
- •Contents at a Glance
- •Contents
- •Foreword
- •About the Authors
- •About the Technical Reviewer
- •Acknowledgments
- •Introduction
- •Who This Book Is For
- •An Overview of This Book
- •Example Code and Companion Web Site
- •Contacting the Authors
- •Overview of HTML5
- •The Story So Far—The History of HTML5
- •The Myth of 2022 and Why It Doesn’t Matter
- •Who Is Developing HTML5?
- •A New Vision
- •Compatibility and Paving the Cow Paths
- •Utility and the Priority of Constituencies
- •Interoperability Simplification
- •Universal Access
- •A Plugin–Free Paradigm
- •What’s In and What’s Out?
- •What’s New in HTML5?
- •New DOCTYPE and Character Set
- •New and Deprecated Elements
- •Semantic Markup
- •Simplifying Selection Using the Selectors API
- •JavaScript Logging and Debugging
- •window.JSON
- •DOM Level 3
- •Monkeys, Squirrelfish, and Other Speedy Oddities
- •Summary
- •Using the Canvas API
- •Overview of HTML5 Canvas
- •History
- •What Is a Canvas?
- •Canvas Coordinates
- •When Not to Use Canvas
- •Fallback Content
- •CSS and Canvas
- •Browser Support for HTML5 Canvas
- •Using the HTML5 Canvas APIs
- •Checking for Browser Support
- •Adding a Canvas to a Page
- •Applying Transformations to Drawings
- •Working with Paths
- •Working with Stroke Styles
- •Working with Fill Styles
- •Filling Rectangular Content
- •Drawing Curves
- •Inserting Images into a Canvas
- •Using Gradients
- •Using Background Patterns
- •Scaling Canvas Objects
- •Using Canvas Transforms
- •Using Canvas Text
- •Applying Shadows
- •Working with Pixel Data
- •Implementing Canvas Security
- •Building an Application with HTML5 Canvas
- •Practical Extra: Full Page Glass Pane
- •Practical Extra: Timing Your Canvas Animation
- •Summary
- •Working with Scalable Vector Graphics
- •Overview of SVG
- •History
- •Understanding SVG
- •Scalable Graphics
- •Creating 2D Graphics with SVG
- •Adding SVG to a Page
- •Simple Shapes
- •Transforming SVG Elements
- •Reusing Content
- •Patterns and Gradients
- •SVG Paths
- •Using SVG Text
- •Putting the Scene Together
- •Building an Interactive Application with SVG
- •Adding Trees
- •Adding the updateTrees Function
- •Adding the removeTree Function
- •Adding the CSS Styles
- •The Final Code
- •Summary
- •Working with Audio and Video
- •Overview of Audio and Video
- •Video Containers
- •Audio and Video Codecs
- •Audio and Video Restrictions
- •Browser Support for Audio and Video
- •Using the Audio and Video API
- •Checking for Browser Support
- •Accessibility
- •Understanding Media Elements
- •Working with Audio
- •Working with Video
- •Practical Extras
- •Summary
- •Using the Geolocation API
- •About Location Information
- •Latitude and Longitude Coordinates
- •Where Does Location Information Come From?
- •IP Address Geolocation Data
- •GPS Geolocation Data
- •Wi-Fi Geolocation Data
- •Cell Phone Geolocation Data
- •User–Defined Geolocation Data
- •Browser Support for Geolocation
- •Privacy
- •Triggering the Privacy Protection Mechanism
- •Dealing with Location Information
- •Using the Geolocation API
- •Checking for Browser Support
- •Position Requests
- •Building an Application with Geolocation
- •Writing the HTML Display
- •Processing the Geolocation Data
- •The Final Code
- •Practical Extras
- •What’s My Status?
