
- •brief contents
- •contents
- •foreword
- •preface
- •acknowledgments
- •about this book
- •Roadmap
- •Code conventions and downloads
- •Author Online
- •About the author
- •about the cover illustration
- •1 Why add Groovy to Java?
- •1.1 Issues with Java
- •1.1.1 Is static typing a bug or a feature?
- •1.1.2 Methods must be in a class, even if you don’t need or want one
- •1.1.3 Java is overly verbose
- •1.1.4 Groovy makes testing Java much easier
- •1.1.5 Groovy tools simplify your build
- •1.2 Groovy features that help Java
- •1.3 Java use cases and how Groovy helps
- •1.3.1 Spring framework support for Groovy
- •1.3.2 Simplified database access
- •1.3.3 Building and accessing web services
- •1.3.4 Web application enhancements
- •1.4 Summary
- •2 Groovy by example
- •2.1 Hello, Groovy
- •2.2 Accessing Google Chart Tools
- •2.2.1 Assembling the URL with query string
- •2.2.2 Transmitting the URL
- •2.2.3 Creating a UI with SwingBuilder
- •2.3 Groovy Baseball
- •2.3.1 Database data and Plain Old Groovy Objects
- •2.3.2 Parsing XML
- •2.3.3 HTML builders and groovlets
- •2.4 Summary
- •3 Code-level integration
- •3.1 Integrating Java with other languages
- •3.2 Executing Groovy scripts from Java
- •3.2.1 Using JSR223 scripting for the Java Platform API
- •3.2.2 Working with the Groovy Eval class
- •3.2.3 Working with the GroovyShell class
- •3.2.4 Calling Groovy from Java the easy way
- •3.2.5 Calling Java from Groovy
- •3.3 Summary
- •4 Using Groovy features in Java
- •4.1 Treating POJOs like POGOs
- •4.2 Implementing operator overloading in Java
- •4.3 Making Java library classes better: the Groovy JDK
- •4.4 Cool AST transformations
- •4.4.1 Delegating to contained objects
- •4.4.2 Creating immutable objects
- •4.4.3 Creating singletons
- •4.5 Working with XML
- •4.6 Working with JSON data
- •4.7 Summary
- •5 Build processes
- •5.1 The build challenge
- •5.2 The Java approach, part 1: Ant
- •5.3 Making Ant Groovy
- •5.3.1 The <groovy> Ant task
- •5.3.2 The <groovyc> Ant task
- •5.3.3 Writing your build in Groovy with AntBuilder
- •5.3.4 Custom build scripts with Gant
- •5.3.5 Ant summary
- •5.4 The Java approach, part 2: Maven
- •5.4.2 The GMaven project
- •5.4.3 Maven summary
- •5.5 Grapes and @Grab
- •5.6 The Gradle build system
- •5.6.1 Basic Gradle builds
- •5.6.2 Interesting configurations
- •5.7 Summary
- •6 Testing Groovy and Java projects
- •6.1 Working with JUnit
- •6.1.1 A Java test for the Groovy implementation
- •6.1.2 A Groovy test for the Java implementation
- •6.1.3 A GroovyTestCase test for a Java implementation
- •6.2 Testing scripts written in Groovy
- •6.2.1 Useful subclasses of GroovyTestCase: GroovyShellTestCase
- •6.2.2 Useful subclasses of GroovyTestCase: GroovyLogTestCase
- •6.3 Testing classes in isolation
- •6.3.1 Coerced closures
- •6.3.2 The Expando class
- •6.3.3 StubFor and MockFor
- •6.4 The future of testing: Spock
- •6.4.1 The Search for Spock
- •6.4.2 Test well, and prosper
- •6.4.4 The trouble with tribbles
- •6.4.5 Other Spock capabilities
- •6.5 Summary
- •7 The Spring framework
- •7.1 A Spring application
- •7.2 Refreshable beans
- •7.3 Spring AOP with Groovy beans
- •7.4 Inline scripted beans
- •7.5 Groovy with JavaConfig
- •7.6 Building beans with the Grails BeanBuilder
- •7.7 Summary
- •8 Database access
- •8.1 The Java approach, part 1: JDBC
- •8.2 The Groovy approach, part 1: groovy.sql.Sql
