
Beginning JavaScript With DOM Scripting And Ajax - From Novice To Professional (2006)
.pdf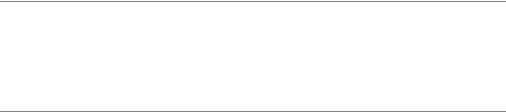
36 |
C H A P T E R 2 ■ D A T A A N D D E C I S I O N S |
This can be a handy feature if you need to add days onto a date. Usually we’d have to take into account the number of days in the different months, and whether it’s a leap year, if we wanted to add a number of days to a date, but it’s much easier to use JavaScript’s understanding of dates instead:
<html>
<body>
<script type="text/javascript">
// Ask the user to enter a date string
var originalDate = prompt( Enter a date (Day, Name of the Month, Year), "31 Dec 2003" );
//Overwrite the originalDate variable with a new Date
//object
var originalDate = new Date( originalDate );
//Ask the user to enter the number of days to be
//added, and convert to number
var addDays = Number( prompt( "Enter number of days to be added", "1" ) )
//Set a new value for originalDate of originalDate
//plus the days to be added
originalDate.setDate( originalDate.getDate( ) + addDays )
//Write out the date held by the originalDate
//object using the toString( ) method document.write( originalDate.toString( ) )
</script>
</body>
</html>
If you enter 31 Dec 2003 when prompted, and 1 for the number of days to be added, then the answer you’ll get is Thu Jan 1 00:00:00 UTC 2004.
■Note Notice that we’re using the Number() method of the Math object on the third line of the script. The program will still run if we don’t, but the result won’t be the same. If you don’t want to use the method, there is a trick to convert different data types: if you subtract 0 from a string that could be converted to a number using parseInt(), parseFloat(), or Number(), then you convert it to a number, and if you add an empty string, '', to a number, you convert it to a string, something you normally do with toString().
On the fourth line, we set the date to the current day of the month, the value returned by originalDate.getDate() plus the number of days to be added; then comes the calculation, and the final line outputs the date contained in the Date object as a string using the toString()

C H A P T E R 2 ■ D A T A A N D D E C I S I O N S |
37 |
method. If you’re using IE5.5+ or Gecko-based browsers (Mozilla, Netscape >6), toDateString() produces a nicely formatted string using the date alone. You can use the same methods for get and set if you’re working with UTC time—all you need to do is add UTC to the method name.
So getHours() becomes getUTCHours(), setMonth() becomes setUTCMonth(), and so on. You can also use the getTimezoneOffset() method to return the difference, in hours, between the computer’s local time and UTC time. (You’ll have to rely on users having set their time zones correctly, and be aware of the differences in daylight saving time between different countries.)
■Note For crucial date manipulation, JavaScript might not be the correct technology, as you cannot trust the client computer to be properly set up. You could, however, populate the initial date of your JavaScript via a server-side language and go from there.
The Math Object
The Math object provides us with lots of mathematical functionality, like finding the square of a number or producing a random number. The Math object is different from the Date and String objects in two ways:
•You can’t create a Math object explicitly, you just go ahead and use it.
•The Math object doesn’t store data, unlike the String and Date object. You call the methods of the Math object using the format:
Math.methodOfMathObject( aNumber ): alert( "The value of pi is " + Math.PI );
We’ll look at a few of the commonly used methods next (you can find a complete reference by running a search at http://www.mozilla.org/docs/web-developer/). We’ll look at the methods for rounding numbers and generating random numbers here.
