
- •CONTENTS
- •1.1 Introduction
- •1.2 What Is a Computer?
- •1.3 Programs
- •1.4 Operating Systems
- •1.5 Java, World Wide Web, and Beyond
- •1.6 The Java Language Specification, API, JDK, and IDE
- •1.7 A Simple Java Program
- •1.8 Creating, Compiling, and Executing a Java Program
- •1.9 (GUI) Displaying Text in a Message Dialog Box
- •2.1 Introduction
- •2.2 Writing Simple Programs
- •2.3 Reading Input from the Console
- •2.4 Identifiers
- •2.5 Variables
- •2.7 Named Constants
- •2.8 Numeric Data Types and Operations
- •2.9 Problem: Displaying the Current Time
- •2.10 Shorthand Operators
- •2.11 Numeric Type Conversions
- •2.12 Problem: Computing Loan Payments
- •2.13 Character Data Type and Operations
- •2.14 Problem: Counting Monetary Units
- •2.15 The String Type
- •2.16 Programming Style and Documentation
- •2.17 Programming Errors
- •2.18 (GUI) Getting Input from Input Dialogs
- •3.1 Introduction
- •3.2 boolean Data Type
- •3.3 Problem: A Simple Math Learning Tool
- •3.4 if Statements
- •3.5 Problem: Guessing Birthdays
- •3.6 Two-Way if Statements
- •3.7 Nested if Statements
- •3.8 Common Errors in Selection Statements
- •3.9 Problem: An Improved Math Learning Tool
- •3.10 Problem: Computing Body Mass Index
- •3.11 Problem: Computing Taxes
- •3.12 Logical Operators
- •3.13 Problem: Determining Leap Year
- •3.14 Problem: Lottery
- •3.15 switch Statements
- •3.16 Conditional Expressions
- •3.17 Formatting Console Output
- •3.18 Operator Precedence and Associativity
- •3.19 (GUI) Confirmation Dialogs
- •4.1 Introduction
- •4.2 The while Loop
- •4.3 The do-while Loop
- •4.4 The for Loop
- •4.5 Which Loop to Use?
- •4.6 Nested Loops
- •4.7 Minimizing Numeric Errors
- •4.8 Case Studies
- •4.9 Keywords break and continue
- •4.10 (GUI) Controlling a Loop with a Confirmation Dialog
- •5.1 Introduction
- •5.2 Defining a Method
- •5.3 Calling a Method
- •5.4 void Method Example
- •5.5 Passing Parameters by Values
- •5.6 Modularizing Code
- •5.7 Problem: Converting Decimals to Hexadecimals
- •5.8 Overloading Methods
- •5.9 The Scope of Variables
- •5.10 The Math Class
- •5.11 Case Study: Generating Random Characters
- •5.12 Method Abstraction and Stepwise Refinement
- •6.1 Introduction
- •6.2 Array Basics
- •6.3 Problem: Lotto Numbers
- •6.4 Problem: Deck of Cards
- •6.5 Copying Arrays
- •6.6 Passing Arrays to Methods
- •6.7 Returning an Array from a Method
- •6.8 Variable-Length Argument Lists
- •6.9 Searching Arrays
- •6.10 Sorting Arrays
- •6.11 The Arrays Class
- •7.1 Introduction
- •7.2 Two-Dimensional Array Basics
- •7.3 Processing Two-Dimensional Arrays
- •7.4 Passing Two-Dimensional Arrays to Methods
- •7.5 Problem: Grading a Multiple-Choice Test
- •7.6 Problem: Finding a Closest Pair
- •7.7 Problem: Sudoku
- •7.8 Multidimensional Arrays
- •8.1 Introduction
- •8.2 Defining Classes for Objects
- •8.3 Example: Defining Classes and Creating Objects
- •8.4 Constructing Objects Using Constructors
- •8.5 Accessing Objects via Reference Variables
- •8.6 Using Classes from the Java Library
- •8.7 Static Variables, Constants, and Methods
- •8.8 Visibility Modifiers
- •8.9 Data Field Encapsulation
- •8.10 Passing Objects to Methods
- •8.11 Array of Objects
- •9.1 Introduction
- •9.2 The String Class
- •9.3 The Character Class
- •9.4 The StringBuilder/StringBuffer Class
- •9.5 Command-Line Arguments
- •9.6 The File Class
- •9.7 File Input and Output
- •9.8 (GUI) File Dialogs
- •10.1 Introduction
