
- •Introduction
- •Introduction to Python and its use in science
- •Launching Python
- •Installing Python on your computer
- •The Canopy window
- •The Interactive Python Pane
- •Interactive Python as a calculator
- •Python Modules
- •Variables
- •Importing Modules
- •Getting help: documentation in IPython
- •Programming is a detail-oriented activity
- •Exercises
- •Strings, Lists, Arrays, and Dictionaries
- •Strings
- •Lists
- •NumPy arrays
- •Dictionaries
- •Random numbers
- •Exercises
- •Input and Output
- •Keyboard input
- •Screen output
- •File input
- •File output
- •Exercises
- •Plotting
- •An interactive session with pyplot
- •Basic plotting
- •Logarithmic plots
- •More advanced graphical output
- •Exercises
- •Conditionals and Loops
- •Conditionals
- •Loops
- •List Comprehensions
- •Exercises
- •Functions
- •Methods and attributes
- •Exercises
- •Curve Fitting
- •Exercises
- •Numerical Routines: SciPy and NumPy
- •Special functions
- •Linear algebra
- •Solving non-linear equations
- •Solving ODEs
- •Discrete (fast) Fourier transforms
- •Exercises
- •Installing Python
- •IPython Notebooks
- •Python Resources

Introduction to Python for Science, Release 0.9.23
import numpy as np
a = np.linspace(0, 32, 1e7) print(a)
a = a*a print(a)
Running this on my computer returns the results faster than I can discern, but certainly much less than a second. This illustrates an important point: for loops are slow. Array operations run much faster and are therefore to be preferred in any case where you have a choice. Sometimes finding an array operation that is equivalent to a loop can be difficult, especially for a novice. Nevertheless, doing so pays rich rewards in execution time. Moreover, the array notation is usually simpler and clearer, providing further reasons to prefer array operations over loops.
6.3 List Comprehensions
List comprehensions are a special feature of core Python for processing and constructing lists. We introduce them here because they use a looping process. They are used quite commonly in Python coding and they often provide elegant compact solutions to some common computing tasks.
Consider, for example the 3 3 matrix
In [1]: x = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
Suppose we want to construct a vector from the diagonal elements of this matrix. We could do so with a for loop with an accumulator as follows
In [2]: diag = []
...: for i in [0, 1, 2]:
...: diag.append(x[i][i])
...:
In [3]: diag
Out[3]: [1, 5, 9]
List comprehensions provide a simpler, cleaner, and faster way of doing the same thing
In [4]: diagLC = [x[i][i] for i in [0, 1, 2]]
In [5]: diagLC
Out[5]: [1, 5, 9]
110 |
Chapter 6. Conditionals and Loops |

