
- •CONTENTS
- •1.1 Introduction
- •1.2 What Is a Computer?
- •1.3 Programs
- •1.4 Operating Systems
- •1.5 Java, World Wide Web, and Beyond
- •1.6 The Java Language Specification, API, JDK, and IDE
- •1.7 A Simple Java Program
- •1.8 Creating, Compiling, and Executing a Java Program
- •1.9 (GUI) Displaying Text in a Message Dialog Box
- •2.1 Introduction
- •2.2 Writing Simple Programs
- •2.3 Reading Input from the Console
- •2.4 Identifiers
- •2.5 Variables
- •2.7 Named Constants
- •2.8 Numeric Data Types and Operations
- •2.9 Problem: Displaying the Current Time
- •2.10 Shorthand Operators
- •2.11 Numeric Type Conversions
- •2.12 Problem: Computing Loan Payments
- •2.13 Character Data Type and Operations
- •2.14 Problem: Counting Monetary Units
- •2.15 The String Type
- •2.16 Programming Style and Documentation
- •2.17 Programming Errors
- •2.18 (GUI) Getting Input from Input Dialogs
- •3.1 Introduction
- •3.2 boolean Data Type
- •3.3 Problem: A Simple Math Learning Tool
- •3.4 if Statements
- •3.5 Problem: Guessing Birthdays
- •3.6 Two-Way if Statements
- •3.7 Nested if Statements
- •3.8 Common Errors in Selection Statements
- •3.9 Problem: An Improved Math Learning Tool
- •3.10 Problem: Computing Body Mass Index
- •3.11 Problem: Computing Taxes
- •3.12 Logical Operators
- •3.13 Problem: Determining Leap Year
- •3.14 Problem: Lottery
- •3.15 switch Statements
- •3.16 Conditional Expressions
- •3.17 Formatting Console Output
- •3.18 Operator Precedence and Associativity
- •3.19 (GUI) Confirmation Dialogs
- •4.1 Introduction
- •4.2 The while Loop
- •4.3 The do-while Loop
- •4.4 The for Loop
- •4.5 Which Loop to Use?
- •4.6 Nested Loops
- •4.7 Minimizing Numeric Errors
- •4.8 Case Studies
- •4.9 Keywords break and continue
- •4.10 (GUI) Controlling a Loop with a Confirmation Dialog
- •5.1 Introduction
- •5.2 Defining a Method
- •5.3 Calling a Method
- •5.4 void Method Example
- •5.5 Passing Parameters by Values
- •5.6 Modularizing Code
- •5.7 Problem: Converting Decimals to Hexadecimals
- •5.8 Overloading Methods
- •5.9 The Scope of Variables
- •5.10 The Math Class
- •5.11 Case Study: Generating Random Characters
- •5.12 Method Abstraction and Stepwise Refinement
- •6.1 Introduction
- •6.2 Array Basics
- •6.3 Problem: Lotto Numbers
- •6.4 Problem: Deck of Cards
- •6.5 Copying Arrays
- •6.6 Passing Arrays to Methods
- •6.7 Returning an Array from a Method
- •6.8 Variable-Length Argument Lists
- •6.9 Searching Arrays
- •6.10 Sorting Arrays
- •6.11 The Arrays Class
- •7.1 Introduction
- •7.2 Two-Dimensional Array Basics
- •7.3 Processing Two-Dimensional Arrays
- •7.4 Passing Two-Dimensional Arrays to Methods
- •7.5 Problem: Grading a Multiple-Choice Test
- •7.6 Problem: Finding a Closest Pair
- •7.7 Problem: Sudoku
- •7.8 Multidimensional Arrays
- •8.1 Introduction
- •8.2 Defining Classes for Objects
- •8.3 Example: Defining Classes and Creating Objects
- •8.4 Constructing Objects Using Constructors
- •8.5 Accessing Objects via Reference Variables
- •8.6 Using Classes from the Java Library
- •8.7 Static Variables, Constants, and Methods
- •8.8 Visibility Modifiers
- •8.9 Data Field Encapsulation
- •8.10 Passing Objects to Methods
- •8.11 Array of Objects
- •9.1 Introduction
- •9.2 The String Class
- •9.3 The Character Class
- •9.4 The StringBuilder/StringBuffer Class
- •9.5 Command-Line Arguments
- •9.6 The File Class
- •9.7 File Input and Output
- •9.8 (GUI) File Dialogs
- •10.1 Introduction
