Rules
- •Understanding Cell References
- •Data Validation Formula Examples
- •Introducing Worksheet Outlines
- •Creating an Outline
- •Working with Outlines
- •Linking Workbooks
- •Creating External Reference Formulas
- •Working with External Reference Formulas
- •Consolidating Worksheets
- •Understanding the Different Web Formats
- •Opening an HTML File
- •Working with Hyperlinks
- •Using Web Queries
- •Other Internet-Related Features
- •Copying and Pasting
- •Copying from Excel to Word
- •Embedding Objects in a Worksheet
- •Using Excel on a Network
- •Understanding File Reservations
- •Sharing Workbooks
- •Tracking Workbook Changes
- •Types of Protection
- •Protecting a Worksheet
- •Protecting a Workbook
- •VB Project Protection
- •Related Topics
- •Using Excel Auditing Tools
- •Searching and Replacing
- •Spell Checking Your Worksheets
- •Using AutoCorrect
- •Understanding External Database Files
- •Importing Access Tables
- •Retrieving Data with Query: An Example
- •Working with Data Returned by Query
- •Using Query without the Wizard
- •Learning More about Query
- •About Pivot Tables
- •Creating a Pivot Table
- •More Pivot Table Examples
- •Learning More
- •Working with Non-Numeric Data
- •Grouping Pivot Table Items
- •Creating a Frequency Distribution
- •Filtering Pivot Tables with Slicers
- •Referencing Cells within a Pivot Table
- •Creating Pivot Charts
- •Another Pivot Table Example
- •Producing a Report with a Pivot Table
- •A What-If Example
- •Types of What-If Analyses
- •Manual What-If Analysis
- •Creating Data Tables
- •Using Scenario Manager
- •What-If Analysis, in Reverse
- •Single-Cell Goal Seeking
- •Introducing Solver
- •Solver Examples
- •Installing the Analysis ToolPak Add-in
- •Using the Analysis Tools
- •Introducing the Analysis ToolPak Tools
- •Introducing VBA Macros
- •Displaying the Developer Tab
- •About Macro Security
- •Saving Workbooks That Contain Macros
- •Two Types of VBA Macros
- •Creating VBA Macros
- •Learning More
- •Overview of VBA Functions
- •An Introductory Example
- •About Function Procedures
- •Executing Function Procedures
- •Function Procedure Arguments
- •Debugging Custom Functions
- •Inserting Custom Functions
- •Learning More
- •Why Create UserForms?
- •UserForm Alternatives
- •Creating UserForms: An Overview
- •A UserForm Example
- •Another UserForm Example
- •More on Creating UserForms
- •Learning More
- •Why Use Controls on a Worksheet?
- •Using Controls
- •Reviewing the Available ActiveX Controls
- •Understanding Events
- •Entering Event-Handler VBA Code
- •Using Workbook-Level Events
- •Working with Worksheet Events
- •Using Non-Object Events
- •Working with Ranges
- •Working with Workbooks
- •Working with Charts
- •VBA Speed Tips
- •What Is an Add-In?
- •Working with Add-Ins
- •Why Create Add-Ins?
- •Creating Add-Ins
- •An Add-In Example
- •System Requirements
- •Using the CD
- •What’s on the CD
- •Troubleshooting
- •The Excel Help System
- •Microsoft Technical Support
- •Internet Newsgroups
- •Internet Web sites
- •End-User License Agreement

Part VI: Programming Excel with VBA
If the active sheet contains a range named data, the following statement assigns the number of cells in the data range to a variable named CellCount:
CellCount = Range(“data”).Count
You can also determine how many rows or columns are contained in a range. The following expression calculates the number of columns in the currently selected range:
Selection.Columns.Count
And, of course, you can also use the Rows property to determine the number of rows in a range. The following statement counts the number of rows in a range named data and assigns the number to a variable named RowCount:
RowCount = Range(“data”).Rows.Count
Working with Workbooks
The examples in this section demonstrate various ways to use VBA to work with workbooks.
Saving all workbooks
The following procedure loops through all workbooks in the Workbooks collection and saves each file that has been saved previously:
Public Sub SaveAllWorkbooks()
Dim Book As Workbook
For Each Book In Workbooks
If Book.Path <> “” Then Book.Save
Next Book
End Sub
Notice the use of the Path property. If a workbook’s Path property is empty, the file has never been saved (it’s a new workbook). This procedure ignores such workbooks and saves only the workbooks that have a nonempty Path property.
Saving and closing all workbooks
The following procedure loops through the Workbooks collection. The code saves and closes all workbooks.
896
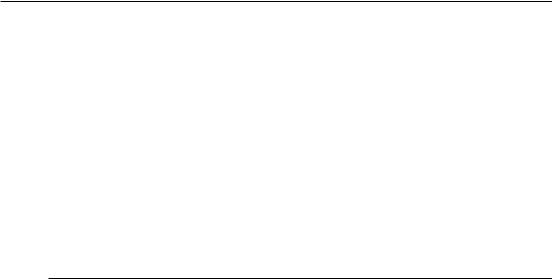
Chapter 44: VBA Examples
Sub CloseAllWorkbooks()
Dim Book As Workbook
For Each Book In Workbooks
If Book.Name <> ThisWorkbook.Name Then
Book.Close savechanges:=True
End If
Next Book
ThisWorkbook.Close savechanges:=True
End Sub
The procedure uses an If statement within the For-Next loop to determine whether the workbook is the workbook that contains the code. This is necessary because closing the workbook that contains the procedure would end the code, and subsequent workbooks would not be affected.
Working with Charts
Manipulating charts with VBA can be confusing, mainly because of the large number of objects involved. To get a feel for working with charts, turn on the macro recorder, create a chart, and perform some routine chart editing. You may be surprised by the amount of code that’s generated.
When you understand how objects function within in a chart, however, you can create some useful macros. This section presents a few macros that deal with charts. When you write macros that manipulate charts, you need to understand some terminology. An embedded chart on a worksheet is a ChartObject object, and the ChartObject contains the actual Chart object. A chart on a chart sheet, on the other hand, does not have a ChartObject container.
It’s often useful to create an object reference to a chart (see “Simplifying object references,” later in this chapter). For example, the following statement creates an object variable (MyChart) for the embedded chart named Chart 1 on the active sheet.
Dim MyChart As Chart
Set MyChart = ActiveSheet.ChartObjects(“Chart 1”)
The following sections contain examples of macros that work with charts.
On the CD
These macros are available on the companion CD-ROM. The file is named chart macros.xlsm.
897

