
- •Contents at a Glance
- •Contents
- •Foreword
- •About the Authors
- •About the Technical Reviewer
- •Acknowledgments
- •Introduction
- •Who This Book Is For
- •An Overview of This Book
- •Example Code and Companion Web Site
- •Contacting the Authors
- •Overview of HTML5
- •The Story So Far—The History of HTML5
- •The Myth of 2022 and Why It Doesn’t Matter
- •Who Is Developing HTML5?
- •A New Vision
- •Compatibility and Paving the Cow Paths
- •Utility and the Priority of Constituencies
- •Interoperability Simplification
- •Universal Access
- •A Plugin–Free Paradigm
- •What’s In and What’s Out?
- •What’s New in HTML5?
- •New DOCTYPE and Character Set
- •New and Deprecated Elements
- •Semantic Markup
- •Simplifying Selection Using the Selectors API
- •JavaScript Logging and Debugging
- •window.JSON
- •DOM Level 3
- •Monkeys, Squirrelfish, and Other Speedy Oddities
- •Summary
- •Using the Canvas API
- •Overview of HTML5 Canvas
- •History
- •What Is a Canvas?
- •Canvas Coordinates
- •When Not to Use Canvas
- •Fallback Content
- •CSS and Canvas
- •Browser Support for HTML5 Canvas
- •Using the HTML5 Canvas APIs
- •Checking for Browser Support
- •Adding a Canvas to a Page
- •Applying Transformations to Drawings
- •Working with Paths
- •Working with Stroke Styles
- •Working with Fill Styles
- •Filling Rectangular Content
- •Drawing Curves
- •Inserting Images into a Canvas
- •Using Gradients
- •Using Background Patterns
- •Scaling Canvas Objects
- •Using Canvas Transforms
- •Using Canvas Text
- •Applying Shadows
- •Working with Pixel Data
- •Implementing Canvas Security
- •Building an Application with HTML5 Canvas
- •Practical Extra: Full Page Glass Pane
- •Practical Extra: Timing Your Canvas Animation
- •Summary
- •Working with Scalable Vector Graphics
- •Overview of SVG
- •History
- •Understanding SVG
- •Scalable Graphics
- •Creating 2D Graphics with SVG
- •Adding SVG to a Page
- •Simple Shapes
- •Transforming SVG Elements
- •Reusing Content
- •Patterns and Gradients
- •SVG Paths
- •Using SVG Text
- •Putting the Scene Together
- •Building an Interactive Application with SVG
- •Adding Trees
- •Adding the updateTrees Function
- •Adding the removeTree Function
- •Adding the CSS Styles
- •The Final Code
- •Summary
- •Working with Audio and Video
- •Overview of Audio and Video
- •Video Containers
- •Audio and Video Codecs
- •Audio and Video Restrictions
- •Browser Support for Audio and Video
- •Using the Audio and Video API
- •Checking for Browser Support
- •Accessibility
- •Understanding Media Elements
- •Working with Audio
- •Working with Video
- •Practical Extras
- •Summary
- •Using the Geolocation API
- •About Location Information
- •Latitude and Longitude Coordinates
- •Where Does Location Information Come From?
- •IP Address Geolocation Data
- •GPS Geolocation Data
- •Wi-Fi Geolocation Data
- •Cell Phone Geolocation Data
- •User–Defined Geolocation Data
- •Browser Support for Geolocation
- •Privacy
- •Triggering the Privacy Protection Mechanism
- •Dealing with Location Information
- •Using the Geolocation API
- •Checking for Browser Support
- •Position Requests
- •Building an Application with Geolocation
- •Writing the HTML Display
- •Processing the Geolocation Data
- •The Final Code
- •Practical Extras
- •What’s My Status?