- •10.2 Immutable Objects and Classes
- •10.3 The Scope of Variables
- •10.4 The this Reference
- •10.5 Class Abstraction and Encapsulation
- •10.6 Object-Oriented Thinking
- •10.7 Object Composition
- •10.8 Designing the Course Class
- •10.9 Designing a Class for Stacks
- •10.10 Designing the GuessDate Class
- •10.11 Class Design Guidelines
- •11.1 Introduction
- •11.2 Superclasses and Subclasses
- •11.3 Using the super Keyword
- •11.4 Overriding Methods
- •11.5 Overriding vs. Overloading
- •11.6 The Object Class and Its toString() Method
- •11.7 Polymorphism
- •11.8 Dynamic Binding
- •11.9 Casting Objects and the instanceof Operator
- •11.11 The ArrayList Class
- •11.12 A Custom Stack Class
- •11.13 The protected Data and Methods
- •11.14 Preventing Extending and Overriding
- •12.1 Introduction
- •12.2 Swing vs. AWT
- •12.3 The Java GUI API
- •12.4 Frames
- •12.5 Layout Managers
- •12.6 Using Panels as Subcontainers
- •12.7 The Color Class
- •12.8 The Font Class
- •12.9 Common Features of Swing GUI Components
- •12.10 Image Icons
- •13.1 Introduction
- •13.2 Exception-Handling Overview
- •13.3 Exception-Handling Advantages
- •13.4 Exception Types
- •13.5 More on Exception Handling
- •13.6 The finally Clause
- •13.7 When to Use Exceptions
- •13.8 Rethrowing Exceptions
- •13.9 Chained Exceptions
- •13.10 Creating Custom Exception Classes
- •14.1 Introduction
- •14.2 Abstract Classes
- •14.3 Example: Calendar and GregorianCalendar
- •14.4 Interfaces
- •14.5 Example: The Comparable Interface
- •14.6 Example: The ActionListener Interface
- •14.7 Example: The Cloneable Interface
- •14.8 Interfaces vs. Abstract Classes
- •14.9 Processing Primitive Data Type Values as Objects
- •14.10 Sorting an Array of Objects
- •14.11 Automatic Conversion between Primitive Types and Wrapper Class Types
- •14.12 The BigInteger and BigDecimal Classes
- •14.13 Case Study: The Rational Class
- •15.1 Introduction
- •15.2 Graphical Coordinate Systems
- •15.3 The Graphics Class
- •15.4 Drawing Strings, Lines, Rectangles, and Ovals
- •15.5 Case Study: The FigurePanel Class
- •15.6 Drawing Arcs
- •15.7 Drawing Polygons and Polylines
- •15.8 Centering a String Using the FontMetrics Class
- •15.9 Case Study: The MessagePanel Class
- •15.10 Case Study: The StillClock Class
- •15.11 Displaying Images
- •15.12 Case Study: The ImageViewer Class
- •16.1 Introduction
- •16.2 Event and Event Source
- •16.3 Listeners, Registrations, and Handling Events
- •16.4 Inner Classes
- •16.5 Anonymous Class Listeners
- •16.6 Alternative Ways of Defining Listener Classes
- •16.7 Problem: Loan Calculator
- •16.8 Window Events
- •16.9 Listener Interface Adapters
- •16.10 Mouse Events
- •16.11 Key Events
- •16.12 Animation Using the Timer Class
- •17.1 Introduction
- •17.2 Buttons
- •17.3 Check Boxes
- •17.4 Radio Buttons
- •17.5 Labels
- •17.6 Text Fields
- •17.7 Text Areas
- •17.8 Combo Boxes
- •17.9 Lists
- •17.10 Scroll Bars
- •17.11 Sliders
- •17.12 Creating Multiple Windows
- •18.1 Introduction
- •18.2 Developing Applets
- •18.3 The HTML File and the <applet> Tag
- •18.4 Applet Security Restrictions
- •18.5 Enabling Applets to Run as Applications
- •18.6 Applet Life-Cycle Methods
- •18.7 Passing Strings to Applets
- •18.8 Case Study: Bouncing Ball
- •18.9 Case Study: TicTacToe
- •18.10 Locating Resources Using the URL Class
- •18.11 Playing Audio in Any Java Program
- •18.12 Case Study: Multimedia Animations
- •19.1 Introduction
- •19.2 How is I/O Handled in Java?