- •10.2 Immutable Objects and Classes
- •10.3 The Scope of Variables
- •10.4 The this Reference
- •10.5 Class Abstraction and Encapsulation
- •10.6 Object-Oriented Thinking
- •10.7 Object Composition
- •10.8 Designing the Course Class
- •10.9 Designing a Class for Stacks
- •10.10 Designing the GuessDate Class
- •10.11 Class Design Guidelines
- •11.1 Introduction
- •11.2 Superclasses and Subclasses
- •11.3 Using the super Keyword
- •11.4 Overriding Methods
- •11.5 Overriding vs. Overloading
- •11.6 The Object Class and Its toString() Method
- •11.7 Polymorphism
- •11.8 Dynamic Binding
- •11.9 Casting Objects and the instanceof Operator
- •11.11 The ArrayList Class
- •11.12 A Custom Stack Class
- •11.13 The protected Data and Methods
- •11.14 Preventing Extending and Overriding
- •12.1 Introduction
- •12.2 Swing vs. AWT
- •12.3 The Java GUI API
- •12.4 Frames
- •12.5 Layout Managers
- •12.6 Using Panels as Subcontainers
- •12.7 The Color Class
- •12.8 The Font Class
- •12.9 Common Features of Swing GUI Components
- •12.10 Image Icons
- •13.1 Introduction
- •13.2 Exception-Handling Overview
- •13.3 Exception-Handling Advantages
- •13.4 Exception Types
- •13.5 More on Exception Handling
- •13.6 The finally Clause
- •13.7 When to Use Exceptions
- •13.8 Rethrowing Exceptions
- •13.9 Chained Exceptions
- •13.10 Creating Custom Exception Classes
- •14.1 Introduction
- •14.2 Abstract Classes
- •14.3 Example: Calendar and GregorianCalendar
- •14.4 Interfaces
- •14.5 Example: The Comparable Interface
- •14.6 Example: The ActionListener Interface
- •14.7 Example: The Cloneable Interface
- •14.8 Interfaces vs. Abstract Classes
- •14.9 Processing Primitive Data Type Values as Objects
- •14.10 Sorting an Array of Objects
- •14.11 Automatic Conversion between Primitive Types and Wrapper Class Types
- •14.12 The BigInteger and BigDecimal Classes
- •14.13 Case Study: The Rational Class
- •15.1 Introduction
- •15.2 Graphical Coordinate Systems
- •15.3 The Graphics Class
- •15.4 Drawing Strings, Lines, Rectangles, and Ovals
- •15.5 Case Study: The FigurePanel Class
- •15.6 Drawing Arcs
- •15.7 Drawing Polygons and Polylines
- •15.8 Centering a String Using the FontMetrics Class
- •15.9 Case Study: The MessagePanel Class
- •15.10 Case Study: The StillClock Class
- •15.11 Displaying Images
- •15.12 Case Study: The ImageViewer Class
- •16.1 Introduction
- •16.2 Event and Event Source
- •16.3 Listeners, Registrations, and Handling Events
- •16.4 Inner Classes
- •16.5 Anonymous Class Listeners
- •16.6 Alternative Ways of Defining Listener Classes
- •16.7 Problem: Loan Calculator
- •16.8 Window Events
- •16.9 Listener Interface Adapters
- •16.10 Mouse Events
- •16.11 Key Events
- •16.12 Animation Using the Timer Class
- •17.1 Introduction
- •17.2 Buttons
- •17.3 Check Boxes
- •17.4 Radio Buttons
- •17.5 Labels
- •17.6 Text Fields
- •17.7 Text Areas
- •17.8 Combo Boxes
- •17.9 Lists
- •17.10 Scroll Bars
- •17.11 Sliders
- •17.12 Creating Multiple Windows
- •18.1 Introduction
- •18.2 Developing Applets
- •18.3 The HTML File and the <applet> Tag
- •18.4 Applet Security Restrictions
- •18.5 Enabling Applets to Run as Applications
- •18.6 Applet Life-Cycle Methods
- •18.7 Passing Strings to Applets
- •18.8 Case Study: Bouncing Ball
- •18.9 Case Study: TicTacToe
- •18.10 Locating Resources Using the URL Class
- •18.11 Playing Audio in Any Java Program
- •18.12 Case Study: Multimedia Animations
- •19.1 Introduction
- •19.2 How is I/O Handled in Java?