- •Show Me on a Google Map
- •Summary
- •Using the Communication APIs
- •Cross Document Messaging
- •Understanding Origin Security
- •Browser Support for Cross Document Messaging
- •Using the postMessage API
- •Building an Application Using the postMessage API
- •XMLHttpRequest Level 2
- •Cross-Origin XMLHttpRequest
- •Progress Events
- •Browser Support for HTML5 XMLHttpRequest Level 2
- •Using the XMLHttpRequest API
- •Building an Application Using XMLHttpRequest
- •Practical Extras
- •Structured Data
- •Framebusting
- •Summary
- •Using the WebSocket API
- •Overview of WebSocket
- •Real-Time and HTTP
- •Understanding WebSocket
- •Writing a Simple Echo WebSocket Server
- •Using the WebSocket API
- •Checking for Browser Support
- •Basic API Usage
- •Building a WebSocket Application
- •Coding the HTML File
- •Adding the WebSocket Code
- •Adding the Geolocation Code
- •Putting It All Together
- •The Final Code
- •Summary
- •Using the Forms API
- •Overview of HTML5 Forms
- •HTML Forms Versus XForms
- •Functional Forms
- •Browser Support for HTML5 Forms
- •An Input Catalog
- •Using the HTML5 Forms APIs
- •New Form Attributes and Functions
- •Checking Forms with Validation
- •Validation Feedback
- •Building an Application with HTML5 Forms
- •Practical Extras
- •Summary
- •Working with Drag-and-Drop
- •Web Drag-and-Drop: The Story So Far
- •Overview of HTML5 Drag-and-Drop
- •The Big Picture
- •Events to Remember
- •Drag Participation
- •Transfer and Control
- •Building an Application with Drag-and-Drop
- •Getting Into the dropzone
- •Handling Drag-and-Drop for Files
- •Practical Extras
- •Customizing the Drag Display
- •Summary
- •Using the Web Workers API
- •Browser Support for Web Workers
- •Using the Web Workers API
- •Checking for Browser Support
- •Creating Web Workers
- •Loading and Executing Additional JavaScript
- •Communicating with Web Workers
- •Coding the Main Page
- •Handling Errors
- •Stopping Web Workers
- •Using Web Workers within Web Workers
- •Using Timers
- •Example Code
- •Building an Application with Web Workers
- •Coding the blur.js Helper Script
- •Coding the blur.html Application Page
- •Coding the blurWorker.js Web Worker Script
- •Communicating with the Web Workers
- •The Application in Action
- •Example Code
- •Summary
- •Using the Storage APIs
- •Overview of Web Storage
- •Browser Support for Web Storage
- •Using the Web Storage API
- •Checking for Browser Support
- •Setting and Retrieving Values
- •Plugging Data Leaks
- •Local Versus Session Storage
- •Other Web Storage API Attributes and Functions
- •Communicating Web Storage Updates
- •Exploring Web Storage
- •Building an Application with Web Storage
- •The Future of Browser Database Storage
- •The Web SQL Database
- •The Indexed Database API
- •Practical Extras
- •JSON Object Storage
- •A Window into Sharing
- •Summary
- •Overview of HTML5 Offline Web Applications
- •Browser Support for HTML5 Offline Web Applications
- •Using the HTML5 Application Cache API
- •Checking for Browser Support
- •Creating a Simple Offline Application
- •Going Offline
- •Manifest Files
- •The ApplicationCache API
- •Application Cache in Action
- •Building an Application with HTML5 Offline Web Applications
- •Creating a Manifest File for the Application Resources
- •Creating the HTML Structure and CSS for the UI
- •Creating the Offline JavaScript
- •Check for ApplicationCache Support
- •Adding the Update Button Handler
- •Add Geolocation Tracking Code
- •Adding Storage Code
- •Adding Offline Event Handling
- •Summary
- •The Future of HTML5
- •Browser Support for HTML5
- •HTML Evolves
- •WebGL
- •Devices
- •Audio Data API
- •Touchscreen Device Events
- •Peer-to-Peer Networking
- •Ultimate Direction
- •Summary
- •Index
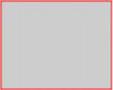
CHAPTER 3 WORKING WITH SCALABLE VECTOR GRAPHICS
Adding SVG to a Page
Adding inline SVG to an HTML page is as simple as adding any other element.
There are several ways to use SVG on the Web, including as <img> elements. We will use inline SVG in HTML, because it will integrate into the HTML document. That will let us later write an interactive application that seamlessly combines HTML, JavaScript, and SVG (see Listing 3-1).
Listing 3-1. SVG Containing a Red Rectangle
<!doctype html>
<svg width="200" height="200"> </svg>
That’s it! No XML namespace necessary. Now, between the start and end svg tags, we can add shapes and other visual objects. If you want to split the SVG content out into a separate .svg file, you will need to change it like so:
<svg width="400" height="600" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink">
</svg>
Now it is a valid XML document with the proper namespace attributes. You will be able to open that document with a wide variety of image viewers and editors. You can also refer to an SVG file from HTML as a static image with code such as <img src="example.svg">. One downside to that approach is that the SVG document is not integrated into the DOM the way inline SVG content is. You won’t be able to script interaction with the SVG elements.
Simple Shapes
The SVG language includes basic shape elements such as rectangles, circles, and ellipses. The size and position of shape elements are defined with attributes. For rectangles, these are width and height. For circles, there is an r attribute for radius. All of these use the CSS syntax for distances, so they can be pixels, points, ems, and so on. Listing 3-2 is a very short HTML document containing inline SVG. It is just a gray rectangle with a red outline that is 100 pixels by 80 pixels in size, and it is displayed in Figure 3-4.
