
- •Contents at a Glance
- •Contents
- •Foreword
- •About the Authors
- •About the Technical Reviewer
- •Acknowledgments
- •Introduction
- •Who This Book Is For
- •An Overview of This Book
- •Example Code and Companion Web Site
- •Contacting the Authors
- •Overview of HTML5
- •The Story So Far—The History of HTML5
- •The Myth of 2022 and Why It Doesn’t Matter
- •Who Is Developing HTML5?
- •A New Vision
- •Compatibility and Paving the Cow Paths
- •Utility and the Priority of Constituencies
- •Interoperability Simplification
- •Universal Access
- •A Plugin–Free Paradigm
- •What’s In and What’s Out?
- •What’s New in HTML5?
- •New DOCTYPE and Character Set
- •New and Deprecated Elements
- •Semantic Markup
- •Simplifying Selection Using the Selectors API
- •JavaScript Logging and Debugging
- •window.JSON
- •DOM Level 3
- •Monkeys, Squirrelfish, and Other Speedy Oddities
- •Summary
- •Using the Canvas API
- •Overview of HTML5 Canvas
- •History
- •What Is a Canvas?
- •Canvas Coordinates
- •When Not to Use Canvas
- •Fallback Content
- •CSS and Canvas
- •Browser Support for HTML5 Canvas
- •Using the HTML5 Canvas APIs
- •Checking for Browser Support
- •Adding a Canvas to a Page
- •Applying Transformations to Drawings
- •Working with Paths
- •Working with Stroke Styles
- •Working with Fill Styles
- •Filling Rectangular Content
- •Drawing Curves
- •Inserting Images into a Canvas
- •Using Gradients
- •Using Background Patterns
- •Scaling Canvas Objects
- •Using Canvas Transforms
- •Using Canvas Text
- •Applying Shadows
- •Working with Pixel Data
- •Implementing Canvas Security
- •Building an Application with HTML5 Canvas
- •Practical Extra: Full Page Glass Pane
- •Practical Extra: Timing Your Canvas Animation
- •Summary
- •Working with Scalable Vector Graphics
- •Overview of SVG
- •History
- •Understanding SVG
- •Scalable Graphics
- •Creating 2D Graphics with SVG
- •Adding SVG to a Page
- •Simple Shapes
- •Transforming SVG Elements
- •Reusing Content
- •Patterns and Gradients
- •SVG Paths
- •Using SVG Text
- •Putting the Scene Together
- •Building an Interactive Application with SVG
- •Adding Trees
- •Adding the updateTrees Function
- •Adding the removeTree Function
- •Adding the CSS Styles
- •The Final Code
- •Summary
- •Working with Audio and Video
- •Overview of Audio and Video
- •Video Containers
- •Audio and Video Codecs
- •Audio and Video Restrictions
- •Browser Support for Audio and Video
- •Using the Audio and Video API
- •Checking for Browser Support
- •Accessibility
- •Understanding Media Elements
- •Working with Audio
- •Working with Video
- •Practical Extras
- •Summary
- •Using the Geolocation API
- •About Location Information
- •Latitude and Longitude Coordinates
- •Where Does Location Information Come From?
- •IP Address Geolocation Data
- •GPS Geolocation Data
- •Wi-Fi Geolocation Data
- •Cell Phone Geolocation Data
- •User–Defined Geolocation Data
- •Browser Support for Geolocation
- •Privacy
- •Triggering the Privacy Protection Mechanism
- •Dealing with Location Information
- •Using the Geolocation API
- •Checking for Browser Support
- •Position Requests
- •Building an Application with Geolocation
- •Writing the HTML Display
- •Processing the Geolocation Data
- •The Final Code
- •Practical Extras
- •What’s My Status?