- •19.3 Text I/O vs. Binary I/O
- •19.4 Binary I/O Classes
- •19.5 Problem: Copying Files
- •19.6 Object I/O
- •19.7 Random-Access Files
- •20.1 Introduction
- •20.2 Problem: Computing Factorials
- •20.3 Problem: Computing Fibonacci Numbers
- •20.4 Problem Solving Using Recursion
- •20.5 Recursive Helper Methods
- •20.6 Problem: Finding the Directory Size
- •20.7 Problem: Towers of Hanoi
- •20.8 Problem: Fractals
- •20.9 Problem: Eight Queens
- •20.10 Recursion vs. Iteration
- •20.11 Tail Recursion
- •APPENDIXES
- •INDEX

25 add(jpRadioButtons, BorderLayout.WEST);
26
27// Create a radio-button group to group three buttons
28ButtonGroup group = new ButtonGroup();
29group.add(jrbRed);
30group.add(jrbGreen);
31group.add(jrbBlue);
32
33// Set keyboard mnemonics
34jrbRed.setMnemonic('E');
35jrbGreen.setMnemonic('G');
36jrbBlue.setMnemonic('U');
38// Register listeners for radio buttons
39jrbRed.addActionListener(new ActionListener() {
40public void actionPerformed(ActionEvent e) {
41messagePanel.setForeground(Color.red);
42}
43});
44jrbGreen.addActionListener(new ActionListener() {
45public void actionPerformed(ActionEvent e) {
46messagePanel.setForeground(Color.green);
47}
48});
49jrbBlue.addActionListener(new ActionListener() {
50public void actionPerformed(ActionEvent e) {
51messagePanel.setForeground(Color.blue);
52}
53});
54
55// Set initial message color to blue
56jrbBlue.setSelected(true);
57messagePanel.setForeground(Color.blue);
58}
59}
RadioButtonDemo extends CheckBoxDemo and adds three radio buttons to specify the message color. When a radio button is clicked, its action event listener sets the corresponding foreground color in messagePanel.
The keyboard mnemonics 'R' and 'B' are already set for the Right button and Bold check box. To avoid conflict, the keyboard mnemonics 'E', 'G', and 'U' are set on the radio buttons Red, Green, and Blue, respectively (lines 34–36).
The program creates a ButtonGroup and puts three JRadioButton instances (jrbRed, jrbGreen, and jrbBlue) in the group (lines 28–31).
A radio button fires an ActionEvent and an ItemEvent when it is selected or deselected. You could process either the ActionEvent or the ItemEvent to choose a color. The example processes the ActionEvent. Please rewrite the code using the ItemEvent as an exercise.
17.5 Labels
A label is a display area for a short text, an image, or both. It is often used to label other components (usually text fields). Figure 17.15 lists the constructors and methods in JLabel.