Listing 3-2. SVG Containing a Red Rectangle
<!doctype html>
<svg width="200" height="200">
<rect x="10" y="20" width="100" height="80" stroke="red" fill="#ccc" /> </svg>
Figure 3-4. An SVG rectangle in an HTML document
67

CHAPTER 3 WORKING WITH SCALABLE VECTOR GRAPHICS
SVG draws objects in the order they appear in the document. If we add a circle after the rectangle, it appears on top of the first shape. We will give that circle an 8 pixel wide blue stroke and no fill style (see Listing 3-3), so it stands out, as shown in Figure 3-5.
Listing 3-3. A Rectangle and a Circle
<!doctype html>
<svg width="200" height="200">
<rect x="10" y="20" width="100" height="80" stroke="red" fill="#ccc" /> <circle cx="120" cy="80" r="40" stroke="#00f" fill="none" stroke-width="8" />
</svg>
Figure 3-5. A rectangle and a circle
Note that the x and y attributes define the position of the top-left corner of the rectangle. The circle, on the other hand, has cx and cy attributes, which are the x and y values for the center of the circle. SVG uses the same coordinate system as the canvas API. The top-left corner of the svg element is position 0,0. See Chapter 2 for the details of the canvas coordinate system.
Transforming SVG Elements
There are organizational elements in SVG intended to combine multiple elements so that they can be transformed or linked to as units. The <g> element stands for “group.” Groups can be used to combine multiple related elements. As a group, they can be referred to by a common ID. A group can also be transformed as a unit. If you add a transform attribute to a group, all of that group’s contents are transformed. The transform attribute can include commands to rotate (see Listing 3-4 and Figure 3-6), translate, scale, and skew. You can also specify a transformation matrix, just as you can with the canvas API.
Listing 3-4. A Rectangle and a Circle Within a Rotated Group
<svg width="200" height="200">
<g transform="translate(60,0) rotate(30) scale(0.75)" id="ShapeGroup"> <rect x="10" y="20" width="100" height="80" stroke="red" fill="#ccc" />
<circle cx="120" cy="80" r="40" stroke="#00f" fill="none" stroke-width="8" /> </g>
</svg>
68
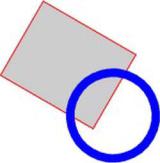
CHAPTER 3 WORKING WITH SCALABLE VECTOR GRAPHICS
Figure 3-6. A rotated group
Reusing Content
SVG has a <defs> element for defining content for future use. It also has an element named <use> that you can link to your definitions. This lets you reuse the same content multiple times and eliminate redundancy. Figure 3-7 shows a group used three times at different transformed positions and scales. The group has the id ShapeGroup, and it contains a rectangle and a circle. The actual rectangle and circle shapes are just defined the one time inside of the <defs> element. The defined group is not, by itself, visible. Instead, there are three <use> elements linked to the shape group, so three rectangles and three circles appear rendered on the page (see Listing 3-5).
Listing 3-5. Using a Group Three Times
<svg width="200" height="200"> <defs>
<g id="ShapeGroup">
<rect x="10" y="20" width="100" height="80" stroke="red" fill="#ccc" /> <circle cx="120" cy="80" r="40" stroke="#00f" fill="none" stroke-width="8" />
</g>
</defs>
<use xlink:href="#ShapeGroup" transform="translate(60,0) scale(0.5)"/> <use xlink:href="#ShapeGroup" transform="translate(120,80) scale(0.4)"/> <use xlink:href="#ShapeGroup" transform="translate(20,60) scale(0.25)"/>
</svg>
69
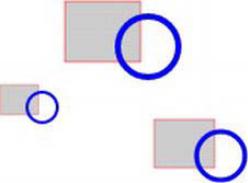
CHAPTER 3 WORKING WITH SCALABLE VECTOR GRAPHICS
Figure 3-7. Three use elements referencing the same group
Patterns and Gradients
The circle and rectangle in Figure 3-7 have simple fill and stroke styles. Objects can be painted with more complex styles, including gradients and patterns (see Listing 3-6). Gradients can be linear or radial. Patterns can be made up of pixel graphics or even other SVG elements. Figure 3-8 shows a rectangle with a linear color gradient as well as a circle with a gravel texture. The texture comes from a JPEG image that is linked to from an SVG image element.
Listing 3-6. Texturing the Rectangle and Circle
<!doctype html>
<svg width="200" height="200"> <defs>
<pattern id="GravelPattern" patternUnits="userSpaceOnUse"
x="0" y="0" width="100" height="67" viewBox="0 0 100 67">
<image x="0" y="0" width="100" height="67" xlink:href="gravel.jpg"></image> </pattern>
<linearGradient id="RedBlackGradient">
<stop offset="0%" stop-color="#000"></stop> <stop offset="100%" stop-color="#f00"></stop>
</linearGradient>
</defs>
<rect x="10" y="20" width="100" height="80" stroke="red" fill="url(#RedBlackGradient)" />
<circle cx="120" cy="80" r="40" stroke="#00f" stroke-width="8" fill="url(#GravelPattern)" />
</svg>
70