
- •Acknowledgments
- •Preface
- •Who Should Read This Book
- •What’s in This Book
- •Arduino Uno and the Arduino Platform
- •Code Examples and Conventions
- •Online Resources
- •The Parts You Need
- •Starter Packs
- •Complete Parts List
- •1. Welcome to the Arduino
- •What You Need
- •What Exactly Is an Arduino?
- •Exploring the Arduino Board
- •Installing the Arduino IDE
- •Meeting the Arduino IDE
- •Hello, World!
- •Compiling and Uploading Programs
- •What If It Doesn’t Work?
- •Exercises
- •2. Creating Bigger Projects with the Arduino
- •What You Need
- •Managing Projects and Sketches
- •Changing Preferences
- •Using Serial Ports
- •What If It Doesn’t Work?
- •Exercises
- •3. Building Binary Dice
- •What You Need
- •Working with Breadboards
- •Using an LED on a Breadboard
- •First Version of a Binary Die
- •Working with Buttons
- •Adding Your Own Button
- •Building a Dice Game
- •What If It Doesn’t Work?
- •Exercises
- •4. Building a Morse Code Generator Library
- •What You Need
- •Learning the Basics of Morse Code
- •Building a Morse Code Generator
- •Fleshing Out the Morse Code Generator’s Interface
- •Outputting Morse Code Symbols
- •Installing and Using the Telegraph Class
- •Publishing Your Own Library
- •What If It Doesn’t Work?
- •Exercises
- •5. Sensing the World Around Us
- •What You Need
- •Measuring Distances with an Ultrasonic Sensor
- •Increasing Precision Using Floating-Point Numbers
- •Increasing Precision Using a Temperature Sensor
- •Creating Your Own Dashboard
- •What If It Doesn’t Work?
- •Exercises
- •What You Need
- •Wiring Up the Accelerometer
- •Bringing Your Accelerometer to Life
- •Finding and Polishing Edge Values
- •Building Your Own Game Controller
- •More Projects
- •What If It Doesn’t Work?
- •Exercises
- •7. Writing a Game for the Motion-Sensing Game Controller
- •Writing a GameController Class
- •Creating the Game
- •What If It Doesn’t Work?
- •Exercises
- •8. Generating Video Signals with an Arduino
- •What You Need
- •How Analog Video Works
- •Building a Digital-to-Analog Converter (DAC)
- •Connecting the Arduino to Your TV Set
- •Using the TVout Library
- •Building a TV Thermometer
- •Working with Graphics in TVout
- •What If It Doesn’t Work?
- •Exercises
- •9. Tinkering with the Wii Nunchuk
- •What You Need
- •Wiring a Wii Nunchuk
- •Talking to a Nunchuk
- •Building a Nunchuk Class
- •Using Our Nunchuk Class
- •Creating Your Own Video Game Console
- •Creating Your Own Video Game
- •What If It Doesn’t Work?
- •Exercises
- •10. Networking with Arduino
- •What You Need
- •Using Your PC to Transfer Sensor Data to the Internet
- •Registering an Application with Twitter
- •Tweeting Messages with Processing
- •Communicating Over Networks Using an Ethernet Shield
- •Using DHCP and DNS
- •What If It Doesn’t Work?
- •Exercises
- •11. Creating a Burglar Alarm with Email Notification
- •What You Need
- •Emailing from the Command Line
- •Emailing Directly from an Arduino
- •Detecting Motion Using a Passive Infrared Sensor
- •Bringing It All Together
- •What If It Doesn’t Work?
- •Exercises
- •What You Need
- •Understanding Infrared Remote Controls
- •Grabbing Remote Control Codes
- •Cloning a Remote
- •Controlling Infrared Devices Remotely with Your Browser
- •Building an Infrared Proxy
- •What If It Doesn’t Work?
- •Exercises
- •13. Controlling Motors with Arduino
- •What You Need
- •Introducing Motors
- •First Steps with a Servo Motor
- •Building a Blaminatr
- •What If It Doesn’t Work?
- •Exercises
- •Current, Voltage, and Resistance
- •Electrical Circuits
- •Learning How to Use a Wire Cutter
- •Learning How to Solder
- •Learning How to Desolder
- •The Arduino Programming Language
- •Bit Operations
- •Learning More About Serial Communication
- •Serial Communication Using Various Languages
- •What Are Google Chrome Apps?