JLabel inherits all the properties from JComponent and has many properties similar to the ones in JButton, such as text, icon, horizontalAlignment, verticalAlignment,
17.5 Labels 583
group buttons
register listener
register listener
register listener

584 Chapter 17 Creating Graphical User Interfaces
horizontalTextPosition, verticalTextPosition, and iconTextGap. For example, the following code displays a label with text and an icon:
//Create an image icon from an image file ImageIcon icon = new ImageIcon("image/grapes.gif");
//Create a label with a text, an icon,
//with centered horizontal alignment
JLabel jlbl = new JLabel("Grapes", icon, SwingConstants.CENTER);
//Set label's text alignment and gap between text and icon jlbl.setHorizontalTextPosition(SwingConstants.CENTER); jlbl.setVerticalTextPosition(SwingConstants.BOTTOM); jlbl.setIconTextGap(5);
javax.swing.JComponent |
|
|
|
|
|
The get and set methods for these data fields are provided |
|
|
|
||
|
|
in the class, but omitted in the UML diagram for brevity. |
|
javax.swing.JLabel |
|||
|
|
||
|
|
|
|
-text: String |
|
The label’s text. |
|
-icon: javax.swing.Icon |
|
The label’s image icon. |
|
-horizontalAlignment: int |
|
The horizontal alignment of the text and icon on the label. |
|
-horizontalTextPosition: int |
|
The horizontal text position relative to the icon on the label. |
|
-verticalAlignment: int |
|
The vertical alignment of the text and icon on the label. |
|
-verticalTextPosition: int |
|
The vertical text position relative to the icon on the label. |
|
-iconTextGap: int |
|
The gap between the text and the icon on the label. |
|
+JLabel() |
|
Creates a default label with no text and icon. |
|
+JLabel(icon: javax.swing.Icon) |
|
Creates a label with an icon. |
|
+JLabel(icon: Icon, hAlignment: int) |
|
Creates a label with an icon and the specified horizontal alignment. |
|
+JLabel(text: String) |
|
Creates a label with text. |
|
+JLabel(text: String, icon: Icon, |
|
Creates a label with text, an icon, and the specified horizontal alignment. |
|
hAlignment: int) |
|
|
|
+JLabel(text: String, hAlignment: int) |
|
Creates a label with text and the specified horizontal alignment. |
|
|
|
|
FIGURE 17.15 JLabel displays text or an icon, or both.
Video Note
Use labels and text fields
17.6 Text Fields
A text field can be used to enter or display a string. JTextField is a subclass of JTextComponent. Figure 17.16 lists the constructors and methods in JTextField.
JTextField inherits JTextComponent, which inherits JComponent. Here is an example of creating a text field with red foreground color and right horizontal alignment:
JTextField jtfMessage = new JTextField("T-Strom"); jtfMessage.setForeground(Color.RED); jtfMessage.setHorizontalAlignment(SwingConstants.RIGHT);
When you move the cursor in the text field and press the Enter key, it fires an ActionEvent. Listing 17.5 gives a program that adds a text field to the preceding example to let the user
set a new message, as shown in Figure 17.17.

javax.swing.text.JTextComponent
-text: String -editable: boolean
javax.swing.JTextField
-columns: int -horizontalAlignment: int
+JTextField() +JTextField(column: int) +JTextField(text: String)
+JTextField(text: String, columns: int)
+addActionEvent(listener: ActionListener): void
17.6 Text Fields 585
The get and set methods for these data fields are provided in the class, but omitted in the UML diagram for brevity.
The text contained in this text component.
Indicates whether this text component is editable (default: true).
The number of columns in this text field.
The horizontal alignment of this text field (default: LEFT). Creates a default empty text field with number of columns set to 0. Creates an empty text field with a specified number of columns. Creates a text field initialized with the specified text.
Creates a text field initialized with the specified text and columns. Adds an ActionListener for this object.
FIGURE 17.16 JTextField enables you to enter or display a string.
JPanel with BorderLayout for a label and
a text field
FIGURE 17.17 A label and a text field are added to set a new message.
