
- •Introduction
- •Introduction to Python and its use in science
- •Launching Python
- •Installing Python on your computer
- •The Canopy window
- •The Interactive Python Pane
- •Interactive Python as a calculator
- •Python Modules
- •Variables
- •Importing Modules
- •Getting help: documentation in IPython
- •Programming is a detail-oriented activity
- •Exercises
- •Strings, Lists, Arrays, and Dictionaries
- •Strings
- •Lists
- •NumPy arrays
- •Dictionaries
- •Random numbers
- •Exercises
- •Input and Output
- •Keyboard input
- •Screen output
- •File input
- •File output
- •Exercises
- •Plotting
- •An interactive session with pyplot
- •Basic plotting
- •Logarithmic plots
- •More advanced graphical output
- •Exercises
- •Conditionals and Loops
- •Conditionals
- •Loops
- •List Comprehensions
- •Exercises
- •Functions
- •Methods and attributes
- •Exercises
- •Curve Fitting
- •Exercises
- •Numerical Routines: SciPy and NumPy
- •Special functions
- •Linear algebra
- •Solving non-linear equations
- •Solving ODEs
- •Discrete (fast) Fourier transforms
- •Exercises
- •Installing Python
- •IPython Notebooks
- •Python Resources
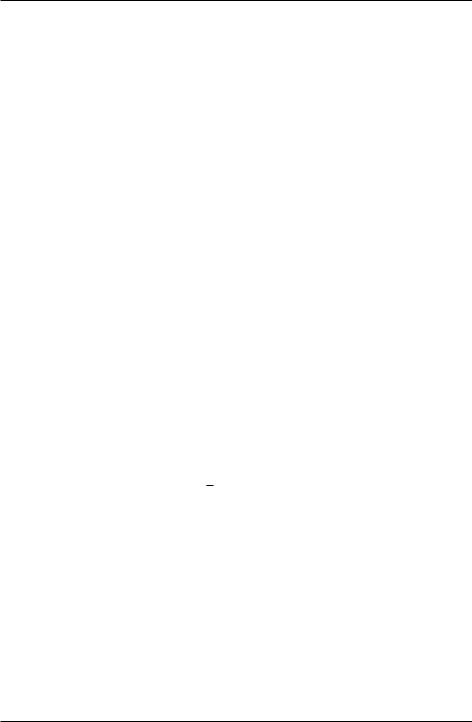
Introduction to Python for Science, Release 0.9.23
2.6.2 Keyword arguments
In addition to regular arguments, Python functions can have keyword arguments (kwargs). Keyword arguments are optional arguments that need not be specified when a function is called. See Basic plotting for examples of the use of keyword arguments. For the moment, we don’t need them so we defer a full discussion of keyword arguments until we introduce user defined functions.
2.7 Variables
2.7.1 Names and the assignment operator
A variable is a name that is used to store data. It can be used to store different kinds of data, but here we consider the simplest case where the data is a single numerical value. Here are a few examples:
In [1]: a = 23
In [2]: p, q = 83.4, sqrt(2)
The equal sign “=” is the assignment operator. In the first statement, it assigns the value of 23 to the variable a. In the second statement it assigns a value of 83.4 to p and a value of 1.4142135623730951 to q. To be more precise, the name of a variable, such as a, is associated with a memory location in your computer; the assignment variable tells the computer to put a particular piece of data, in this case a numerical value, in that memory location. Note that Python stores the numerical value, not the expression used to generate
it. Thus, q is assigned the 17-digit number 1.4142135623730951 generated by evaluating p
the expression sqrt(2), not with 2. (Actually the value of q is stored as a binary, base 2, number using scientific notation with a mantissa and an exponent.)
Suppose we write
In [3]: b = a
In this case Python associates a new memory location with the name b, distinct from the one associated with a, and sets the value stored at that memory location to 23, the value of a. The following sequence of statements demonstrate that fact. Can you see how? Notice that simply typing a variable name and pressing Return prints out the value of the variable.