- •8.3 The Java approach, part 2: Hibernate and JPA
- •8.4 The Groovy approach, part 2: Groovy and GORM
- •8.4.1 Groovy simplifications
- •8.5 Groovy and NoSQL databases
- •8.5.1 Populating Groovy vampires
- •8.5.2 Querying and mapping MongoDB data
- •8.6 Summary
- •9 RESTful web services
- •9.1 The REST architecture
- •9.3 Implementing JAX-RS with Groovy
- •9.4 RESTful Clients
- •9.5 Hypermedia
- •9.5.1 A simple example: Rotten Tomatoes
- •9.5.2 Adding transitional links
- •9.5.3 Adding structural links
- •9.5.4 Using a JsonBuilder to control the output
- •9.6 Other Groovy approaches
- •9.6.1 Groovlets
- •9.6.2 Ratpack
- •9.6.3 Grails and REST
- •9.7 Summary
- •10 Building and testing web applications
- •10.1 Groovy servlets and ServletCategory
- •10.2 Easy server-side development with groovlets
- •10.2.1 A “Hello, World!” groovlet
- •10.2.2 Implicit variables in groovlets
- •10.3.2 Integration testing with Gradle
- •10.3.3 Automating Jetty in the Gradle build
- •10.4 Grails: the Groovy “killer app”
- •10.4.1 The quest for the holy Grails
- •10.5 Summary
- •A.1 Installing a JDK
- •A.2 Installing Groovy
- •A.3 Testing your installation
- •A.4 IDE support
- •A.5 Installing other projects in the Groovy ecosystem
- •B.1 Scripts and the traditional example
- •B.2 Variables, numbers, and strings
- •B.2.1 Numbers
- •B.2.2 Strings and Groovy strings
- •B.3 Plain Old Groovy Objects
- •B.4 Collections
- •B.4.1 Ranges
- •B.4.2 Lists
- •B.4.3 Maps
- •B.5 Closures
- •B.6 Loops and conditionals
- •B.6.1 Loops
- •B.6.2 Conditionals
- •B.6.3 Elvis
- •B.6.4 Safe de-reference
- •B.7 File I/O
- •B.8.1 Parsing and slurping XML
- •B.8.2 Generating XML
- •B.8.3 Validation
- •B.9 JSON support
- •B.9.1 Slurping JSON
- •B.9.2 Building JSON
- •index
- •Symbols

Working with JSON data |
89 |
4.6Working with JSON data
Groovy processes JSON data as easily as it processes XML. To conclude this chapter, let me present a trivial example of JSON response data from a web service.
The service is known as ICNDB: the Internet Chuck Norris Database. It is located at http://icndb.com and has a RESTful API for retrieving the associated jokes. If you send an HTTP GET request to http://api.icndb.com/jokes/random?limitTo=[nerdy] you get back a string in JSON form.
Groovy makes it easy to send a GET request. In the Groovy JDK the String class has a toURL method, which converts it to an instance of java.net.URL. Then the Groovy JDK adds a method to the URL class called getText. Accessing the web service is therefore as simple as
String url = 'http://api.icndb.com/jokes/random?limitTo=[nerdy]' String jsonTxt = url.toURL().text
println jsonTxt
Executing this returns a JSON object of the form
{ "type": "success", "value": { "id": 563, "joke": "Chuck Norris causes the Windows Blue Screen of Death.", "categories": ["nerdy"] } }
In all the Google geocoder demonstrations I’ve used so far in this book I introduced the XmlSlurper class, whose parse method takes the URL in string form and automatically converts the result to a DOM tree. Since version 1.8, Groovy also includes a JsonSlurper, but it doesn’t have as many overloads of the parse method as the XmlSlurper does. It does, however, contain a parseText method, which can process the jsonTxt returned from the previous code.
If I add that to the earlier lines, the complete ICNDB script is shown in the next listing.