Part VI: Programming Excel with VBA
Modifying the chart type
The following example changes the chart type of every embedded chart on the active sheet. It makes each chart an area chart by adjusting the ChartType property of the Chart object. A built-in constant, xlColumnClustered, represents a standard column chart.
Sub ChartType()
Dim ChtObj As ChartObject
For Each ChtObj In ActiveSheet.ChartObjects
ChtObj.Chart.ChartType = xlColumnClustered
Next ChtObj
End Sub
The preceding example uses a For-Next loop to cycle through all the ChartObject objects on the active sheet. Within the loop, the chart type is assigned a new value, making it an area chart.
The following macro performs the same function but works on all chart sheets in the active workbook:
Sub ChartType2()
Dim Cht As Chart
For Each Cht In ActiveWorkbook.Charts
Cht.ChartType = xlColumnClustered
Next Cht
End Sub
Modifying chart properties
The following example changes the legend font for all charts that are on the active sheet. It uses a For-Next loop to process all ChartObject objects and uses the HasLegend property to ensure that the chart has a legend. The code then adjusts the properties of the Font object contained in the Legend object:
Sub LegendMod()
Dim ChtObj As ChartObject
For Each ChtObj In ActiveSheet.ChartObjects
ChtObj.Chart.HasLegend = True
With ChtObj.Chart.Legend.Font
.Name = “Arial”
.FontStyle = “Bold”
.Size = 8
End With
Next ChtObj
End Sub
898
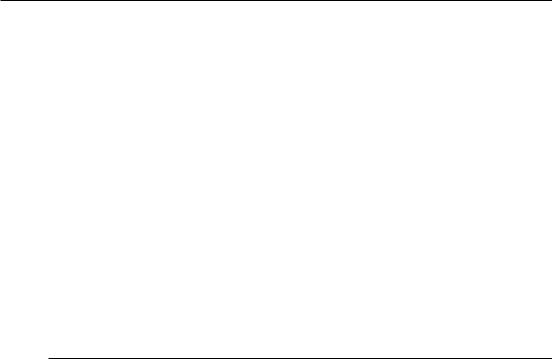
Chapter 44: VBA Examples
Applying chart formatting
This example applies several different formatting types to the specified chart (in this case, Chart 1 on the active sheet):
Sub ChartMods()
With ActiveSheet.ChartObjects(“Chart 1”).Chart
.ChartType = xlArea
.ChartArea.Font.Name = “Arial”
.ChartArea.Font.FontStyle = “Regular”
.ChartArea.Font.Size = 9
.PlotArea.Interior.ColorIndex = 6
.Axes(xlValue).TickLabels.Font.Bold = True
.Axes(xlCategory).TickLabels.Font.Bold = True
End With
End Sub
One way to learn about these properties is to record a macro while you apply various changes to a chart.
VBA Speed Tips
VBA is fast, but it’s often not fast enough. This section presents programming examples that you can use to help speed your macros.
Turning off screen updating
You’ve probably noticed that when you execute a macro, you can watch everything that occurs in the macro. Sometimes, this view is instructive; but, after you get the macro working properly, it can be annoying and slow things considerably.
Fortunately, you can disable the normal screen updating that occurs when you execute a macro. Insert the following statement to turn off screen updating:
Application.ScreenUpdating = False
If, at any point during the macro, you want the user to see the results of the macro, use the following statement to turn screen updating back on:
Application.ScreenUpdating = True
899