Rounding Numbers
You saw earlier that the parseInt() function will make a fractional number whole by removing everything after the decimal point (so 24.999 becomes 24). Pretty often you’ll want more mathematically accurate calculations, if you’re working with financial calculations, for example, and for these you can use one of the Math object’s three rounding functions: round(), ceil(), and floor(). This is how they work:
•round(): Rounds a number up when the decimal is . 5 or greater
•ceil() (as in ceiling): Always rounds up, so 23.75 becomes 24, as does 23.25
•floor(): Always rounds down, so 23.75 becomes 23, as does 23.25
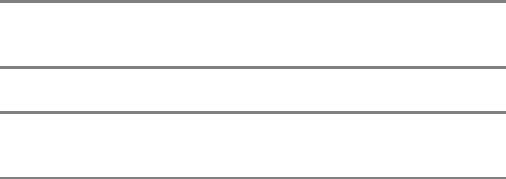
38 |
C H A P T E R 2 ■ D A T A A N D D E C I S I O N S |
Here they are at work in a simple example:
<html>
<body>
<script type="text/javascript">
var numberToRound = prompt( "Please enter a number", "" ) document.write( "round( ) = " + Math.round( numberToRound ) ); document.write( "<br>" );
document.write( "floor( ) = " + Math.floor( numberToRound ) ); document.write( "<br>" );
document.write( "ceil( ) = " + Math.ceil( numberToRound ) ); </script>
</body>
</html>
Even though we used prompt() to obtain a value from the user, which as we saw earlier returns a string, the number returned is still treated as a number. This is because the rounding methods do the conversion for us just so long as the string contains something that can be converted to a number.
If we enter 23.75, we get the following result:
round() = 24 floor() = 23 ceil() = 24
If we enter -23.75, we get
round() = -24 floor() = -24 ceil() = -23
Generating a Random Number
You can generate a fractional random number that is 0 or greater but smaller than 1 using the Math object’s random() method. Usually you’ll need to multiply the number, and then use one of the rounding methods in order to make it useful.
For example, in order to mimic a die throw, we’d need to generate a random number between 1 and 6. We could create this by multiplying the random fraction by 5, to give a fractional number between 0 and 5, and then round the number up or down to a whole number using the round() method. (We couldn’t just multiply by 6 and then round up every time using ceil(), because that would give us the occasional 0.) Then we’d have a whole number between

C H A P T E R 2 ■ D A T A A N D D E C I S I O N S |
39 |
0 and 5, so by adding 1, we can get a number between 1 and 6. This approach won’t give you a perfectly balanced die, but it’s good enough for most purposes. Here’s the code:
<html>
<body>
<script type="text/javascript">
var diceThrow = Math.round( Math.random( ) * 5 ) + 1; document.write( "You threw a " + diceThrow );
</script>
</body>
</html>
Arrays
JavaScript allows us to store and access related data using an array. An array is a bit like a row of boxes (elements), each box containing a single item of data. An array can work with any of the data types that JavaScript supports. So, for example, you could use an array to work with a list of items that the users will select from, or for a set of graph coordinates, or to reference a group of images.
Array objects, like String and Date objects, are created using the new keyword together with the constructor. We can initialize an Array object when we create it:
var preInitArray = new Array( "First item", "Second item", "Third Item" );
Or set it to hold a certain number of items:
var preDeterminedSizeArray = new Array( 3 );
Or just create an empty array:
var anArray = new Array();
You can add new items to an array by assigning values to the elements:
anArray[0] = "anItem" anArray[1] = "anotherItem" anArray[2] = "andAnother"
■Tip You do not have to use the array() constructor; instead it is perfectly valid to use a shortcut notation:
var myArray = [1, 2, 3];
var yourArray = ["red", "blue", "green"]
40 |
C H A P T E R 2 ■ D A T A A N D D E C I S I O N S |
Once we’ve populated an array, we can access its elements through their indexes or positions (which, once again, are zero-based) using square brackets:
<html>
<body>
<script type="text/javascript">
var preInitArray = new Array( "First Item", "Second Item", "Third Item" );
document.write( preInitArray[0] + "<br>" ); document.write( preInitArray[1] + "<br>" ); document.write( preInitArray[2] + "<br>" );
</script>
</body>
</html>
Using index numbers to store items is useful if you want to loop through the array—we’ll be looking at loops next.