- •19.3 Text I/O vs. Binary I/O
- •19.4 Binary I/O Classes
- •19.5 Problem: Copying Files
- •19.6 Object I/O
- •19.7 Random-Access Files
- •20.1 Introduction
- •20.2 Problem: Computing Factorials
- •20.3 Problem: Computing Fibonacci Numbers
- •20.4 Problem Solving Using Recursion
- •20.5 Recursive Helper Methods
- •20.6 Problem: Finding the Directory Size
- •20.7 Problem: Towers of Hanoi
- •20.8 Problem: Fractals
- •20.9 Problem: Eight Queens
- •20.10 Recursion vs. Iteration
- •20.11 Tail Recursion
- •APPENDIXES
- •INDEX
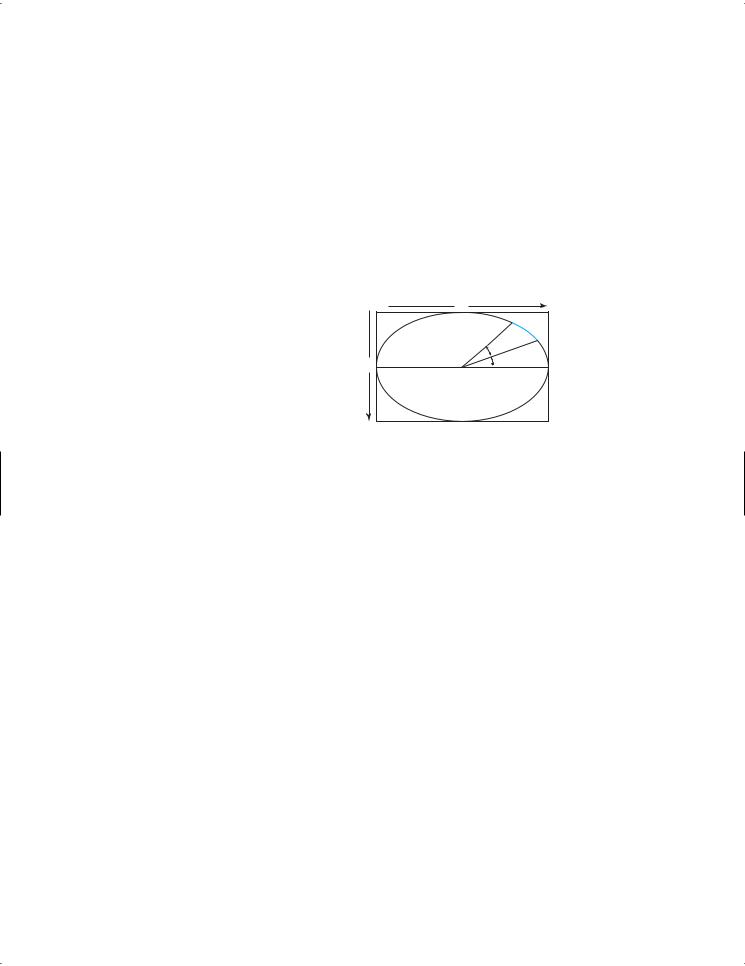
506 Chapter 15 Graphics
15.6 Drawing Arcs
An arc is conceived as part of an oval bounded by a rectangle. The methods to draw or fill an arc are as follows:
drawArc(int x, int y, int w, int h, int startAngle, int arcAngle); fillArc(int x, int y, int w, int h, int startAngle, int arcAngle);
Parameters x, y, w, and h are the same as in the drawOval method; parameter startAngle is the starting angle; arcAngle is the spanning angle (i.e., the angle covered by the arc). Angles are measured in degrees and follow the usual mathematical conventions (i.e., 0 degrees is in the easterly direction, and positive angles indicate counterclockwise rotation from the easterly direction); see Figure 15.11.