Part VI: Programming Excel with VBA
Preventing alert messages
One benefit of using a macro is that you can perform a series of actions automatically. You can start a macro and then get a cup of coffee while Excel does its thing. Some operations cause Excel to display messages that must be attended to, however. For example, if your macro deletes a sheet, you see the message that is shown in the dialog box in Figure 44.3. These types of messages mean that you can’t execute your macro unattended.
FIGURE 44.3
You can instruct Excel not to display these types of alerts while a macro is running.
To avoid these alert messages (and automatically choose the default response) , insert the following VBA statement:
Application.DisplayAlerts = False
To turn alerts back on, use this statement:
Application.DisplayAlerts = True
Simplifying object references
As you may have discovered, references to objects can get very lengthy — especially if your code refers to an object that’s not on the active sheet or in the active workbook. For example, a fully qualified reference to a Range object may look like this:
Workbooks(“MyBook.xlsx”).Worksheets(“Sheet1”).Range(“IntRate”)
If your macro uses this range frequently, you may want to use the Set command to create an object variable. For example, to assign this Range object to an object variable named Rate, use the following statement:
Set Rate= Workbooks(“MyBook.xlsx”).Worksheets(“Sheet1”).Range(“IntRate”)
After this variable is defined, you can use the variable Rate instead of the lengthy reference. For example
Rate.Value = .0725
900

Chapter 44: VBA Examples
Besides simplifying your coding, using object variables also speeds your macros quite a bit. I’ve seen macros execute twice as fast after creating object variables.
Declaring variable types
Usually, you don’t have to worry about the type of data that’s assigned to a variable. Excel handles all these details behind the scenes. For example, if you have a variable named MyVar, you can assign a number of any type to it. You can even assign a text string to it later in the procedure.
If you want your procedures to execute as fast as possible, though, you should tell Excel in advance what type of data is going be assigned to each of your variables. Providing this information in your VBA procedure is known as declaring a variable’s type.
Table 44.1 lists all the data types that are supported by VBA. This table also lists the number of bytes that each type uses and the approximate range of possible values.
TABLE 44.1
|
VBA Data Types |
|
Data Type |
Bytes Used |
Approximate Range of Values |
|
|
|
Byte |
1 |
0 to 255 |
|
|
|
Boolean |
2 |
True or False |
|
|
|
Integer |
2 |
–32,768 to 32,767 |
|
|
|
Long (long integer) |
4 |
–2,147,483,648 to 2,147,483,647 |
|
|
|
Single (single-precision |
4 |
–3.4E38 to –1.4E–45 for negative values; 1.4E–45 to |
floating-point) |
|
4E38 for positive values |
|
|
|
Double (double-precision |
8 |
–1.7E308 to –4.9E–324 for negative values; 4.9E–324 |
floating-point) |
|
to .7E308 for positive values |
|
|
|
Currency (scaled integer) |
8 |
–9.2E14 to 9.2E14 |
|
|
|
Decimal |
14 |
+/–7.9E28 with no decimal point |
|
|
|
Date |
8 |
January 1, 100 to December 31, 9999 |
|
|
|
Object |
4 |
Any object reference |
|
|
|
String (variable-length) |
10 + string length |
0 to approximately 2 billion |
|
|
|
String (fixed-length) |
Length of string |
1 to approximately 65,400 |
|
|
|
Variant (with numbers) |
16 |
Any numeric value up to the range of a Double |
|
|
|
Variant (with characters) |
22 + string length |
Same range as for variable-length String |
|
|
|
User-defined (using Type) |
Number required by |
Range of each element is the same as the range of its |
|
elements |
data type |
|
|
|
901

Part VI: Programming Excel with VBA
If you don’t declare a variable, Excel uses the Variant data type. In general, the best technique is to use the data type that uses the smallest number of bytes yet can still handle all the data assigned to it. An exception is when you’re performing floating-point calculations. In such a case, it is always best to use the Double data type (rather than the Single data type) to maintain maximum precision. Another exception involves the Integer data type. Although the Long data type uses more bytes, it usually results in faster performance.
When VBA works with data, execution speed is a function of the number of bytes that VBA has at its disposal. In other words, the fewer bytes that are used by data, the faster VBA can access and manipulate the data.
To declare a variable, use the Dim statement before you use the variable for the first time. For example, to declare the variable Units as a Long data type, use the following statement:
Dim Units as Long
To declare the variable UserName as a string, use the following statement:
Dim UserName as String
If you declare a variable within a procedure, the declaration is valid only within that procedure. If you declare a variable outside of any procedures (but before the first procedure), the variable is valid in all procedures in the module.
If you use an object variable (as described in “Simplifying object references,” earlier in this chapter), you can declare the variable as the appropriate object data type. The following is an example:
Dim Rate as Range
Set Rate = Workbooks(“MyBook.xlsx”).Worksheets(“Sheet1”).Range(“IntRate”)
To force yourself to declare all the variables that you use, insert the following statement at the top of your module:
Option Explicit
If you use this statement, Excel displays an error message if it encounters a variable that hasn’t been declared. After you get into the habit of correctly declaring all your variables, you will find that it helps eliminate errors and makes spotting errors easier.
902