LISTING 17.5 TextFieldDemo.java
1 import java.awt.*;
2 import java.awt.event.*;
3 import javax.swing.*;
4
5 public class TextFieldDemo extends RadioButtonDemo {
6 private JTextField jtfMessage = new JTextField(10);
7
8/** Main method */
9public static void main(String[] args) {
10TextFieldDemo frame = new TextFieldDemo();
11frame.pack();
12frame.setTitle("TextFieldDemo");
13frame.setLocationRelativeTo(null); // Center the frame
14frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
15frame.setVisible(true);
16}
17
18public TextFieldDemo() {
19// Create a new panel to hold label and text field
20JPanel jpTextField = new JPanel();
21jpTextField.setLayout(new BorderLayout(5, 0));
ButtonDemo
CheckBoxDemo
RadioButtonDemo
TextFieldDemo
create frame pack frame
create UI
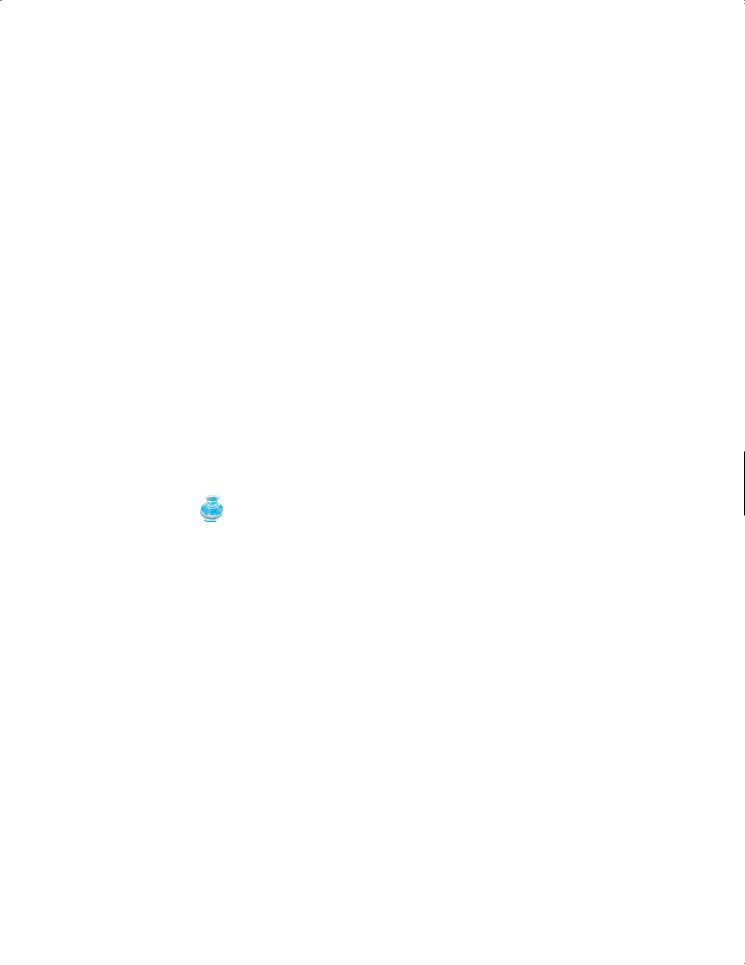
586 Chapter 17 |
Creating Graphical User Interfaces |
||||||
|
22 |
|
jpTextField.add( |
||||
|
23 |
|
new JLabel("Enter a new message"), BorderLayout.WEST); |
||||
|
24 |
|
jpTextField.add(jtfMessage, BorderLayout.CENTER); |
||||
|
25 |
|
add(jpTextField, BorderLayout.NORTH); |
||||
|
26 |
|
|
|
|
|
|
|
27 |
|
jtfMessage.setHorizontalAlignment(JTextField.RIGHT); |
||||
|
28 |
|
|
|
|
|
|
|
29 |
|
// Register listener |
||||
listener |
30 |
|
jtfMessage.addActionListener(new ActionListener() { |
|
|||
|
31 |
|
/** Handle ActionEvent */ |
||||
|
32 |
|
public void actionPerformed(ActionEvent e) { |
||||
|
33 |
|
|
messagePanel.setMessage(jtfMessage.getText()); |
|
|
|
|
34 |
|
|
jtfMessage.requestFocusInWindow(); |
|
|
|
|
35 |
} |
|
|
|
|
|
|
36 |
}); |
|
|
|
|
|
|
37 |
} |
|
|
|
|
|
|
38 |
} |
|
|
|
|
|
|
TextFieldDemo extends RadioButtonDemo and adds a label and a text field to let the user |
||||||
|
enter a new message. After you set a new message in the text field and press the Enter key, a |
||||||
|
new message is displayed. Pressing the Enter key on the text field triggers an action event. |
||||||
|
The listener sets a new message in messagePanel (line 33). |
||||||
pack() |
|
The pack() method (line 11) automatically sizes the frame according to the size of the |
|||||
|
components placed in it. |
||||||
requestFocusInWindow() |
|
The requestFocusInWindow() method (line 34) defined in the Component class requests |
|||||
|
the component to receive input focus. Thus, jtfMessage.requestFocusInWindow() |
||||||
|
requests the input focus on jtfMessage. You will see the cursor on jtfMessage after the |
||||||
|
actionPerformed method is invoked. |
||||||
|
|
Note |
|||||
JPasswordField |
|
If a text field is used for entering a password, use JPasswordField to replace JTextField. |
|||||
|
|
JPasswordField extends JTextField and hides the input text with echo characters (e.g., |
|||||
|
|
******). By default, the echo character is *. You can specify a new echo character using the |
|||||
|
|
setEchoChar(char) method. |
|||||
|
17.7 Text Areas |
||||||
|