- •Show Me on a Google Map
- •Summary
- •Using the Communication APIs
- •Cross Document Messaging
- •Understanding Origin Security
- •Browser Support for Cross Document Messaging
- •Using the postMessage API
- •Building an Application Using the postMessage API
- •XMLHttpRequest Level 2
- •Cross-Origin XMLHttpRequest
- •Progress Events
- •Browser Support for HTML5 XMLHttpRequest Level 2
- •Using the XMLHttpRequest API
- •Building an Application Using XMLHttpRequest
- •Practical Extras
- •Structured Data
- •Framebusting
- •Summary
- •Using the WebSocket API
- •Overview of WebSocket
- •Real-Time and HTTP
- •Understanding WebSocket
- •Writing a Simple Echo WebSocket Server
- •Using the WebSocket API
- •Checking for Browser Support
- •Basic API Usage
- •Building a WebSocket Application
- •Coding the HTML File
- •Adding the WebSocket Code
- •Adding the Geolocation Code
- •Putting It All Together
- •The Final Code
- •Summary
- •Using the Forms API
- •Overview of HTML5 Forms
- •HTML Forms Versus XForms
- •Functional Forms
- •Browser Support for HTML5 Forms
- •An Input Catalog
- •Using the HTML5 Forms APIs
- •New Form Attributes and Functions
- •Checking Forms with Validation
- •Validation Feedback
- •Building an Application with HTML5 Forms
- •Practical Extras
- •Summary
- •Working with Drag-and-Drop
- •Web Drag-and-Drop: The Story So Far
- •Overview of HTML5 Drag-and-Drop
- •The Big Picture
- •Events to Remember
- •Drag Participation
- •Transfer and Control
- •Building an Application with Drag-and-Drop
- •Getting Into the dropzone
- •Handling Drag-and-Drop for Files
- •Practical Extras
- •Customizing the Drag Display
- •Summary
- •Using the Web Workers API
- •Browser Support for Web Workers
- •Using the Web Workers API
- •Checking for Browser Support
- •Creating Web Workers
- •Loading and Executing Additional JavaScript
- •Communicating with Web Workers
- •Coding the Main Page
- •Handling Errors
- •Stopping Web Workers
- •Using Web Workers within Web Workers
- •Using Timers
- •Example Code
- •Building an Application with Web Workers
- •Coding the blur.js Helper Script
- •Coding the blur.html Application Page
- •Coding the blurWorker.js Web Worker Script
- •Communicating with the Web Workers
- •The Application in Action
- •Example Code
- •Summary
- •Using the Storage APIs
- •Overview of Web Storage
- •Browser Support for Web Storage
- •Using the Web Storage API
- •Checking for Browser Support
- •Setting and Retrieving Values
- •Plugging Data Leaks
- •Local Versus Session Storage
- •Other Web Storage API Attributes and Functions
- •Communicating Web Storage Updates
- •Exploring Web Storage
- •Building an Application with Web Storage
- •The Future of Browser Database Storage
- •The Web SQL Database
- •The Indexed Database API
- •Practical Extras
- •JSON Object Storage
- •A Window into Sharing
- •Summary
- •Overview of HTML5 Offline Web Applications
- •Browser Support for HTML5 Offline Web Applications
- •Using the HTML5 Application Cache API
- •Checking for Browser Support
- •Creating a Simple Offline Application
- •Going Offline
- •Manifest Files
- •The ApplicationCache API
- •Application Cache in Action
- •Building an Application with HTML5 Offline Web Applications
- •Creating a Manifest File for the Application Resources
- •Creating the HTML Structure and CSS for the UI
- •Creating the Offline JavaScript
- •Check for ApplicationCache Support
- •Adding the Update Button Handler
- •Add Geolocation Tracking Code
- •Adding Storage Code
- •Adding Offline Event Handling
- •Summary
- •The Future of HTML5
- •Browser Support for HTML5
- •HTML Evolves
- •WebGL
- •Devices
- •Audio Data API
- •Touchscreen Device Events
- •Peer-to-Peer Networking
- •Ultimate Direction
- •Summary
- •Index

CHAPTER 11 USING THE STORAGE APIS
Local Versus Session Storage
Sometimes, an application needs values that persist beyond the life of a single tab or window or need to be shared across multiple views. In these cases, it is more appropriate to use a different Web Storage implementation: localStorage. The good news is that you already know how to use localStorage. The only programmatic difference between sessionStorage and localStorage is the name by which each is accessed—through the sessionStorage and localStorage objects, respectively. The primary behavioral differences are how long the values persist and how they are shared. Table 11-1 shows the differences between the two types of storage.
Table 11-1. Differences Between sessionStorage and localStorage
sessionStorage |
localStorage |
Values persist only as long as the window or tab |
Values persist beyond window and browser lifetimes. |
in which they were stored (survives a browser |
|
refresh; not a browser restart). |
|
Values are only visible within the window or tab that created them.
Values are shared across every window or tab running at the same origin.
Keep in mind that browsers sometimes redefine the lifespan of a tab or window. For example, some browsers will save and restore the current session when a browser crashes, or when a user shuts down the display with many open tabs. In these cases, the browser may choose to keep the sessionStorage around when the browser restarts or resumes. So, in effect, sessionStorage may live longer than you think!
Other Web Storage API Attributes and Functions
The Web Storage API is one of the simplest in the HTML5 set. We have already looked at both explicit and implicit ways to set and retrieve data from the session and local storage areas. Let’s complete our survey of the API by looking at the full set of available attributes and function calls.
The sessionStorage and localStorage objects can be retrieved from the window object of the document in which they are being used. Other than their names and the duration of their values, they are identical in functionality. Both implement the Storage interface, which is shown in Listing 11-2.
Listing 11-2. The Storage Interface
interface Storage {
readonly attribute unsigned long length; getter DOMString key(in unsigned long index); getter any getItem(in DOMString key);
setter creator void setItem(in DOMString key, in any data); deleter void removeItem(in DOMString key);
void clear();
};
Let’s look at the attributes and functions here in more detail.
269

CHAPTER 11 USING THE STORAGE APIS
•The length attribute specifies how many key-value pairs are currently stored in the storage object. Remember that storage objects are specific to their origin, so that implies that the items (and length) of the storage object only reflect the items stored for the current origin.