In [4]: a=23
2.7. Variables |
17 |
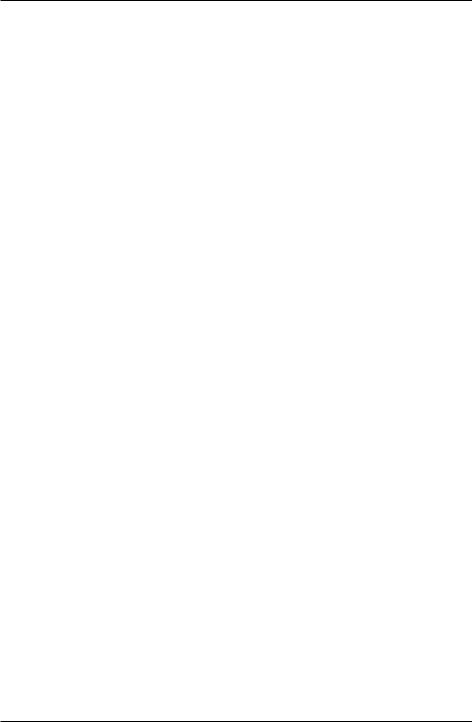
Introduction to Python for Science, Release 0.9.23
In [5]: b=a
In [6]: a
Out[6]: 23
In [7]: b
Out[7]: 23
In [8]: a=12
In [9]: a
Out[9]: 12
In [10]: b
Out[10]: 23
The assignment variable works from right to left; that is, it assigns the value of the number on the right to the variable name on the left. Therefore, the statement “5=a” makes no sense in Python. The assignment operator “=” in Python is not equivalent to the equals sign “=” we are accustomed to in algebra.
The assignment operator can be used to increment or change the value of a variable
In [11]: b = b+1
In [12]: b
Out[12]: 24
The statement, b = b+1 makes no sense in algebra, but in Python (and most computer languages), it makes perfect sense: it means “add 1 to the current value of b and assign the result to b.” This construction appears so often in computer programming that there is a special set of operators to perform such changes to a variable: +=, -=, *=, and /=. Here are some examples of how they work:
In [13]: c , d = 4, 7.92
In [14]: c += 2
In [15]: c
Out[15]: 6
In [16]: c *= 3
In [16]: c
Out[16]: 18
18 |
Chapter 2. Launching Python |
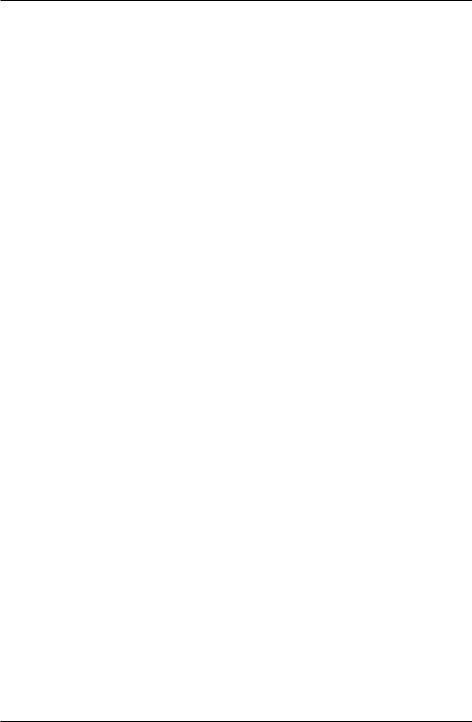
Introduction to Python for Science, Release 0.9.23
In [17]: d /= -2
In [17]: d
Out[17]: -3.96
In [18]: d -= 4
In [19]: d
Out[19]: -7.96
Verify that you understand how the above operations work.
2.7.2 Legal and recommended variable names
Variable names in Python must start with a letter, and can be followed by as many alphanumeric characters as you like. Spaces are not allowed in variable names. However, the underscore character “_” is allowed, but no other character that is not a letter or a number is permitted.