If you want to let the user enter multiple lines of text, you have to create several instances of |
||||||
|
JTextField. A better alternative is to use JTextArea, which enables the user to enter mul- |
||||||
|
tiple lines of text. Figure 17.18 lists the constructors and methods in JTextArea. |
||||||
|
|
Like JTextField, JTextArea inherits JTextComponent, which contains the methods |
|||||
|
getText, setText, isEditable, and setEditable. Here is an example of creating a text |
||||||
|
area with 5 rows and 20 columns, line-wrapped on words, red foreground color, and |
||||||
|
Courier font, bold, 20 pixels. |
||||||
|
|
JTextArea jtaNote = new JTextArea("This is a text area", 5, 20); |
|||||
wrap line |
|
jtaNote.setLineWrap(true); |
|||||
wrap word |
|
jtaNote.setWrapStyleWord(true); |
|||||
|
|
jtaNote.setForeground(Color.red); |
|||||
|
|
jtaNote.setFont(new Font("Courier", Font.BOLD, 20)); |
JTextArea does not handle scrolling, but you can create a JScrollPane object to hold an instance of JTextArea and let JScrollPane handle scrolling for JTextArea, as follows:
// Create a scroll pane to hold text area
JScrollPane scrollPane = new JScrollPane(jta = new JTextArea()); add(scrollPane, BorderLayout.CENTER);

17.7 Text Areas 587
Listing 17.7 gives a program that displays an image and a text in a label, and a text in a text area, as shown in Figure 17.19.
javax.swing.text.JTextComponent |
|
|
|
|
|
The get and set methods for these data fields are provided |
|
|
|||
|
|
in the class, but omitted in the UML diagram for brevity. |
|
javax.swing.JTextArea |
|||
|
|
||
|
|
|
|
-columns: int |
|
The number of columns in this text area. |
|
-rows: int |
|
The number of rows in this text area. |
|
-tabSize: int |
|
The number of characters used to expand tabs (default: 8). |
|
-lineWrap: boolean |
|
Indicates whether the line in the text area is automatically |
|
|
|
wrapped (default: false). |
|
-wrapStyleWord: boolean |
|
Indicates whether the line is wrapped on words or characters (default: false). |
|
+JTextArea() |
|
Creates a default empty text area. |
|
+JTextArea(rows: int, columns: int) |
|
Creates an empty text area with the specified number of rows and columns. |
|
+JTextArea(text: String) |
|
Creates a new text area with the specified text displayed. |
|
+JTextArea(text: String, rows: int, columns: int) |
|
Creates a new text area with the specified text and number of rows and columns. |
|
+append(s: String): void |
|
Appends the string to text in the text area. |
|
+insert(s: String, pos: int): void |
|
Inserts string s in the specified position in the text area. |
|
+replaceRange(s: String, start: int, end: int): |
|
Replaces partial text in the range from position start to end with string s. |
|
void |
|
Returns the actual number of lines contained in the text area. |
|
+getLineCount(): int |
|
||
|
|
|
FIGURE 17.18 JTextArea enables you to enter or display multiple lines of characters.