Listing 15.4 is an example of how to draw arcs; the output is shown in Figure 15.12.
(x, y) |
w |
|
arcAngle |
h |
startAngle |
|
FIGURE 15.11 The drawArc method draws an arc based on an oval with specified angles.
LISTING 15.4 DrawArcs.java
|
1 |
import javax.swing.JFrame; |
||||
|
2 |
import javax.swing.JPanel; |
||||
|
3 |
import java.awt.Graphics; |
||||
|
4 |
|
|
|
|
|
|
5 |
public class DrawArcs extends JFrame { |
||||
|
6 |
|
public DrawArcs() { |
|||
|
7 |
|
|
setTitle("DrawArcs"); |
||
add a panel |
8 |
|
|
add(new ArcsPanel()); |
||
|
9 |
} |
|
|
|
|
|
10 |
|
|
|
|
|
|
11 |
|
/** Main method */ |
|||
|
12 |
|
public static void main(String[] args) { |
|||
|
13 |
|
|
DrawArcs frame = new DrawArcs(); |
||
|
14 |
|
frame.setSize(250, 300); |
|||
|
15 |
|
frame.setLocationRelativeTo(null); // Center the frame |
|||
|
16 |
|
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); |
|||
|
17 |
|
frame.setVisible(true); |
|||
|
18 |
} |
|
|
|
|
|
19 |
} |
|
|
|
|
|
20 |
|
|
|
|
|
|
21 |
// The class for drawing arcs on a panel |
||||
|
22 |
class ArcsPanel extends JPanel { |
||||
|
23 |
// Draw four blades of a fan |
||||
override paintComponent |
24 |
|
protected void paintComponent(Graphics g) |
{ |
||
|
25 |
|
|
super.paintComponent(g); |
|
|
|
26 |
|
|
|
|
|
|
27 |
|
int xCenter = getWidth() / 2; |
|||
|
28 |
|
int yCenter = getHeight() / 2; |
|||
|
29 |
|
int radius = (int)(Math.min(getWidth(), getHeight()) * 0.4); |
|||
|
30 |
|
|
|
|
|
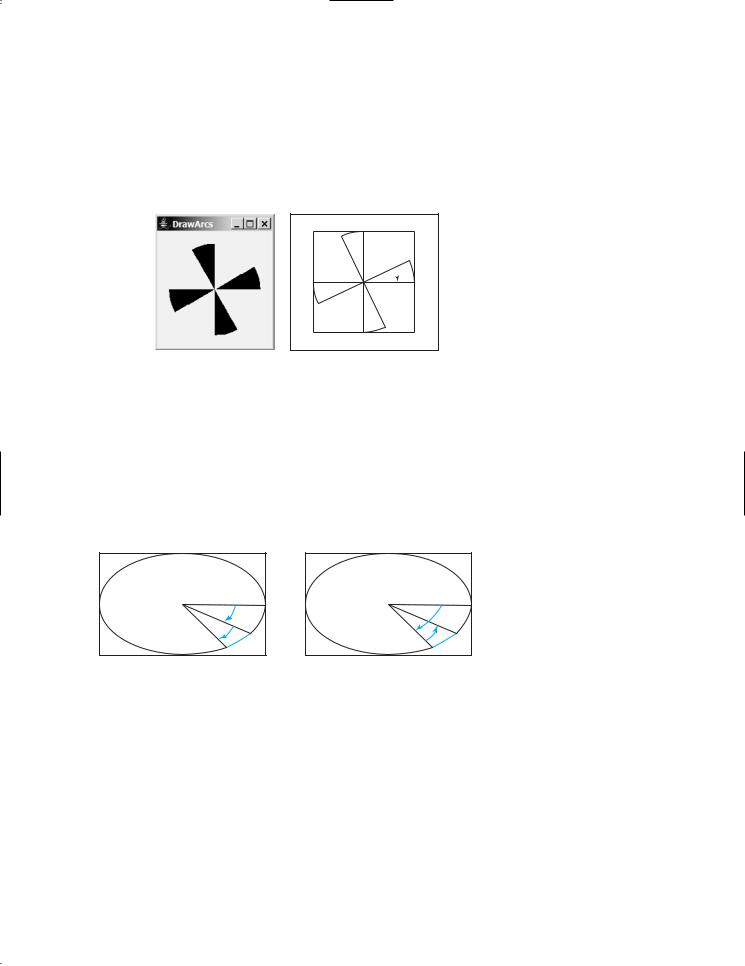
15.7 Drawing Polygons and Polylines 507
31int x = xCenter - radius;
32int y = yCenter - radius;
34 |
g.fillArc(x, y, 2 |
* radius, 2 |
* radius, 0, 30); |
|
30° arc from 0° |
|||||||
35 |
g.fillArc(x, y, 2 |
* radius, 2 |
* radius, 90, 30); |
|
30° arc from 90° |