Introduction to Python for Science, Release 0.9.23
A one-line list comprehension replaces a three-line accumulator plus loop code.
Suppose we now want the square of this list:
In [6]: [y*y for y in diagLC]
Out[6]: [1, 25, 81]
Notice here how y serves as a dummy variable accessing the various elements of the list diagLC.
Extracting a column from a 2-dimensaional array such as x is quite easy. For example the second row is obtained quite simply in the following fashion
In [7]: x[1]
Out[7]: [4, 5, 6]
Obtaining a column is not as simple, but a list comprehension makes it quite straightforward:
In [7]: c1 = [a[1] for a in x]
In [8]: c1
Out[8]: [2, 5, 8]
Another, slightly less elegant way to accomplish the same thing is
In [9]: [x[i][1] for i in range(3)]
Out[9]: [2, 5, 8]
Suppose you have a list of numbers and you want to extract all the elements of the list that are divisible by three. A slightly fancier list comprehension accomplishes the task quite simply and demonstrates a new feature:
In [10]: y = [-5, -3, 1, 7, 4, 23, 27, -9, 11, 41]
In [14]: [a for a in y if a%3==0] Out[14]: [-3, 27, -9]
As we see in this example, a conditional statement can be added to a list comprehension. Here it serves as a filter to select out only those elements that are divisible by three.
6.3. List Comprehensions |
111 |
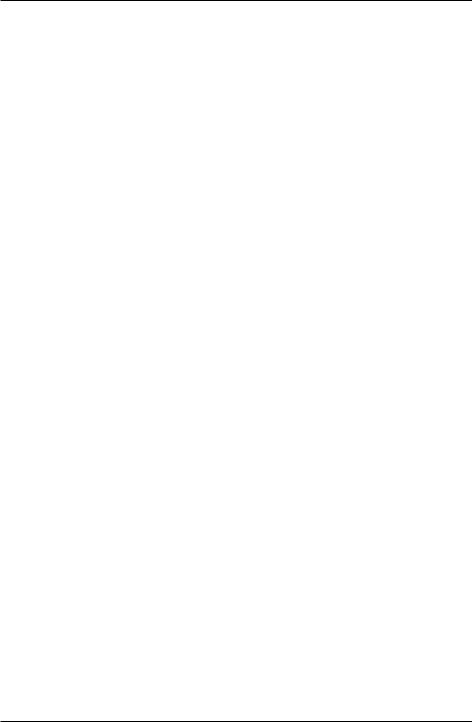
Introduction to Python for Science, Release 0.9.23
6.4 Exercises
1.Write a program to calculate the factorial of a positive integer input by the user. Recall that the factorial function is given by x! = x(x 1)(x 2):::(2)(1) so that
1! = 1, 2! = 2, 3! = 6, 4! = 24, ...
(a)Write the factorial function using a Python while loop.
(b)Write the factorial function using a Python for loop.
Check your programs to make sure they work for 1, 2, 3, 5, and beyond, but especially for the first 5 integers.
2.The following Python program finds the smallest non-trivial (not 1) prime factor of a positive integer.
n = int(raw_input("Input an integer > 1: ")) i = 2
while (n % i) != 0: i += 1
print("The smallest factor of n is:", i )
(a)Type this program into your computer and verify that it works as advertised. Then briefly explain how it works and why the while loop always terminates.
(b)Modify the program so that it tells you if the integer input is a prime number or not. If it is not a prime number, write your program so that it prints out the smallest prime factor. Using your program verify that the following integers are prime numbers: 101, 8191, 947431.
3.Consider the matrix list x = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]. Write a list comprehension to extract the last column of the matrix [3, 6, 9]. Write another list comprehension to create a vector of twice the square of the middle column [8, 50, 128].
4.Write a program that calculates the value of an investment after some number of years specified by the user if
(a)the principal is compounded annually
(b)the principle is compounded monthly
(c)the principle is compounded daily
112 |
Chapter 6. Conditionals and Loops |

Introduction to Python for Science, Release 0.9.23
Your program should ask the user for the initial investment (principal), the interest rate in percent, and the number of years the money will be invested (allow for fractional years). For an initial investment of $1000 at an interest rate of 6%, after 10 years I get $1790.85 when compounded annually, $1819.40 when compounded monthly, and $1822.03 when compounded daily, assuming 12 months in a year and 365.24 days in a year, where the monthly interest rate is the annual rate divided by 12 and the daily rate is the annual rate divided by 365 (don’t worry about leap years).
5.Write a program that determines the day of the week for any given calendar date after January 1, 1900, which was a Monday. Your program will need to take into account leap years, which occur in every year that is divisible by 4, except for years that are divisible by 100 but are not divisible by 400. For example, 1900 was not a leap year, but 2000 was a leap year. Test that your program gives the following answers: Monday 1900 January 1, Tuesday 1933 December 5, Wednesday 1993 June 23, Thursday 1953 January 15, Friday 1963 November 22, Saturday 1919 June 28, Sunday 2005 August 28.
6.4. Exercises |
113 |
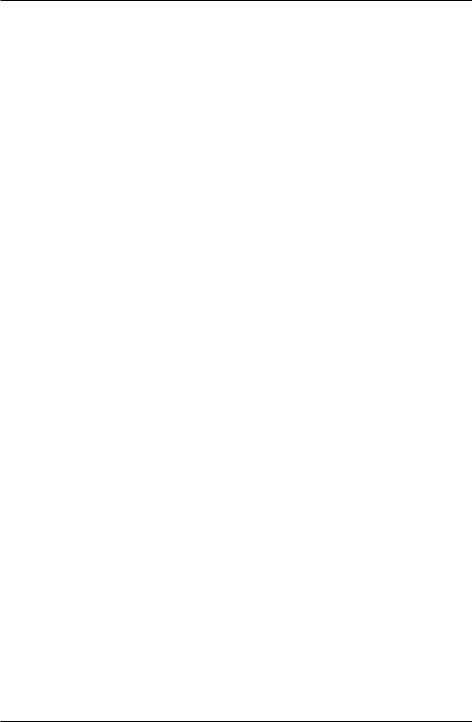
Introduction to Python for Science, Release 0.9.23
114 |
Chapter 6. Conditionals and Loops |