A label showing an image and a text
DescriptionPanel
with BorderLayout
A text area inside a scroll panel
FIGURE 17.19 The program displays an image in a label, a title in a label, and a text in the text area.
Here are the major steps in the program:
1.Create a class named DescriptionPanel that extends JPanel, as shown in Listing 17.6. This class contains a text area inside a scroll pane, and a label for displaying an image icon and a title. This class is used in the present example and will be reused in later examples.
2.Create a class named TextAreaDemo that extends JFrame, as shown in Listing 17.7. Create an instance of DescriptionPanel and add it to the center of the frame. The relationship between DescriptionPanel and TextAreaDemo is shown in Figure 17.20.
LISTING 17.6 DescriptionPanel.java
1 import javax.swing.*;
2 import java.awt.*;
3
4 public class DescriptionPanel extends JPanel {
5/** Label for displaying an image icon and a text */
6 |
private JLabel jlblImageTitle = new JLabel(); |
label |
7 |
|
|

588 Chapter 17 Creating Graphical User Interfaces
|
8 |
|
/** Text area for displaying text */ |
|
|
|
|
|
|
||||||||
text area |
9 |
|
private JTextArea jtaDescription = new JTextArea(); |
||||||||||||||
|
10 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
11 |
|
public DescriptionPanel() { |
|
|
|
|
|
|
||||||||
|
12 |
|
|
// Center the icon and text and place the text under the icon |
|||||||||||||
label properties |
13 |
|
|
jlblImageTitle.setHorizontalAlignment(JLabel.CENTER); |
|||||||||||||
|
14 |
|
|
jlblImageTitle.setHorizontalTextPosition(JLabel.CENTER); |
|||||||||||||
|
15 |
|
|
jlblImageTitle.setVerticalTextPosition(JLabel.BOTTOM); |
|||||||||||||
|
16 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
17 |
|
|
// Set the font in the label and the text field |
|||||||||||||
|
18 |
|
|
jlblImageTitle.setFont(new Font("SansSerif", Font.BOLD, 16)); |
|||||||||||||
|
19 |
|
|
jtaDescription.setFont(new Font("Serif", Font.PLAIN, 14)); |
|||||||||||||
|
20 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
21 |
|
|
// Set lineWrap and wrapStyleWord true for the text area |
|||||||||||||
wrap line |
22 |
|
|
jtaDescription.setLineWrap(true); |
|
|
|
|
|
|
|
||||||
wrap word |
23 |
|
|
jtaDescription.setWrapStyleWord(true); |
|
|
|
|
|
|
|
||||||
read only |
24 |
|
|
jtaDescription.setEditable(false); |
|
|
|
|
|
|
|
|
|||||
|
25 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
26 |
|
|
// Create a scroll pane to hold the text area |
|||||||||||||
scroll pane |
27 |
|
|
JScrollPane scrollPane = new JScrollPane(jtaDescription); |
|||||||||||||
|
28 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
29 |
|
|
// Set BorderLayout for the panel, add label and scrollpane |
|||||||||||||
|
30 |
|
|
setLayout(new BorderLayout(5, 5)); |
|
|
|
|
|
|
|||||||
|
31 |
|
|
add(scrollPane, BorderLayout.CENTER); |
|
|
|
|
|
|
|||||||
|
32 |
|
|
add(jlblImageTitle, BorderLayout.WEST); |
|
|
|
|
|
|
|||||||
|
33 |
} |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
34 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
35 |
|
/** Set the title */ |
|
|
|
|
|
|
||||||||
|
36 |
|
public void setTitle(String title) { |
|
|
|
|
|
|
||||||||
|
37 |
|
|
jlblImageTitle.setText(title); |
|
|
|
|
|
|
|||||||
|
38 |
} |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
39 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
40 |
|
/** Set the image icon */ |
|
|
|
|
|
|
||||||||
|
41 |
|
public void setImageIcon(ImageIcon icon) { |
|
|
|
|
|
|
||||||||
|
42 |
|
|
jlblImageTitle.setIcon(icon); |
|
|
|
|
|
|
|||||||
|
43 |
} |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
44 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
45 |
|