- •Show Me on a Google Map
- •Summary
- •Using the Communication APIs
- •Cross Document Messaging
- •Understanding Origin Security
- •Browser Support for Cross Document Messaging
- •Using the postMessage API
- •Building an Application Using the postMessage API
- •XMLHttpRequest Level 2
- •Cross-Origin XMLHttpRequest
- •Progress Events
- •Browser Support for HTML5 XMLHttpRequest Level 2
- •Using the XMLHttpRequest API
- •Building an Application Using XMLHttpRequest
- •Practical Extras
- •Structured Data
- •Framebusting
- •Summary
- •Using the WebSocket API
- •Overview of WebSocket
- •Real-Time and HTTP
- •Understanding WebSocket
- •Writing a Simple Echo WebSocket Server
- •Using the WebSocket API
- •Checking for Browser Support
- •Basic API Usage
- •Building a WebSocket Application
- •Coding the HTML File
- •Adding the WebSocket Code
- •Adding the Geolocation Code
- •Putting It All Together
- •The Final Code
- •Summary
- •Using the Forms API
- •Overview of HTML5 Forms
- •HTML Forms Versus XForms
- •Functional Forms
- •Browser Support for HTML5 Forms
- •An Input Catalog
- •Using the HTML5 Forms APIs
- •New Form Attributes and Functions
- •Checking Forms with Validation
- •Validation Feedback
- •Building an Application with HTML5 Forms
- •Practical Extras
- •Summary
- •Working with Drag-and-Drop
- •Web Drag-and-Drop: The Story So Far
- •Overview of HTML5 Drag-and-Drop
- •The Big Picture
- •Events to Remember
- •Drag Participation
- •Transfer and Control
- •Building an Application with Drag-and-Drop
- •Getting Into the dropzone
- •Handling Drag-and-Drop for Files
- •Practical Extras
- •Customizing the Drag Display
- •Summary
- •Using the Web Workers API
- •Browser Support for Web Workers
- •Using the Web Workers API
- •Checking for Browser Support
- •Creating Web Workers
- •Loading and Executing Additional JavaScript
- •Communicating with Web Workers
- •Coding the Main Page
- •Handling Errors
- •Stopping Web Workers
- •Using Web Workers within Web Workers
- •Using Timers
- •Example Code
- •Building an Application with Web Workers
- •Coding the blur.js Helper Script
- •Coding the blur.html Application Page
- •Coding the blurWorker.js Web Worker Script
- •Communicating with the Web Workers
- •The Application in Action
- •Example Code
- •Summary
- •Using the Storage APIs
- •Overview of Web Storage
- •Browser Support for Web Storage
- •Using the Web Storage API
- •Checking for Browser Support
- •Setting and Retrieving Values
- •Plugging Data Leaks
- •Local Versus Session Storage
- •Other Web Storage API Attributes and Functions
- •Communicating Web Storage Updates
- •Exploring Web Storage
- •Building an Application with Web Storage
- •The Future of Browser Database Storage
- •The Web SQL Database
- •The Indexed Database API
- •Practical Extras
- •JSON Object Storage
- •A Window into Sharing
- •Summary
- •Overview of HTML5 Offline Web Applications
- •Browser Support for HTML5 Offline Web Applications
- •Using the HTML5 Application Cache API
- •Checking for Browser Support
- •Creating a Simple Offline Application
- •Going Offline
- •Manifest Files
- •The ApplicationCache API
- •Application Cache in Action
- •Building an Application with HTML5 Offline Web Applications
- •Creating a Manifest File for the Application Resources
- •Creating the HTML Structure and CSS for the UI
- •Creating the Offline JavaScript
- •Check for ApplicationCache Support
- •Adding the Update Button Handler
- •Add Geolocation Tracking Code
- •Adding Storage Code
- •Adding Offline Event Handling
- •Summary
- •The Future of HTML5
- •Browser Support for HTML5
- •HTML Evolves
- •WebGL
- •Devices
- •Audio Data API
- •Touchscreen Device Events
- •Peer-to-Peer Networking
- •Ultimate Direction
- •Summary
- •Index
CHAPTER 11 USING THE STORAGE APIS
•Cookies are transmitted back and forth from server to browser on every request scoped to that cookie. Not only does this mean that cookie data is visible on the network, making them a security risk when not encrypted, but also that any data persisted as cookies will be consuming network bandwidth every time a URL is loaded. As such, the relatively small size of cookies makes more sense.