Listing 4.11 chuck_norris.groovy, which processes data from ICNDB
import groovy.json.JsonSlurper
String url = 'http://api.icndb.com/jokes/random?limitTo=[nerdy]' String jsonTxt = url.toURL().text
def json = new JsonSlurper().parseText(jsonTxt) def joke = json?.value?.joke
println joke
The parseText method on JsonSlurper converts the JSON data into Groovy maps and lists. I then access the value property of the json object, which is a contained JSON object. It has a joke property, which contains the string I’m looking for.
The result of executing this script is something like this:
Chuck Norris can make a method abstract and final
Just as generating XML is done by scripting the output through a MarkupBuilder, generating JSON data uses the groovy.json.JsonBuilder class. See the GroovyDocs for JsonBuilder for a complete example.
www.it-ebooks.info
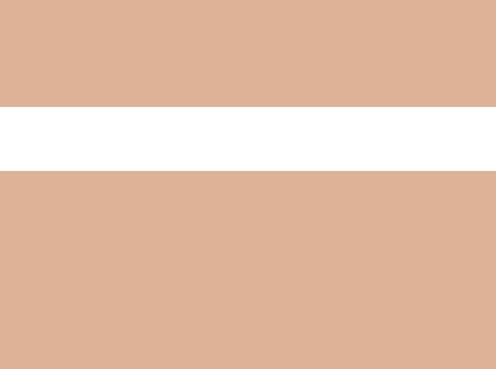
90 |
CHAPTER 4 Using Groovy features in Java |
Lessons learned (JSON)
1The JsonSlurper class has a parseText method for working with JSON formatted strings.
2The JsonBuilder class generates JSON strings using the same mechanism as the XmlSlurper.
This completes the tour of Groovy features that can be added to Java applications regardless of use case.
Lessons learned (Groovy features used in Java)
1When Groovy access a POJO it can use the map-based constructor as though it were a POGO.
2Every operator in Groovy delegates to a method, and if that method is implemented in a Java class the operator in Groovy will still use it. This means you can do operator overloading even in a Java class.
3The Groovy JDK documents all the methods that Groovy adds to the Java standard API through metaprogramming.
4Groovy AST transformations can only be applied to Groovy classes, but the classes can be mixed with Java in interesting ways. This chapter includes examples of @Delegate, @Immutable, and @Singleton.
4.7Summary
This chapter reviewed many ways that Groovy can help Java at the basic level, from POJO enhancements to AST transformations to building XML and more. I’ll use these techniques in future chapters wherever they can help. I’ll also review other helpful techniques along the way, though these are most of the major ones.
The next couple of chapters, however, change the focus. Although mixing Java and Groovy is easy and is a major theme of this book, some companies are reluctant to add Groovy to production code until their developers have a certain minimum comfort level with the language. As it happens, there are two major areas where Groovy can strongly impact and simplify Java projects without being integrated directly. The first of those is one of the major pain points in enterprise development: the build process. The other is testing, which is valued more highly the better the developer.
By covering these two techniques early in the book I can then use, for example, Gradle builds and Spock tests when I attack the use cases Java developers typically encounter, like web services, database manipulation, or working with the Spring framework.
www.it-ebooks.info

Part 2
Groovy tools
Welcome to part 2: “Groovy tools.” In these two chapters, I address two of the major ways Groovy is often introduced into an organization: namely, build processes and testing.
Chapter 5 on build processes reviews the dominant build tools in the Java world, Ant and Maven, and shows how to add Groovy dependencies to each. It also covers the Ant tasks that work with Groovy and the major Groovy plugins for Maven. Finally, it provides an introduction to Gradle, one of the most important projects in the Groovy world, and includes examples covering several interesting build tasks.
Chapter 6 on testing starts with JUnit tests in both Java and Groovy and then looks at the JUnit subclass GroovyTestCase and its descendants and what additional capabilities they bring. Then it covers the MockFor and StubFor classes in the Groovy library, which are great ways to build mock objects and also provide some insight into Groovy metaprogramming. Finally, the chapter ends with a good overview of the Spock testing framework, which includes mocking capabilities of its own.
www.it-ebooks.info
www.it-ebooks.info