- •Creating a Minimal Chrome App
- •Starting the Chrome App
- •Exploring the Chrome Serial API
- •Writing a SerialDevice Class
- •Index
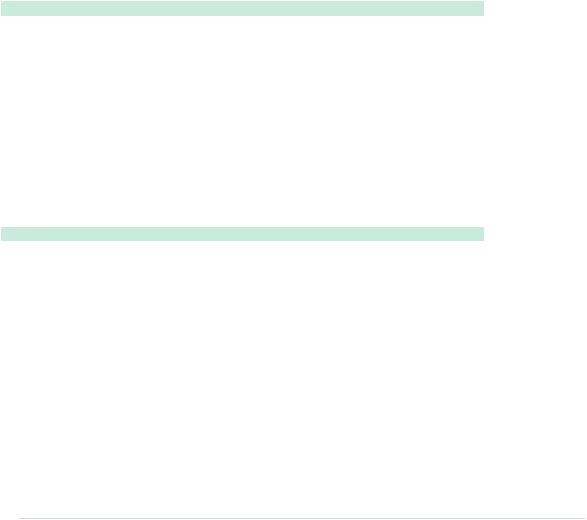
Working with Graphics in TVout • 139
Next, we output the current temperature as text. We use TVout’s select_font, set_cursor, and print methods to output the text “Current Temperature” in a font that is 6 pixels wide and 8 pixels high. After that, we output the current temperature in degrees Celsius using the 8x8 font. The TVout library doesn’t define a symbol for degrees Celsius, so we use the draw_circle method in line 21 to draw a small circle to simulate a degrees symbol.
We’re done! That’s all the code we need to make the TV thermometer work. The only thing I haven’t explained in detail is how outputting the thermometer image works. You’ll learn more about that in the next section.
Working with Graphics in TVout
In TvThermometer.ino we’ve included the thermometer.h file without explaining what
it contains. Here’s how it looks:
Video/TvThermometer/thermometer.h
#ifndef THERMOMETER_H #define THERMOMETER_H
extern const unsigned char thermometer[]; #endif
Quite disappointing, isn’t it? The file declares only a single variable named thermometer. This variable is an array of unsigned character values, and the extern keyword tells the compiler that we only want to declare the variable. That is, we can refer to it in our program, but we still have to define it to allocate some memory.
We actually define the thermometer variable in thermometer.cpp (we’ve skipped a few lines for brevity):
Video/TvThermometer/thermometer.cpp
Line 1 #include <Arduino.h>
-#include <avr/pgmspace.h>
-#include "thermometer.h"
-PROGMEM const unsigned char thermometer[] = { 5 20, 94,
-B00000000, B11110000, B00000000,
-B00000001, B00001000, B00000000,
-B00000010, B00000100, B00000000,
-B00000010, B00000100, B00000000,
10 B00000010, B00000100, B00000000,
-B00000010, B00000111, B10000000, // 40.0
-B00000010, B00000100, B00000000,
-B00000010, B00000100, B00000000,
-B00000010, B00000100, B00000000,
15 B00000010, B00000100, B00000000,
-B00000010, B00000100, B00000000,
report erratum • discuss

Chapter 8. Generating Video Signals with an Arduino • 140
-B00000010, B00000100, B00000000,
-B00000010, B00000100, B00000000,
-B00000010, B00000100, B00000000, 20 B00000010, B00000100, B00000000,
-// ...
-B00000010, B00000100, B00000000, // 5.5
-B00000111, B11111110, B00000000,
-B00001111, B11111111, B00000000,
25 B00011111, B11111111, B10000000,
-B00111111, B11111111, B11000000,
-B01111111, B11111111, B11100000,
-B01111111, B11111111, B11100000,
-B11111111, B11111111, B11110000, 30 B11111111, B11111111, B11110000,
-B11111111, B11111111, B11110000,
-B11111111, B11111111, B11110000,
-B11111111, B11111111, B11110000,
-B11111111, B11111111, B11110000, 35 B01111111, B11111111, B11100000,
-B01111111, B11111111, B11100000,
-B00111111, B11111111, B11000000,
-B00011111, B11111111, B10000000,
-B00001111, B11111111, B00000000, 40 B00000111, B11111110, B00000000,
-B00000001, B11111000, B00000000,
-B00000001, B11111000, B00000000,
-};
This file looks weird at first, but it’s really simple. First, we include Arduino.h because we’ll need to declare binary constants later. After that, we include avr/pgmspace.h because we want to store our image data in the Arduino’s flash RAM. Eventually, we include thermometer.h because we need the declaration of our thermometer image data.
In line 4, we eventually define the thermometer variable we declared in thermometer.h. The definition differs slightly from the declaration because it contains the PROGMEM directive.5 This directive tells the compiler to copy the data stored in the thermometer variable to the Arduino’s flash memory. Usually, when you define a variable in an Arduino program, it occupies memory in the SRAM. Most Arduinos don’t have a lot of SRAM (the Arduino Uno only has 2 KB), so it’s a valuable resource and you shouldn’t waste it. As a rule of thumb, you should store all constant data in the Arduino’s flash RAM. Use SRAM only for information that might change during program execution.