/** Set the text description */ |
|
|
|
|
|
|
||||||||
|
46 |
|
public void setDescription(String text) { |
|
|
|
|
|
|
||||||||
|
47 |
|
|
jtaDescription.setText(text); |
|
|
|
|
|
|
|||||||
|
48 |
} |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
49 |
} |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
||||
|
|
|
|
|
|
|
|
|
|
|
|
|
javax.swing.JFrame |
||||
|
|
|
|
|
javax.swing.JPanel |
|
|
|
|
|
|
|
|||||
|
|
|
|
|
|
|
1 |
|
1 |
|
|
|
|
||||
|
|
|
|
|
|
|
|
|
|
|
|
||||||
|
|
|
|
|
|
|
|
|
|
|
|
||||||
|
|
|
|
|
DescriptionPanel |
|
TextAreaDemo |
|
|||||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
||||
|
|
|
|
|
|
|
|
|
|
|
|
||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
-jlblImage: JLabel
-jtaDescription: JTextArea
+setImageIcon(icon: ImageIcon): void +setTitle(title: String): void +setDescription(text: String): void
FIGURE 17.20 TextAreaDemo uses DescriptionPanel to display an image, title, and text description of a national flag.

17.7 Text Areas 589
The text area is inside a JScrollPane (line 27), which provides scrolling functions for the text area. Scroll bars automatically appear if there is more text than the physical size of the text area, and disappear if the text is deleted and the remaining text does not exceed the text area size.
The lineWrap property is set to true (line 22) so that the line is automatically wrapped when the text cannot fit in one line. The wrapStyleWord property is set to true (line 23) so that the line is wrapped on words rather than characters. The text area is set noneditable (line 24), so you cannot edit the description in the text area.
It is not necessary to create a separate class for DescriptionPanel in this example. Nevertheless, this class was created for reuse in the next section, where you will use it to display a description panel for various images.
LISTING 17.7 TextAreaDemo.java
1 import java.awt.*;
2 import javax.swing.*;
3
4 public class TextAreaDemo extends JFrame {
5// Declare and create a description panel
6 |
private DescriptionPanel descriptionPanel = new DescriptionPanel(); |
create decriptionPanel |
7 |
|
|
8public static void main(String[] args) {
9 |
TextAreaDemo frame = new TextAreaDemo(); |
create frame |
10frame.pack();
11frame.setLocationRelativeTo(null); // Center the frame
12frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
13frame.setTitle("TextAreaDemo");
14frame.setVisible(true);
15}
16 |
|
|
17 |
public TextAreaDemo() { |
|
18 |
// Set title, text and image in the description panel |
create UI |
19descriptionPanel.setTitle("Canada");
20String description = "The Maple Leaf flag \n\n" +
21"The Canadian National Flag was adopted by the Canadian " +
22"Parliament on October 22, 1964 and was proclaimed into law " +
23"by Her Majesty Queen Elizabeth II (the Queen of Canada) on " +
24"February 15, 1965. The Canadian Flag (colloquially known " +
25"as The Maple Leaf Flag) is a red flag of the proportions " +
26"two by length and one by width, containing in its center a " +
27"white square, with a single red stylized eleven-point " +
28"maple leaf centered in the white square.";
29descriptionPanel.setDescription(description);
30descriptionPanel.setImageIcon(new ImageIcon("image/ca.gif"));
31
32// Add the description panel to the frame
33setLayout(new BorderLayout());
34 |
add(descriptionPanel, BorderLayout.CENTER); |
add decriptionPanel |
35}
36}
TextAreaDemo simply creates an instance of DescriptionPanel (line 6) and sets the title (line 19), image (line 30), and text in the description panel (line 29). DescriptionPanel is a subclass of JPanel. DescriptionPanel contains a label for displaying an image icon and a text title, and a text area for displaying a description of the image.