
6_Location
.pdfCore Location
lecturer: Davidov Dmitriy
What is the Core Location Framework?
The Core Location framework lets you determine the current location or heading associated with a device. The framework uses the available hardware to determine the user’s position and heading. You use the classes and protocols in this framework to configure and schedule the delivery of location and heading events. You can also use it to define geographic regions and monitor when the user crosses the boundaries of those regions. In iOS, you can also define a region around a Bluetooth beacon.
Getting the User’s Location
Apps use location data for many purposes, ranging from social networking to turn- by-turn navigation services. They get location data through the classes of the Core Location framework. This framework provides several services that you can use to get and monitor the device’s current location:
-The standard location service offers a highly configurable way to get the current location and track changes.
-Region monitoring lets you monitor boundary crossings for defined geographical regions and Bluetooth low-energy beacon regions. (Beacon region monitoring is available in iOS only.)
-The significant-change location service provides a way to get the current location and be notified when significant changes occur, but it’s critical to use it correctly to avoid using too much power.
Require location service
If your iOS app requires location services to function properly, include the
UIRequiredDeviceCapabilities key in the app’s Info.plist file. The App Store uses the information in this key to prevent users from downloading apps to devices that don’t contain the listed features.
The value for the UIRequiredDeviceCapabilities is an array of strings indicating the features that your app requires. Two strings are relevant to location services:
-Include the location-services string if you require location services in general.
-Include the gps string if your app requires the accuracy offered only by GPS hardware.
Requesting Authorization for Location Services
iOS 8 fixes that by breaking up location services permissions into two different types of authorization.
-“When In Use” authorization only gives the app permission to receive your location when — you guessed it — the app is in use.
-(void)requestWhenInUseAuthorization;
-“Always” authorization, gives the app traditional background permissions, just as has always existed in prior iOS versions.
-(void)requestAlwaysAuthorization;
- (void)locationManager:(CLLocationManager *)manager didChangeAuthorizationStatus:(CLAuthorizationStatus)status;
Correct method to start observing location.
Determining Whether Location Services Status
+ (CLAuthorizationStatus)authorizationStatus;
typedef enum { kCLAuthorizationStatusNotDetermined = 0,
//The user has not yet made a choice regarding whether this app can use location services.
kCLAuthorizationStatusRestricted,
//This app is not authorized to use location services. The user cannot change this app’s status, possibly due to active restrictions such as parental controls being in place.
kCLAuthorizationStatusDenied,
//The user explicitly denied the use of location services for this app or location services are currently disabled in Settings.
kCLAuthorizationStatusAuthorized,
kCLAuthorizationStatusAuthorizedAlways = kCLAuthorizationStatusAuthorized, kCLAuthorizationStatusAuthorizedWhenInUse
} CLAuthorizationStatus;
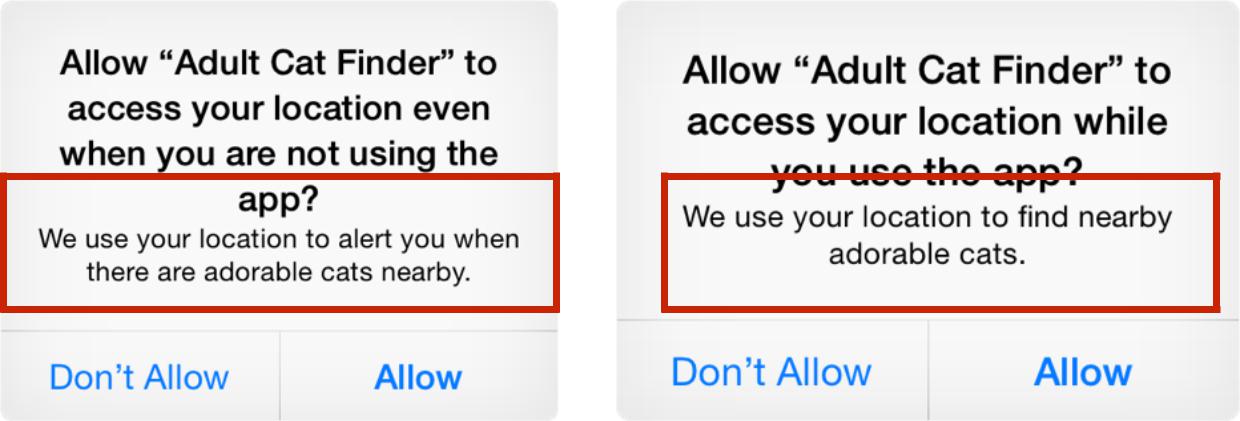
User Message for Authorization request
Info.plist
NSLocationWhenInUseUsageDescription
NSLocationAlwaysUsageDescription

User Message for Authorization request
[[UIApplication sharedApplication] openURL:[NSURL URLWithString:UIApplicationOpenSettingsURLString]];
Determining Whether Location Services Are Available
There are situations where location services may not be available. For example:
-The user disables location services in the Settings app or System Preferences.
-The user denies location services for a specific app.
-The device is in Airplane mode and unable to power up the necessary hardware.
CLLocationManager
+(BOOL)locationServicesEnabled;
Authorization Workflow
1 Call the authorizationStatus class method to get the current authorization status for your app.
If the authorization status is kCLAuthorizationStatusRestricted or kCLAuthorizationStatusDenied, your app is not permitted to use location services and you should abort your attempt to use them.
2 Create your CLLocationManager object and assign a delegate to it. Store a strong reference to your location manager somewhere in your app.
3 In iOS, if the authorization status was kCLAuthorizationStatusNotDetermined, call the requestWhenInUseAuthorization or requestAlwaysAuthorization method to request the appropriate type of authorization from the user.
4 Depending on the services you need, call one or more of the following methods:
-If you use the standard location service, call the locationServicesEnabled method.
-If you use the significant location-change service, call the significantLocationChangeMonitoringAvailable method.
-If you use heading information, call the headingAvailable method.
-If you monitor geographic or beacon regions, call the isMonitoringAvailableForClass: method.
-If you perform ranging on Bluetooth beacons, call the isRangingAvailable