You can use keywords to access the array elements instead of a numerical index, like this:
<html>
<body>
<script type="text/javascript">
//Creating an array object and setting index
//position 0 to equal the string Fruit
var anArray = new Array( ); anArray[0] = "Fruit";
//Setting the index using the keyword
//'CostOfApple' as the index. anArray["CostOfApple"] = 0.75; document.write( anArray[0] + "<br>" ); document.write( anArray["CostOfApple"] );
</script>
</body>
</html>
Keywords are good for situations where you can give the data useful labels, or if you’re storing entries that are only meaningful in context, like a list of graph coordinates. You can’t, however, access entries using an index number if they have been set using keywords (as you can in some other languages, like PHP). We can also use variables for the index. We can rewrite the previous example using variables (one holding a string and the other a number), instead of literal values:
<html>
<body>
<script type="text/javascript"> var anArray = new Array( ); var itemIndex = 0;
var itemKeyword = "CostOfApple";
C H A P T E R 2 ■ D A T A A N D D E C I S I O N S |
41 |
anArray[itemIndex] = "Fruit"; anArray[itemKeyword] = 0.75;
document.write( anArray[itemIndex] + "<br>" ); document.write( anArray[itemKeyword] );
</script>
</body>
</html>
Let’s put what we’ve discussed about arrays and the Math object into an example. We’ll write a script that randomly selects a banner to display at the top of the page.
We’ll use an Array object to hold some image source names, like this:
var bannerImages = new Array(); bannerImages[0] = "Banner1.jpg"; bannerImages[1] = "Banner2.jpg"; bannerImages[2] = "Banner3.jpg"; bannerImages[3] = "Banner4.jpg"; bannerImages[4] = "Banner5.jpg"; bannerImages[5] = "Banner6.jpg"; bannerImages[6] = "Banner7.jpg";
Then we need six images with corresponding names to sit in the same folder as the HTML page. You can use your own or download mine from http://www.beginningjavascript.com.
Next we’ll initialize a new variable, randomImageIndex, and use it to generate a random number. We’ll use the same method that we used to generate a random die throw earlier, but without adding 1 to the result because we need a random number from 0 to 6:
var randomImageIndex = Math.round( Math.random( ) * 6 );
Then we’ll use document.write() to write the randomly selected image into the page. Here’s the complete script:
<html>
<body>
<script type="text/javascript"> var bannerImages = new Array( ); bannerImages[0] = "Banner1.jpg"; bannerImages[1] = "Banner2.jpg"; bannerImages[2] = "Banner3.jpg"; bannerImages[3] = "Banner4.jpg"; bannerImages[4] = "Banner5.jpg"; bannerImages[5] = "Banner6.jpg"; bannerImages[6] = "Banner7.jpg";
var randomImageIndex = Math.round( Math.random( ) * 6 ); document.write( "<img alt=\"\" src=\"" +
bannerImages[randomImageIndex] + "\">" ); </script>
</body>
</html>

42 |
C H A P T E R 2 ■ D A T A A N D D E C I S I O N S |
And that’s all there is to it. Having the banner change will make it more noticeable to visitors than if you displayed the same banner every time they came to the page—and, of course, it gives the impression that the site is being updated frequently.
The Array Object’s Methods and Properties
One of the most commonly used properties of the Array object is the length property, which returns the index one count higher than the index of the last array item in the array. If, for example, you’re working with an array with elements with indexes of 0, 1, 2, 3, the length will be 4—which is useful to know if you want to add another element.
The Array object provides a number of methods for manipulating arrays, including methods for cutting a number of items from an array, or joining two arrays together. We’ll look at the methods for concatenating, slicing, and sorting next.
Cutting a Slice of an Array
The slice() method is to an Array object what the substring() method is to a String object. You simply tell the method which elements you want to be sliced. This would be useful, for example, if you wanted to slice information being passed using a URL.
The slice() method takes two parameters: the index of the first element of the slice, which will be included in the slice, and the index of the final element, which won’t be. To access the second, third, and fourth values from an array holding five values in all, we use the indexes 1 and 4:
<html>
<body>
<script type="text/javascript">
// Create and initialize the array
var fullArray = new Array( "One", "Two", "Three", "Four", "Five" );
//Slice from element 1 to element 4 and store
//in new variable sliceOfArray
var sliceOfArray = fullArray.slice( 1, 4 );
// Write out new ( zero-based ) array of 3 elements document.write( sliceOfArray[0] + "<br>" ); document.write( sliceOfArray[1] + "<br>" ); document.write( sliceOfArray[2] + "<br>" );
</script>
</body>
</html>
The new array stores the numbers in a new zero-based array, so slicing indexes 0, 1, and 2 gives us the following:
Two
Three
Four
C H A P T E R 2 ■ D A T A A N D D E C I S I O N S |
43 |
The original array is unaffected, but you could overwrite the Array object in the variable by setting it to the result of the slice() method if you needed to:
fullArray = fullArray.slice( 1, 4 );
Joining Two Arrays
The Array object’s concat() method allows us to concatenate arrays. We can add two or more arrays using this method, each new array starting where the previous one ends. Here we’re joining three arrays: arrayOne, arrayTwo, and arrayThree:
<html>
<body>
<script type="text/javascript">
var arrayOne = new Array( "One", "Two", "Three", "Four", "Five" );
var arrayTwo = new Array( "ABC", "DEF", "GHI" );
var arrayThree = new Array( "John", "Paul", "George", "Ringo" );
var joinedArray = arrayOne.concat( arrayTwo, arrayThree ); document.write( "joinedArray has " + joinedArray.length +
" elements<br>" );
document.write( joinedArray[0] + "<br>" ) document.write( joinedArray[11] + "<br>" )
</script>
</body>
</html>
The new array, joinedArray, has 12 items. The items in this array are the same as they were in each of the previous arrays; they’ve simply been concatenated together. The original arrays remain untouched.