In many cases, the same results could be achieved without involving a network or remote server. This is where the HTML5 Web Storage API comes in. By using this simple API, developers can store values in easily retrievable JavaScript objects that persist across page loads. By using either sessionStorage or localStorage, developers can choose to let those values survive either across page loads in a single window or tab or across browser restarts, respectively. Stored data is not transmitted across the network, and is easily accessed on return visits to a page. Furthermore, larger values can be persisted using the Web Storage API values as high as a few megabytes. This makes Web Storage suitable for document and file data that would quickly blow out the size limit of a cookie.
Browser Support for Web Storage
Web Storage is one of the most widely adopted features of HTML5. In fact, since the arrival of Internet Explorer 8 in 2009 all currently shipping browser versions support Web Storage in some capacity. At the time of this publication, the market share of browsers that do not support storage is dwindling down into single digit percentages.
Web Storage is one of the safest new APIs to use in your web applications today because of its widespread support. As usual, though, it is a good idea to first test if Web Storage is supported before you use it. The subsequent section “Checking for Browser Support” will show you how you can programmatically check if Web Storage is supported.
Using the Web Storage API
The Web Storage API is surprisingly simple to use. We’ll start by covering basic storage and retrieval of values and then move on to the differences between sessionStorage and localStorage. Finally, we’ll look at the more advanced aspects of the API, such as event notification when values change.
Checking for Browser Support
The storage database for a given domain is accessed directly from the window object. Therefore, determining if a user’s browser supports the Web Storage API is as easy as checking for the existence of window.sessionStorage or window.localStorage. Listing 11-1 shows a routine that checks for storage support and displays a message about the browser’s support for the Web Storage API. Instead of using this code, you can also use the JavaScript utility library Modernizr, which handles some cases that may result in a false positive.
264
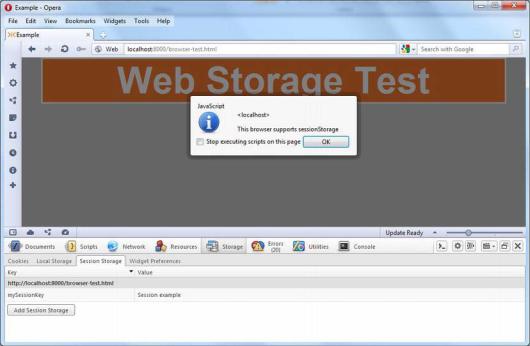
CHAPTER 11 USING THE STORAGE APIS
Listing 11-1. Checking for Web Storage Support
function checkStorageSupport() {
//sessionStorage
if (window.sessionStorage) {
alert('This browser supports sessionStorage');
}else {
alert('This browser does NOT support sessionStorage');
}
//localStorage
if (window.localStorage) {
alert('This browser supports localStorage'); } else {
alert('This browser does NOT support localStorage');
}
}
Figure 11-1 shows this check for storage support in action.
Figure 11-1. Checking for browser support in Opera
265

CHAPTER 11 USING THE STORAGE APIS
Some browsers do not support sessionStorage for files accessed directly from the file system. Make sure you serve up the pages from a web server when you run the examples in this chapter! For example, you can start Python’s simple HTTP server in the code/storage directory as follows:
python -m SimpleHTTPServer 9999
After that, you can access the files at http://localhost:9999/. For example,
http://localhost:9999/browser-test.html.
However, you are free to use any server or URL location to run the examples.
Note If a user is browsing with his browser set to “private” mode, then localStorage values will not actually persist once the browser is shut down. This is by design, as users of this mode have explicitly chosen to leave no traces behind. Nonetheless, your application should respond gracefully if storage values are not available in a later browsing session.
Setting and Retrieving Values
To begin, we’ll focus on the session storage capability as you learn to set and retrieve simple values in a page. Setting a value can easily be done in a single statement, which we’ll initially write using the longhand notation:
sessionStorage.setItem(‘myFirstKey’, ‘myFirstValue’);
There are a few important points to notice from this storage access statement:
•We can omit the reference to the window for a shorthand notation, as the storage objects are made available in the default page context.
•The function we are calling is setItem, which takes a key string and a value string. Although some browsers might support passing in nonstring values, the specification only allows strings as values.
•This particular call will set into the session storage the string myFirstValue, which can later be retrieved by the key myFirstKey.
