
- •Introduction
- •Introduction to Python and its use in science
- •Launching Python
- •Installing Python on your computer
- •The Canopy window
- •The Interactive Python Pane
- •Interactive Python as a calculator
- •Python Modules
- •Variables
- •Importing Modules
- •Getting help: documentation in IPython
- •Programming is a detail-oriented activity
- •Exercises
- •Strings, Lists, Arrays, and Dictionaries
- •Strings
- •Lists
- •NumPy arrays
- •Dictionaries
- •Random numbers
- •Exercises
- •Input and Output
- •Keyboard input
- •Screen output
- •File input
- •File output
- •Exercises
- •Plotting
- •An interactive session with pyplot
- •Basic plotting
- •Logarithmic plots
- •More advanced graphical output
- •Exercises
- •Conditionals and Loops
- •Conditionals
- •Loops
- •List Comprehensions
- •Exercises
- •Functions
- •Methods and attributes
- •Exercises
- •Curve Fitting
- •Exercises
- •Numerical Routines: SciPy and NumPy
- •Special functions
- •Linear algebra
- •Solving non-linear equations
- •Solving ODEs
- •Discrete (fast) Fourier transforms
- •Exercises
- •Installing Python
- •IPython Notebooks
- •Python Resources

Introduction to Python for Science, Release 0.9.23
5,2.5,148,3
6,3,136,2.5
7,3.5,120,3
8,4,99,4
9,4.5,83,2.5
10,5,55,3.6
11,5.5,35,1.75
12,6,5,0.75
As its name suggests, the CSV file is simply a text file with the data that was formerly in spreadsheet columns now separated by commas. We can read the data in this file into a Python program using the loadtxt NumPy function once again. Here is the code
In [3]: dataPt, time, height, error = loadtxt("MyData.csv", skiprows=5 , unpack=True, delimiter=’,’)
The form of the function is exactly the same as before except we have added the argument delimiter=’,’ that tells loadtxt that the columns are separated by commas instead of white space (spaces or tabs), which is the default. Once again, we set the skiprows argument to skip the header at the beginning of the file and to start reading at the first row of data. The data are output to the arrays to the right of the assignment operator = exactly as in the previous example.
4.4 File output
4.4.1 Writing data to a text file
There is a plethora of ways to write data to a data file in Python. We will stick to one very simple one that’s suitable for writing data files in text format. It uses the NumPy savetxt routine, which is the counterpart of the loadtxt routine introduced in the previous section. The general form of the routine is
savetxt(filename, array, fmt="%0.18e", delimiter=" ", newline="\n", header="", footer="", comments="# ")
We illustrate savetext below with a script that first creates four arrays by reading in the data file MyData.txt, as discussed in the previous section, and then writes that same data set to another file MyDataOut.txt.
1import numpy as np
2
3dataPt, time, height, error = np.loadtxt("MyData.txt",
66 |
Chapter 4. Input and Output |
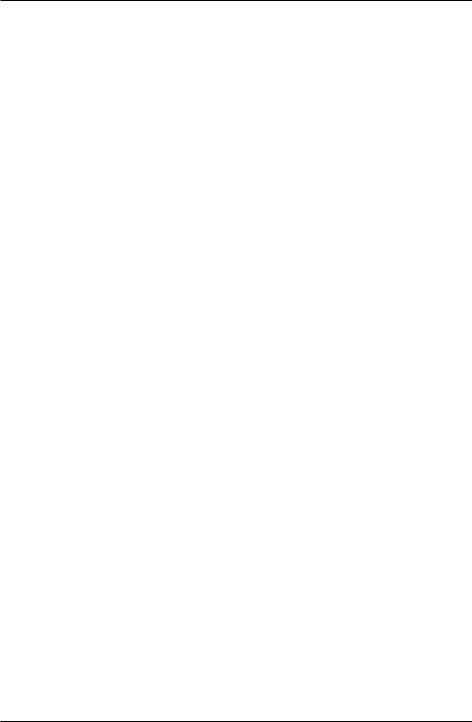
Introduction to Python for Science, Release 0.9.23
4 |
skiprows=5, unpack=True) |
5 |
|
6 |
np.savetxt(’MyDataOut.txt’, |
7 |
zip(dataPt, time, height, error), fmt="%12.1f") |
The first argument of of savetxt is a string, the name of the data file to be created. Here we have chosen the name MyDataOut.txt, inserted with quotes, which designates it as a string literal. Beware, if there is already a file of that name on your computer, it will be overwritten—the old file will be destroyed and a new one will be created.
The second argument is the data array the is to be written to the data file. Because we want to write not one but four data arrays to the file, we have to package the four data arrays as one, which we do using the zip function, a Python function that combines returns a list of tuples, where the ith tuple contains the ith element from each of the arrays (or lists, or tuples) listed as its arguments. Since there are four arrays, each row will be a tuple with four entries, producing a table with four columns. Note that the first two arguments, the filename and data array, are regular arguments and thus must appear as the first and second arguments in the correct order. The remaining arguments are all keyword arguments, meaning that they are optional and can appear in any order, provided you use the keyword.
The next argument is a format string that determines how the elements of the array are displayed in the data file. The argument is optional and, if left out, is the format 0.18e, which displays numbers as 18 digit floats in exponential (scientific) notation. Here we choose a different format, 12.1f, which is a float displayed with 1 digit to the right of the decimal point and a minimum width of 12. By choosing 12, which is more digits than any of the numbers in the various arrays have, we ensure that all the columns will have the same width. It also ensures that the decimal points in column of numbers are aligned. This is evident in the data file below, MyDataOut.txt, which was produced by the above script.