Converting an Array to a String and Back
Having data in an array is dead handy when you want to loop through it or select certain elements. However, when you need to send the data somewhere else, it might be a good idea to convert that data to a string. You could do that by looping through the array and adding each element value to a string. However, there is no need for that, as the Array object has a method called join() that does that for you. The method takes a string as a parameter. This string will be added in between each element.
<script type="text/javascript">
var arrayThree = new Array( "John", "Paul", "George", "Ringo" );
var lineUp=arrayThree.join( ', ' ); alert( lineUp );
</script>

44 |
C H A P T E R 2 ■ D A T A A N D D E C I S I O N S |
The resulting string, lineUp, has the value "John, Paul, George, Ringo". The opposite of join() is split(), which is a method that converts a string to an array.
<script type="text/javascript">
var |
lineUp="John, Paul, |
George, Ringo"; |
var |
members=lineUp.split( |
', ' ); |
alert( members.length ); |
|
</script>
Sorting an Array
The sort() method allows us to sort the items in an array into alphabetical or numerical order:
<html>
<body>
<script type="text/javascript">
var arrayToSort = new Array( "Cabbage", "Lemon", "Apple", "Pear", "Banana" );
var sortedArray = arrayToSort.sort( ); document.write( sortedArray[0] + "<br>" ); document.write( sortedArray[1] + "<br>" ); document.write( sortedArray[2] + "<br>" ); document.write( sortedArray[3] + "<br>" ); document.write( sortedArray[4] + "<br>" );
</script>
</body>
</html>
The items are arranged like this:
Apple
Banana
Cabbage
Lemon
Pear
If, however, you lower the case of one of the letters—the A of Apple, for example—then you’ll end up with a very different result. The sorting is strictly mathematical—by the number of the character in the ASCII set, not like a human being would sort the words.

C H A P T E R 2 ■ D A T A A N D D E C I S I O N S |
45 |
If you wanted to change the order the sorted elements are displayed, you can use the reverse() method to display the last in the alphabet as the first element:
<script type="text/javascript">
var arrayToSort = new Array( "Cabbage", "Lemon", "Apple", "Pear", "Banana" );
var sortedArray = arrayToSort.sort( );
var reverseArray = sortedArray.reverse( ); document.write( reverseArray[0] + "<br />" ); document.write( reverseArray[1] + "<br />" ); document.write( reverseArray[2] + "<br />" ); document.write( reverseArray[3] + "<br />" ); document.write( reverseArray[4] + "<br />" );
</script>
The resulting list is now in reverse order:
Pear
Lemon
Cabbage
Banana
Apple
Making Decisions in JavaScript
Decision making is what gives programs their apparent intelligence. You can’t write a good program without it, whether you’re creating a game, checking a password, giving the user a set of choices based on previous decisions they have made, or something else.
Decisions are based on conditional statements, which are simply statements that evaluate to true or false. This is where the primitive Boolean data type comes in useful. Loops are the other essential tool of decision making, enabling you to loop through user input or an array, for example, and make decisions accordingly.
The Logical and Comparison Operators
There are two main groups of operators we’ll look at:
•Data comparison operators: Compare operands and return Boolean values.
•Logical operators: Test for more than one condition.
We’ll start with the comparison operators.