|||||||
36 |
g.fillArc(x, |
y, |
2 |
* radius, |
2 |
* |
radius, |
180, |
30); |
30° arc from 180° |
||
37 |
g.fillArc(x, |
y, |
2 |
* radius, |
2 |
* |
radius, |
270, |
30); |
30° arc from 270° |
38}
39}
(x, y)
30
FIGURE 15.12 The program draws four filled arcs.
Angles may be negative. A negative starting angle sweeps clockwise from the easterly direc- negative degrees tion, as shown in Figure 15.13. A negative spanning angle sweeps clockwise from the starting
angle. The following two statements draw the same arc:
g.fillArc(x, y, 2 * radius, 2 * radius, -30, -20); g.fillArc(x, y, 2 * radius, 2 * radius, -50, 20);
The first statement uses negative starting angle -30 and negative spanning angle -20, as shown in Figure 15.13(a). The second statement uses negative starting angle -50 and positive spanning angle 20, as shown in Figure 15.13(b).
–30 |
–50 |
–20 |
20 |
(a)Negative starting angle –30 and negative spanning angle –20
(b)Negative starting angle –50 and positive spanning angle 20
FIGURE 15.13 Angles may be negative.
15.7 Drawing Polygons and Polylines
To draw a polygon, first create a Polygon object using the Polygon class, as shown in Figure 15.14.
A polygon is a closed two-dimensional region. This region is bounded by an arbitrary number of line segments, each being one side (or edge) of the polygon. A polygon comprises a list of (x, y)-coordinate pairs in which each pair defines a vertex of the polygon, and two successive pairs are the endpoints of a line that is a side of the polygon. The first and final points are joined by a line segment that closes the polygon.
Here is an example of creating a polygon and adding points into it:
Polygon polygon = new Polygon(); polygon.addPoint(40, 20);
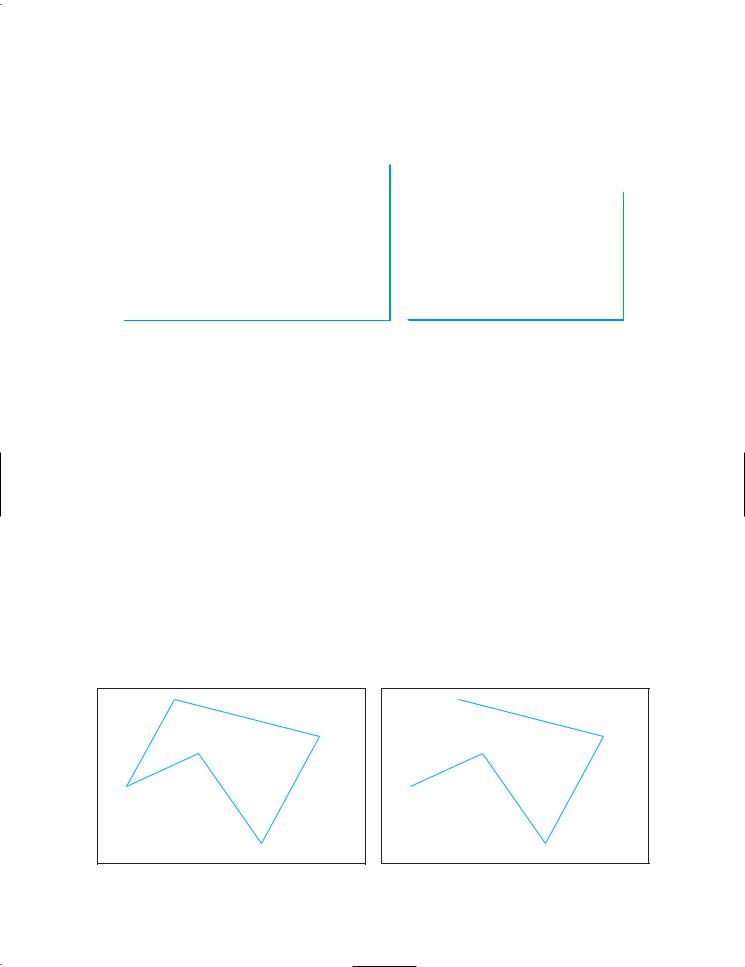