Recall that Python is case sensitive, so the variable a is distinct from the variable A.
We recommend giving your variables descriptive names as in the following calculation:
In [20]: distance = 34.
In [21]: time_traveled = 0.59
In [22]: velocity = distance/time_traveled
In [23]: velocity
Out[23]: 57.6271186440678
The variable names distance, time_traveled, and velocity immediately remind you of what is being calculated here. This is good practice. But so is keeping variable names reasonably short, so don’t go nuts!
2.7.3 Reserved words in Python
There are also some names or words that are reserved by Python for special purposes or functions. You must avoid using these names, which are provided here for your reference:
2.7. Variables |
19 |
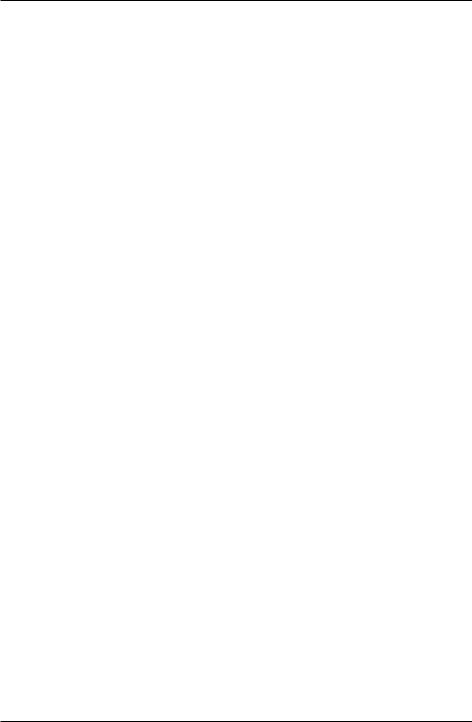
Introduction to Python for Science, Release 0.9.23
and |
del |
from |
not |
while |
|
|
|
|
|
as |
elif |
global |
or |
with |
assert |
else |
if |
pass |
yield |
|
|
|
|
|
break |
except |
import |
|
|
|
|
|
|
|
class |
exec |
in |
raise |
|
|
|
|
|
|
continue |
finally |
is |
return |
|
|
|
|
|
|
def |
for |
lambda |
try |
|
|
|
|
|
|
In addition, you should not use function names, like sin, cos, and sqrt, defined in the SciPy, NumPy, or any other library that you are using.
2.8 Script files and programs
Performing calculations in the IPython shell is handy if the calculations are short. But calculations quickly become tedious when they are more than a few lines long. If you discover you made a mistake at some early step, for example, you may have to go back and retype all the steps subsequent to the error. Having code saved in a file means you can just correct the error and rerun the code without having to retype it. Saving code can also be useful if you want to reuse it later, perhaps with different inputs.
For these and many other reasons, we save code in computer files. We call the sequence of commands stored in a file a script or a program or sometimes a routine. Programs can become quite sophisticated and complex. Here we are only going to introduce the simplest features of programming by writing a very simple script. Much later, we will introduce some of the more advanced features of programming.
To write a script you need a text editor. In principle, any text editor will do, but it’s more convenient to use an editor that was designed for the task. We are going to use the Code Editor in the Canopy window that appears when you launch the Canopy application (see Canopy window). This editor, like most good programming editors, provides syntax highlighting, which color codes key words, comments, and other features of the Python syntax according to their function, and thus makes it easier to read the code and easier to spot programming mistakes. The Canopy code editor also provides syntax checking, much like a spell-checker in a word processing program, that identifies many coding errors. This can greatly speed the coding process. Tab completion also works in the Canopy Code Editor.