•The key(index) function allows retrieval of a given key. Generally, this is most useful when you wish to iterate across all the keys in a particular storage object. Keys are zero-based, meaning that the first key is at index (0) and the last key is at index (length – 1). Once a key is retrieved, it can be used to fetch its corresponding value. Keys will retain their indices over the life of a given storage object unless a key or one of its predecessors is removed.
•As you’ve already seen, getItem(key) function is one way to retrieve the value based on a given key. The other is to reference the key as an array index to the storage object. In both cases, the value null will be returned if the key does not exist in storage.
•Similarly, setItem(key, value) function will put a value into storage under the specified key name, or replace an existing value if one already exists under that key name. Note that it is possible to receive an error when setting an item value; if the user has storage turned off for that site, or if the storage is already filled to its maximum amount, a QUOTA_EXCEEDED_ERR error will be thrown during the attempt. Make sure to handle such an error should your application depend on proper storage behavior.
Figure 11-3. Quota Exceeded Error in Chrome
270
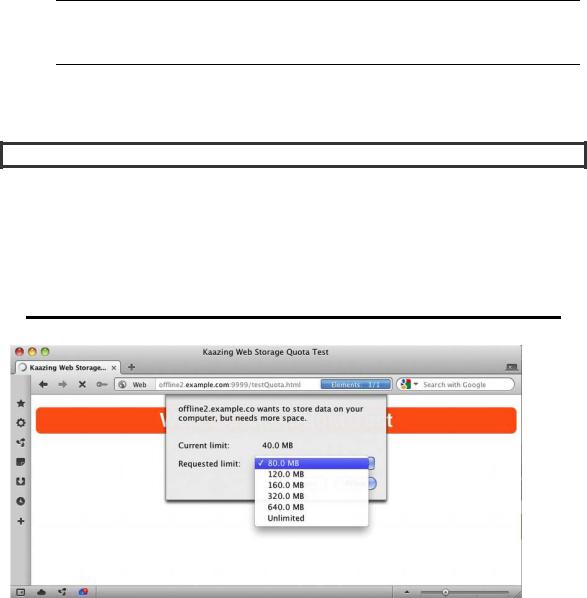
CHAPTER 11 USING THE STORAGE APIS
•The removeItem(key) function does exactly as you might expect. If a value is currently in storage under the specified key, this call will remove it. If no item was stored under that key, no action is taken.
Note Unlike some collection and data frameworks, removing an item does not return the old value as a result of the call to remove it. Make sure you’ve stored any copy you need independent of the removal.
•Finally, the clear() function removes all values from the storage list. It is safe to call this on an empty storage object; as such, a call will simply do nothing.
DISK SPACE QUOTA
Peter says: “The specification recommends that browsers allow five megabytes per origin. Browsers should prompt the user when the quota is reached in order to grant more space and may also provide ways for users to see how much space each origin is using.
In reality, the behavior is still a bit inconsistent. Some browsers silently allow a larger quota or prompt for a space increase, while others simply throw the QUOTA_EXCEEDED_ERR error shown in Figure 11-3, while others, like Opera, shown in Figure 11-4, implement a nice way to allocate more quota on the fly. The test file testQuota.html used in this example is located in the code/storage directory.”
Figure 11-4. On-the-fly Quota increase in Opera
271

CHAPTER 11 USING THE STORAGE APIS
Communicating Web Storage Updates
Sometimes, things get a little more complicated, and storage needs to be accessed by more than one page, browser tab, or worker. Perhaps your application needs to trigger many operations in succession whenever a storage value is changed. For just these cases, the Web Storage API includes an event mechanism to allow notifications of data updates to be communicated to interested listeners. Web Storage events are fired on the window object for every window of the same origin as the storage operation, regardless of whether or not the listening window is doing any storage operations itself.
Note Web Storage events can be used to communicate between windows on the same origin. This will be explored a bit more thoroughly in the “Practical Extras” section.
To register to receive the storage events of a window’s origin, simply register an event listener, for example:
window.addEventListener("storage", displayStorageEvent, true);
As you can see, the name storage is used to indicate interest in storage events. Any time a Storage event—either sessionStorage or localStorage—for that origin is raised any registered event listener will receive the storage event as the specified event handler. The storage event itself takes the form shown in Listing 11-3.
Listing 11-3. The StorageEvent Interface
interface StorageEvent : Event { readonly attribute DOMString key; readonly attribute any oldValue; readonly attribute any newValue; readonly attribute DOMString url;
readonly attribute Storage storageArea;
};
The StorageEvent object will be the first object passed to the event handler, and it contains all the information necessary to understand the nature of the storage change.
•The key attribute contains the key value that was updated or removed in the storage.
•The oldValue contains the previous value corresponding to the key before it was updated, and the newValue contains the value after the change. If the value was newly added, the oldValue will be null, and if the value has been removed, the newValue will be null.
•The url will point to the origin where the storage event occurred.
•Finally, the storageArea provides a convenient reference to the sessionStorage or localStorage where the value was changed. This gives the handler an easy way to query the storage for current values or make changes based on other storage changes.
272