5.http://arduino.cc/en/Reference/PROGMEM
report erratum • discuss

Working with Graphics in TVout • 141
Image data like our thermometer usually doesn’t change, so you should always store it in flash RAM using the PROGMEM directive. TVout expects image data in raw format. The first two bytes contain the width and height of an image. The data that follows contains the image data line by line. In thermometer.cpp, each line of image data contains three bytes, because the image is 20 pixels wide, and 20 pixels occupy three bytes. Consequently, the file contains 94 lines each representing a single line of the thermometer image. Because we’ve used binary literals to encode the image data, you can actually see how the image looks when reading the source code. A 1 represents a white pixel, and a 0 represents a black pixel.
Drawing Images for Your Arduino Programs
You can draw simple images directly in the source code by editing binary numbers. As soon as your images get more complex, you need some tool support. For graphics that are still fairly simple but that are too complex to edit the binary numbers in the source code, you can use any drawing program, of course, but most modern tools are way too complicated for this job.
I’ve created the thermometer image with a fairly simple online tool named
Piskel.6 It’s open source, it’s easy to use, and it feels just right for creating Arduino graphics. You can see it in action in Figure 25, You can find good online editors for pixel graphics, on page 142.
Applications like Piskel really help to create images for your Arduino programs, but they usually store these images in .gif or .png files. In the next section, you’ll learn how to convert these files into source code.
Turning Pixels into C++ Code
After you’ve finished your pixel art, you still have to convert it into a C/C++ file. You could do it manually, but that wouldn’t be very pragmatic, would it? It’d be much better to write a small program that does the conversion automatically.
You could write such a program in any modern programming language; we’ll use Ruby here. Processing graphics in Ruby is easy thanks to the rmagick library. This library is a binding to ImageMagick,7 a powerful tool for transforming images. Before you can install rmagick, you have to install ImageMagick.
6.http://www.piskelapp.com/
7.http://www.imagemagick.org/
report erratum • discuss
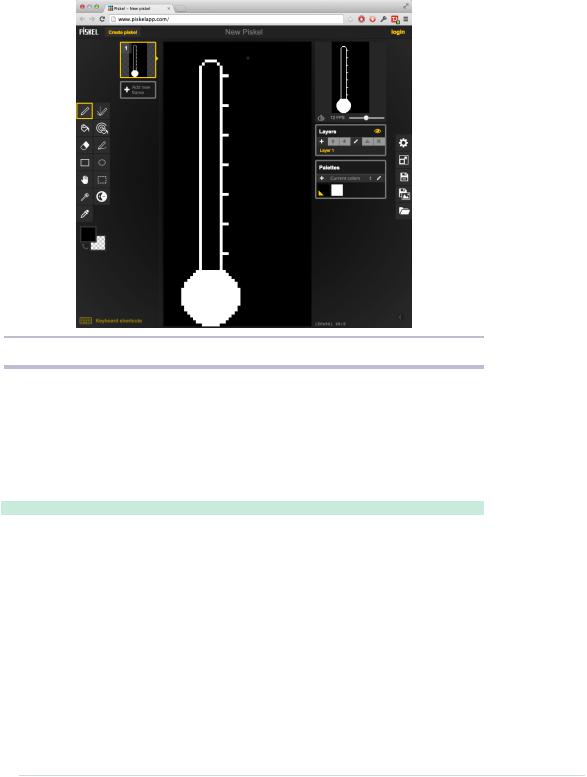
Chapter 8. Generating Video Signals with an Arduino • 142
Figure 25—You can find good online editors for pixel graphics.
When ImageMagick is available on your system, you can install the rmagick
|
library using the following command: |
|
maik> gem install rmagick |
|
Now you can use rmagick in your Ruby programs. We’ll use it to convert a |
|
graphics file into a C++ file: |
|
Video/img2cpp.rb |
Line 1 |
require 'RMagick' |
- |
include Magick |
- |
|
- |
image = Image::read(ARGV[0]).first |
5 |
|
-puts '#include "thermometer.h"'
-puts 'PROGMEM const unsigned char thermometer[] = {'
-puts " #{image.columns}, #{image.rows},"
-
10 (0..image.rows).each do |y| - print ' B'
-(0..image.columns).each do |x|
-pixel = image.pixel_color(x, y)
-print pixel.red == 0 ? '0' : '1' 15 print ', B' if (x + 1) % 8 == 0
report erratum • discuss