20 |
Chapter 2. Launching Python |
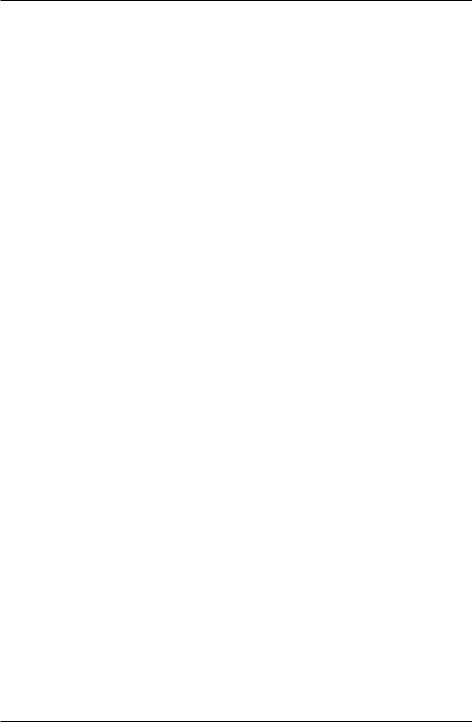
Introduction to Python for Science, Release 0.9.23
2.8.1 Scripting Example 1
Let’s work through an example to see how scripting works. Suppose you are going on a road trip and you would like to estimate how long the drive will take, how much gas you will need, and the cost of the gas. It’s a simple calculation. As inputs, you will need the distance of the trip, your average speed, the cost of gasoline, and the mileage of your car.
Writing a script to do these calculations is straightforward. First, launch Canopy and open the code editor. You should see a tab with the word untitled at the top left of the code editor pane (see Canopy window). If you don’t, go to the File menu and select New File. Use the mouse to place your cursor at the top of the code editor pane. Enter the following code and save the code in a file called myTrip.py in the PyProgs folder you created earlier. This stores your script (or program) on your computer’s disk. The exact name of the file is not important but the extension .py is essential. It tells the computer, and more importantly Python, that this is a Python program.
# Calculates time, gallons of gas |
used, and cost of gasoline for a trip |
|||
distance = 400. |
# miles |
|
||
mpg = |
30. |
# car mileage |
||
speed |
= 60. |
# |
average |
speed |
costPerGallon = 4.10 |
# |
price of gas |
time = distance/speed gallons = distance/mpg
cost = gallons*costPerGallon
The number (or hash) symbol # is the “comment” character in Python; anything on a line following # is ignored when the code is executed. Judicious use of comments in your code will make your code much easier to understand days, weeks, or months after the time you wrote it. Use comments generously.
Now you are ready to run the code. Before doing so, you first need to use the IPython console to move to the PyProgs directory where the file containing the code resides. From the IPython console, use the cd command to move to the PyProgs directory. For example, you might type
In [1]: cd ~/Documents/PyProgs/
To run or execute a script, simply type run filename, which in this case means type run myTrip.py. When you run a script, Python simply executes the sequence of commands in the order they appear.
In [2]: run myTrip.py
Once you have run the script, you can see the values of the variables calculated in the
2.8. Script files and programs |
21 |
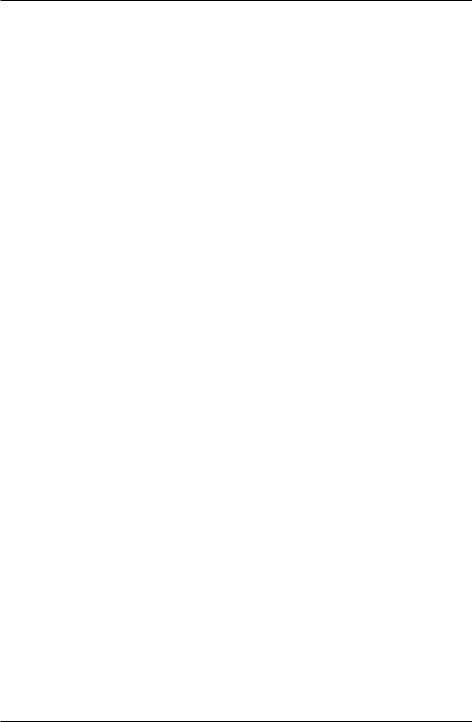
Introduction to Python for Science, Release 0.9.23
script simply by typing the name of the variable. IPython responds with the value of that variable.