To retrieve the value, the long-hand notation involves making a call to the getItem function. For example, if we augmented our previous example with the following statement
alert(sessionStorage.getItem(‘myFirstKey’));
The browser raises a JavaScript alert displaying the text myFirstValue. As you can see, setting and retrieving values from the Web Storage API is very straightforward.
However, there is an even simpler way to access the storage objects in your code. You are also able to use expando-properties to set values in storage. Using this approach, the setItem and getItem calls can be avoided entirely by simply setting and retrieving values corresponding to the key-value pair directly on the sessionStorage object. Using this approach, our value set call can be rewritten as follows:
sessionStorage.myFirstKey = ‘myFirstValue’;
Or even
266
CHAPTER 11 USING THE STORAGE APIS
sessionStorage[‘myFirstKey’] = ‘myFirstValue’;
Similarly, the value retrieval call can be rewritten as:
alert(sessionStorage.myFirstKey);
We’ll use these formats interchangeably in the chapter for the sake of readability.
That’s it for the basics. You now have all the knowledge you need to use session storage in your application. However, you might be wondering what’s so special about this sessionStorage object. After all, JavaScript allows you to set and get properties on nearly any object. The difference is in the scope. What you may not have realized is that our example set and get calls do not need to occur in the same web page. As long as pages are served from the same origin—the combination of scheme, host, and port—then values set on sessionStorage can be retrieved from other pages using the same keys. This also applies to subsequent loads of the same page. As a developer, you are probably used to the idea that changes made in script will disappear whenever a page is reloaded. That is no longer true for values that are set in the Web Storage API; they will continue to exist across page loads.
Plugging Data Leaks
How long do the values persist? For objects set into sessionStorage, they will persist as long as the browser window (or tab) is not closed. As soon as a user closes the window—or browser, for that matter—the sessionStorage values are cleared out. It is useful to consider a sessionStorage value to be somewhat like a sticky note reminder. Values put into sessionStorage won’t last long, and you should not put anything truly valuable into them, as the values are not guaranteed to be around whenever you are looking for them.
Why, then, would you choose to use the session storage area in your web application? Session storage is perfect for short-lived processes that would normally be represented in wizards or dialogs. If you have data to store over the course of a few pages, that you would not be keen to have resurface the next time a user visits your application, feel free to store them in the session storage area. In the past, these types of values might be submitted by forms and cookies and transmitted back and forth on every page load. Using storage eliminates that overhead.
The sessionStorage API has another very specific use that solves a problem that has plagued many web-applications: scoping of values. Take, for example, a shopping application that lets you purchase airline tickets. In such an application, preference data such as the ideal departure date and return date could be sent back and forth from browser to server using cookies. This allows the server to remember previous choices as the user moves through the application, picking seats and a choice of meals.
However, it is very common for users to open multiple windows as they shop for travel deals, comparing flights from different vendors for the same departure time. This causes problems in a cookie system, because if a user switches back and forth between browser windows while comparing prices and availability, they are likely to set cookie values in one window that will be unexpectedly applied to another window served from the same URL on its next operation. This is sometimes referred to as leaking data and is caused by the fact that cookies are shared based on the origin where they are stored. Figure 11-2 shows how this can play out.
267
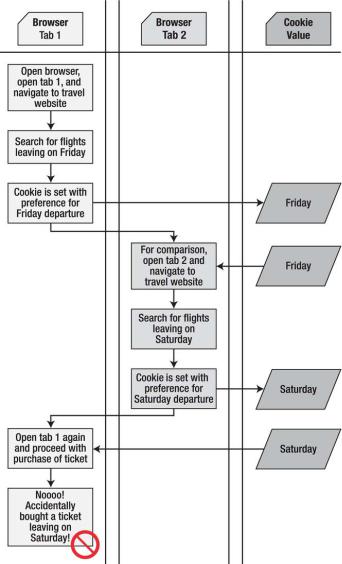
CHAPTER 11 USING THE STORAGE APIS
Figure 11-2. Data leakage while using a travel site to compare prices
Using sessionStorage, on the other hand, allows temporary values like a departure date to be saved across pages that access the application but not leak into other windows where the user is also browsing for flights. Therefore, those preferences will be isolated to each window where the corresponding flights are booked.
268