0.0 |
0.0 |
180.0 |
3.5 |
1.0 |
0.5 |
182.0 |
4.5 |
2.0 |
1.0 |
178.0 |
4.0 |
3.0 |
1.5 |
165.0 |
5.5 |
4.0 |
2.0 |
160.0 |
2.5 |
5.0 |
2.5 |
148.0 |
3.0 |
6.0 |
3.0 |
136.0 |
2.5 |
7.0 |
3.5 |
120.0 |
3.0 |
8.0 |
4.0 |
99.0 |
4.0 |
9.0 |
4.5 |
83.0 |
2.5 |
10.0 |
5.0 |
55.0 |
3.6 |
11.0 |
5.5 |
35.0 |
1.8 |
12.0 |
6.0 |
5.0 |
0.8 |
4.4. File output |
67 |

Introduction to Python for Science, Release 0.9.23
We omitted the optional delimiter keyword argument, which leaves the delimiter as the default space.
We also omitted the optional header keyword argument, which is a string variable that allows you to write header text above the data. For example, you might want to label the data columns and also include the information that was in the header of the original data file. To do so, you just need to create a string with the information you want to include and then use the header keyword argument. The code below illustrates how to do this.
1import numpy as np
2
3dataPt, time, height, error = np.loadtxt("MyData.txt",
4
5
skiprows=5, unpack=True)
6 info = ’Data for falling mass experiment’
7info += ’\nDate: 16-Aug-2013’
8info += ’\nData taken by Lauren and John’
9 |
info |
+= |
’\n\n data |
point |
time (sec) height (mm) ’ |
10 |
info |
+= |
’uncertainty |
(mm)’ |
|
11
12np.savetxt(’MyDataOut.txt’,
13zip(dataPt, time, height, error), header=info, fmt="%12.1f")
Now the data file produces has a header preceding the data. Notice that the header rows all start with a # comment character, which is the default setting for the savetxt function. This can be changed using the keyword argument comments. You can find more information about savetxt using the IPython help function or from the online NumPy documentation.
#Data for falling mass experiment
#Date: 16-Aug-2013
#Data taken by Lauren and John
# |
|
|
|
|
# |
data point |
time (sec) height (mm) |
uncertainty (mm) |
|
|
0.0 |
0.0 |
180.0 |
3.5 |
|
1.0 |
0.5 |
182.0 |
4.5 |
|
2.0 |
1.0 |
178.0 |
4.0 |
|
3.0 |
1.5 |
165.0 |
5.5 |
|
4.0 |
2.0 |
160.0 |
2.5 |
|
5.0 |
2.5 |
148.0 |
3.0 |
|
6.0 |
3.0 |
136.0 |
2.5 |
|
7.0 |
3.5 |
120.0 |
3.0 |
|
8.0 |
4.0 |
99.0 |
4.0 |
|
9.0 |
4.5 |
83.0 |
2.5 |
|
10.0 |
5.0 |
55.0 |
3.6 |
68 |
Chapter 4. Input and Output |
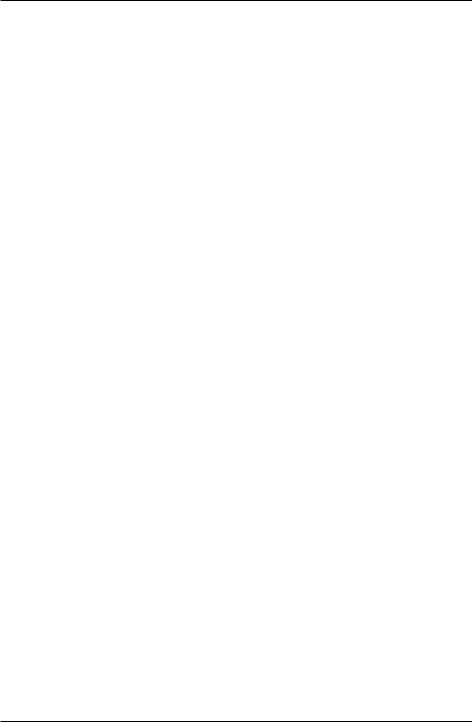
Introduction to Python for Science, Release 0.9.23
11.0 |
5.5 |
35.0 |
1.8 |
12.0 |
6.0 |
5.0 |
0.8 |
4.4.2 Writing data to a CSV file
To produce a CSV file, you would specify a comma as the delimiter. You might use the 0.1f format specifier, which leaves no extra spaces between the comma data separators, as the file is to be read by a spreadsheet program, which will determine how the numbers are displayed. The code, which could be substituted for the savetxt line in the above code reads
np.savetxt(’MyDataOut.csv’,
zip(dataPt, time, height, error), fmt="%0.1f", delimiter=",")
and produces the following data file
0.0,0.0,180.0,3.5
1.0,0.5,182.0,4.5
2.0,1.0,178.0,4.0
3.0,1.5,165.0,5.5
4.0,2.0,160.0,2.5
5.0,2.5,148.0,3.0
6.0,3.0,136.0,2.5
7.0,3.5,120.0,3.0
8.0,4.0,99.0,4.0
9.0,4.5,83.0,2.5
10.0,5.0,55.0,3.6
11.0,5.5,35.0,1.8
12.0,6.0,5.0,0.8
This data file, with a csv extension, can be directly read into a spreadsheet program like Excel.
4.4. File output |
69 |