In [3]: |
time |
Out[3]: |
6.666666666666667 |
In [4]: |
gallons |
Out[4]: |
13.333333333333334 |
In [5]: |
cost |
Out[5]: |
54.666666666666664 |
You can change the number of digits IPython displays using the command %precision:
In [6]: %precision 2
Out[6]: u’%.2f’
In [7]: time
Out[7]: 6.67
In [8]: gallons
Out[8]: 13.33
In [9]: cost
Out[9]: 54.67
Typing %precision returns IPython to its default state; %precision %e causes IPython to display numbers in exponential format (scientific notation).
Note about printing
If you want your script to return the value of a variable (that is, print the value of the variable to your computer screen), use the print function. For example, at the end of our script, if we include the code
print(time) print(gallons) print(cost)
the script will return the values of the variables time, gallons, and cost that the script calculated. We will discuss the print function in much greater detail, as well as other methods for data output, in Chapter 4 on Input and Output.
22 |
Chapter 2. Launching Python |
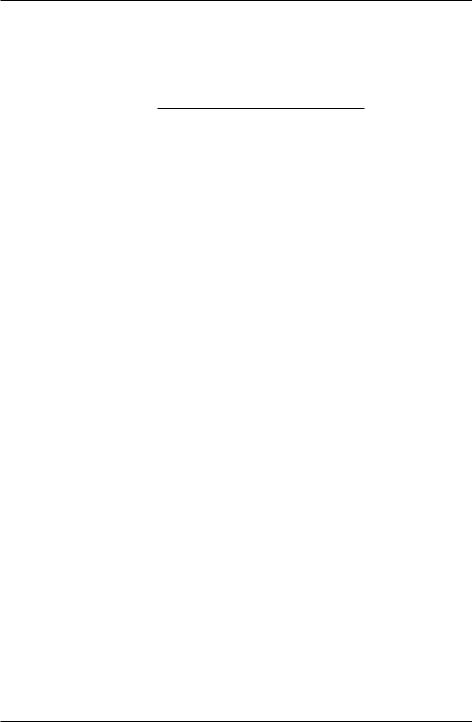
Introduction to Python for Science, Release 0.9.23
2.8.2 Scripting Example 2
Let’s try another problem. Suppose you want to find the distance between two Cartesian coordinates (x1; y1; z1) and (x2; y2; z2). The distance, of course, is given by the formula
p
r = (x2 x1)2 + (y2 y1)2 + (z2 z1)2
Now let’s write a script to do this calculation and save it in a file called twoPointDistance.py.
# Calculates the distance between two 3d Cartesian coordinates import numpy as np
x1, y1, z1 = 23.7, -9.2, -7.8 x2, y2, z2 = -3.5, 4.8, 8.1
dr = np.sqrt( (x2-x1)**2 + (y2-y1)**2 + (z2-z1)**2 )
We have introduced extra spaces into some of the expressions to improve readability. They are not necessary; where and whether you include them is largely a matter of taste.
There are two important differences between the code above and the commands we would have written into the IPython console to execute the same set of commands. The first is the statement on the second line
...
import numpy as np
...
and the second is the “np.” in front of the sqrt function on the last line. If you leave out the import numpy as np line and remove the np. in front of the sqrt function, you will get the following error message
----> 7 dr = sqrt( (x2-x1)**2 + (y2-y1)**2 + (z2-z1)**2 )
NameError: name ’sqrt’ is not defined
The reason for the error is that the sqrt function is not a part of core Python. But it is a part of the NumPy module discussed earlier. To make the NumPy library available to the script, you need to add the statement import numpy as np. Then, when you call a NumPy function, you need to write the function with the np. prefix. Failure to do either will result in a error message. Now we can run the script.
In [10]: run twoPointDistance.py
In [11]: dr
Out[11]: 34.48
2.8. Script files and programs |
23 |