508 Chapter 15 Graphics
polygon.addPoint(70, 40); polygon.addPoint(60, 80); polygon.addPoint(45, 45); polygon.addPoint(20, 60);
java.awt.Polygon |
|
|
|
|
|
+xpoints: int[] |
|
x-coordinates of all points in the polygon. |
+ypoints: int[] |
|
y-coordinates of all points in the polygon. |
+npoints: int |
|
The number of points in the polygon. |
|
|
Creates an empty polygon. |
+Polygon() |
|
|
+Polygon(xpoints: int[], ypoints: int[], |
|
Creates a polygon with the specified points. |
npoints: int) |
|
|
+addPoint(x: int, y: int) |
|
Appends a point to the polygon. |
|
|
|
FIGURE 15.14 The Polygon class models a polygon.
After these points are added, xpoints is {40, 70, 60, 45, 20}, ypoints is {20, 40, 80, 45, 60}, and npoints is 5. xpoints, ypoints, and npoints are public data fields in Polygon, which is a bad design. In principle, all data fields should be kept private.
To draw or fill a polygon, use one of the following methods in the Graphics class:
drawPolygon(Polygon polygon);
fillPolygon(Polygon polygon);
drawPolygon(int[] xpoints, int[] ypoints, int npoints);
fillPolygon(int[] xpoints, int[] ypoints, int npoints);
For example:
int x[] = {40, 70, 60, 45, 20}; int y[] = {20, 40, 80, 45, 60}; g.drawPolygon(x, y, x.length);
The drawing method opens the polygon by drawing lines between point (x[i], y[i]) and point (x[i+1], y[i+1]) for i = 0, ... , x.length-1; it closes the polygon by drawing a line between the first and last points (see Figure 15.15(a)).
(x[0], y[0]) |
(x[0], y[0]) |
|
|
(x[1], y[1]) |
(x[1], y[1]) |
(x[3], y[3]) |
(x[3], y[3]) |
(x[4], y[4]) |
(x[4], y[4]) |
(x[2], y[2]) |
(x[2], y[2]) |
(a) Polygon |
(b) Polyline |
FIGURE 15.15 The drawPolygon method draws a polygon, and the polyLine method draws a polyline.
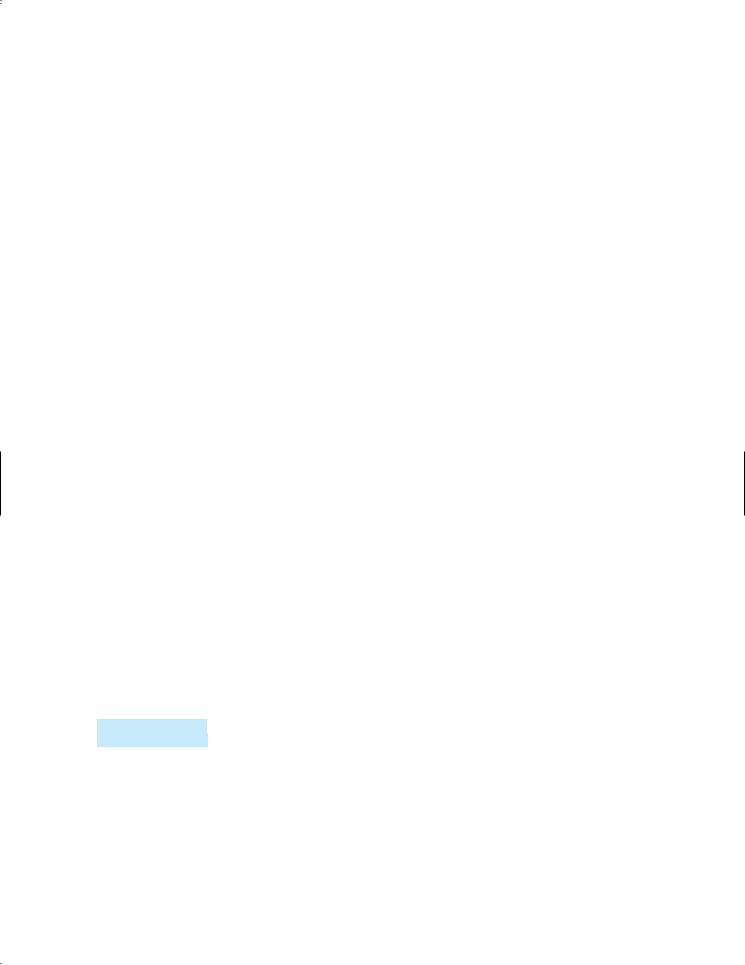
15.7 Drawing Polygons and Polylines 509
To draw a polyline, use the drawPolyline(int[] x, int[] y, int nPoints) method, which draws a sequence of connected lines defined by arrays of x- and y-coordinates. For example, the following code draws the polyline, as shown in Figure 15.15(b).
int x[] = {40, 70, 60, 45, 20}; int y[] = {20, 40, 80, 45, 60}; g.drawPolyline(x, y, x.length);
Listing 15.5 is an example of how to draw a hexagon, with the output shown in Figure 15.16.
LISTING 15.5 DrawPolygon.java
1 import javax.swing.JFrame;
2 import javax.swing.JPanel;
3 import java.awt.Graphics;
4 import java.awt.Polygon;
5
6 public class DrawPolygon extends JFrame {
7public DrawPolygon() {
8setTitle("DrawPolygon");
9 |
add(new PolygonsPanel()); |
add a panel |
10 |
} |
|
11 |
|
|
12/** Main method */
13public static void main(String[] args) {
14DrawPolygon frame = new DrawPolygon();
15frame.setSize(200, 250);
16frame.setLocationRelativeTo(null); // Center the frame
17frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
18frame.setVisible(true);
19}
20}
21 |
|
|
|
|
|
|
22 |
// Draw a polygon in the panel |
|
||||
23 |
class PolygonsPanel extends JPanel { |
|
||||
24 |
|
protected void paintComponent(Graphics g) |
{ |
paintComponent |
||
25 |
|
|
super.paintComponent(g); |
|
|
|
26 |
|
|
|
|
|
|
27int xCenter = getWidth() / 2;
28int yCenter = getHeight() / 2;
29int radius = (int)(Math.min(getWidth(), getHeight()) * 0.4);
31// Create a Polygon object
32Polygon polygon = new Polygon();
34// Add points to the polygon in this order
35 |
polygon.addPoint(xCenter + radius, yCenter); |
add a point |
36polygon.addPoint((int)(xCenter + radius *
37Math.cos(2 * Math.PI / 6)), (int)(yCenter – radius *
38Math.sin(2 * Math.PI / 6)));
39polygon.addPoint((int)(xCenter + radius *
40Math.cos(2 * 2 * Math.PI / 6)), (int)(yCenter – radius *
41Math.sin(2 * 2 * Math.PI / 6)));
42polygon.addPoint((int)(xCenter + radius *
43Math.cos(3 * 2 * Math.PI / 6)), (int)(yCenter – radius *
44Math.sin(3 * 2 * Math.PI / 6)));
45polygon.addPoint((int)(xCenter + radius *
46Math.cos(4 * 2 * Math.PI / 6)), (int)(yCenter – radius *
47Math.sin(4 * 2 * Math.PI / 6)));
48polygon.addPoint((int)(xCenter + radius *